How to build a simple website using HTML and CSS?
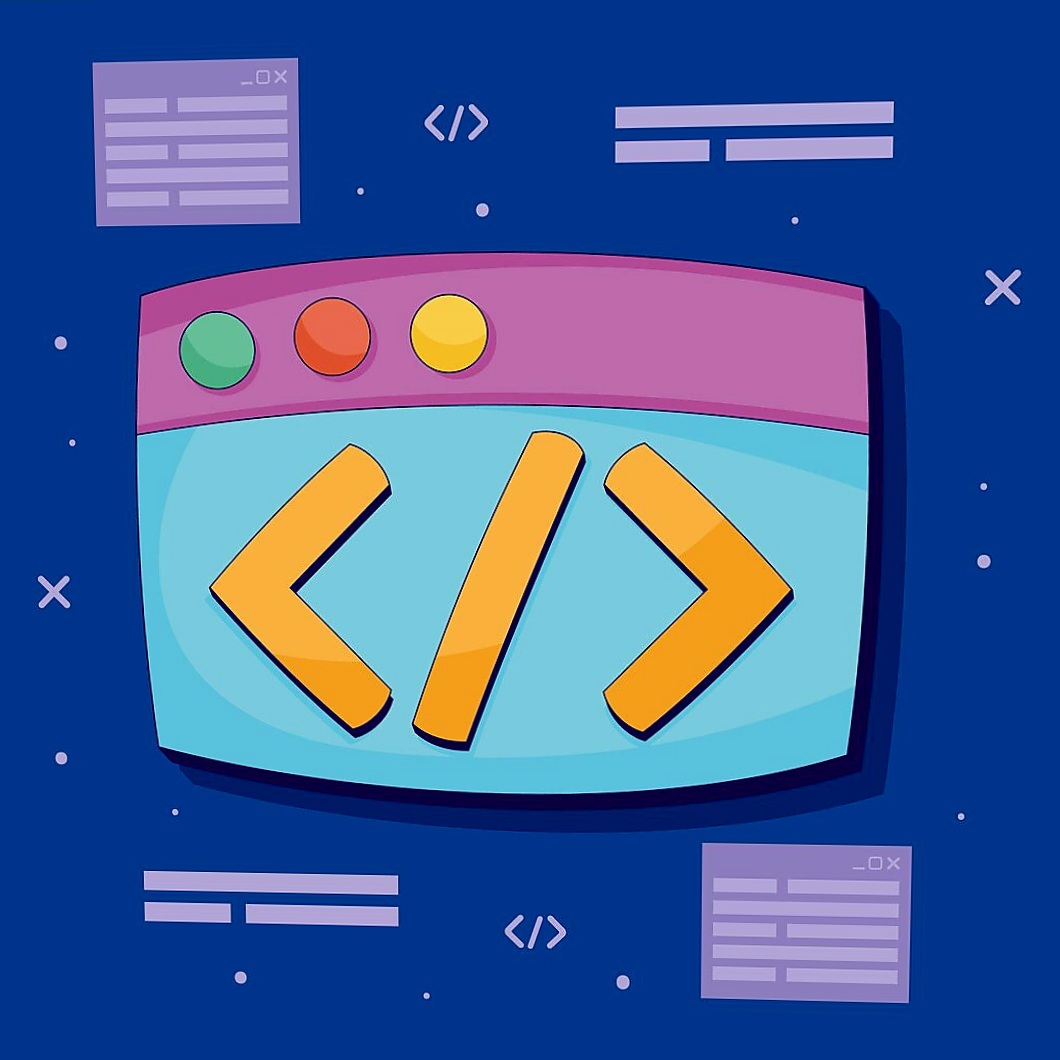
How to Build a Simple Website Using HTML and CSS: A Step-by-Step Guide for Beginners
Creating your first website is an empowering experience that opens doors to endless possibilities in the digital world. Whether you’re looking to showcase your portfolio, start a blog, or establish an online presence for your business, building a simple website using HTML and CSS is an excellent starting point. This comprehensive guide will walk you through each step, ensuring that even beginners can successfully create a functional and visually appealing website. Optimized for SEO, this article covers all the essential information you need to embark on your web development journey.
Table of Contents
- Introduction
- Understanding HTML and CSS
- What is HTML?
- What is CSS?
- Planning Your Website
- Define Your Purpose
- Sketch the Layout
- Setting Up Your Development Environment
- Choose a Code Editor
- Create Project Folders
- Building the Structure with HTML
- Basic HTML Structure
- Adding Content
- Styling with CSS
- Linking CSS to HTML
- Basic CSS Syntax
- Applying Styles
- Enhancing Your Website
- Adding Images
- Creating Navigation Menus
- Responsive Design Basics
- Testing Your Website
- Browser Compatibility
- Validating HTML and CSS
- Deploying Your Website
- Choosing a Hosting Service
- Uploading Your Files
- SEO Best Practices for Your Simple Website
- Use Semantic HTML
- Optimize Meta Tags
- Improve Page Load Speed
- Ensure Mobile-Friendliness
- Conclusion
- Additional SEO Tips for Your Web Development Guide
Introduction
Building a website from scratch might seem daunting at first, but with the right guidance and tools, it’s entirely achievable. HTML (HyperText Markup Language) and CSS (Cascading Style Sheets) are the foundational technologies for creating web pages. HTML provides the structure, while CSS handles the presentation and layout. This guide is designed to help you understand these technologies and apply them to create a simple, yet effective website.
Understanding HTML and CSS
What is HTML?
HTML is the standard markup language used to create web pages. It structures the content on the web, allowing you to define elements like headings, paragraphs, links, images, and more. HTML uses a series of tags to denote different parts of the content.
What is CSS?
CSS is a stylesheet language that describes the presentation of an HTML document. It controls the layout, colors, fonts, and overall visual appearance of the website. By separating content (HTML) from style (CSS), you can manage and maintain your website more efficiently.
Planning Your Website
Define Your Purpose
Before you start coding, it’s essential to define the purpose of your website. Ask yourself:
- What is the main goal of the website? (e.g., portfolio, blog, business site)
- Who is your target audience?
- What key features do you need? (e.g., contact form, gallery, navigation menu)
Sketch the Layout
Creating a rough sketch or wireframe of your website helps visualize the structure and layout. Decide on the placement of:
- Header: Typically contains the logo and navigation menu.
- Main Content Area: Where your primary content will reside.
- Sidebar: Optional area for additional information or links.
- Footer: Usually includes contact information, social media links, and copyright notices.
Tools like Figma, Sketch, or even pen and paper can be useful for sketching your layout.
Setting Up Your Development Environment
Choose a Code Editor
A good code editor enhances productivity and makes coding easier. Popular choices include:
- Visual Studio Code (VS Code): Highly customizable with a vast extension marketplace.
- Sublime Text: Lightweight and fast with powerful features.
- Atom: Open-source with a user-friendly interface.
Create Project Folders
Organize your project files by creating a dedicated folder structure:
my-website/
├── index.html
├── styles.css
├── images/
│ └── logo.png
└── scripts/
└── main.js (optional)
This structure keeps your files organized and manageable.
Building the Structure with HTML
Basic HTML Structure
Start by creating an index.html
file with the basic HTML boilerplate:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My Simple Website</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<header>
<h1>Welcome to My Website</h1>
<nav>
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
</header>
<main>
<section id="home">
<h2>Home</h2>
<p>This is the home section of your website.</p>
</section>
<section id="about">
<h2>About</h2>
<p>Learn more about me and what I do.</p>
</section>
<section id="contact">
<h2>Contact</h2>
<p>Get in touch through the following methods.</p>
</section>
</main>
<footer>
<p>© 2024 My Simple Website</p>
</footer>
</body>
</html>
Adding Content
Populate your HTML with meaningful content. Use appropriate tags to structure your information:
- Headings:
<h1>
to<h6>
for hierarchical titles. - Paragraphs:
<p>
for text content. - Links:
<a href="#">
for navigation. - Images:
<img src="images/logo.png" alt="Logo">
to include visuals.
Styling with CSS
Linking CSS to HTML
Ensure your CSS file is linked correctly in the <head>
section of your HTML:
<link rel="stylesheet" href="styles.css">
Basic CSS Syntax
CSS rules consist of selectors and declarations:
selector {
property: value;
}
Applying Styles
Create a styles.css
file and add styles to enhance the appearance of your website:
/* Reset default browser styles */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
/* Body Styling */
body {
font-family: Arial, sans-serif;
line-height: 1.6;
background-color: #f4f4f4;
color: #333;
padding: 20px;
}
/* Header Styling */
header {
background-color: #35424a;
color: #ffffff;
padding: 20px 0;
text-align: center;
}
header h1 {
margin-bottom: 10px;
}
nav ul {
list-style: none;
display: flex;
justify-content: center;
}
nav ul li {
margin: 0 15px;
}
nav ul li a {
color: #ffffff;
text-decoration: none;
font-weight: bold;
}
nav ul li a:hover {
text-decoration: underline;
}
/* Main Content Styling */
main {
margin-top: 20px;
}
section {
background-color: #ffffff;
padding: 20px;
margin-bottom: 20px;
border-radius: 5px;
}
/* Footer Styling */
footer {
text-align: center;
padding: 10px 0;
background-color: #35424a;
color: #ffffff;
position: fixed;
width: 100%;
bottom: 0;
}
This CSS provides a clean and modern look, enhancing the user experience.
Enhancing Your Website
Adding Images
Include images to make your website more visually appealing. Ensure you use the correct path and provide alternative text for accessibility:
<img src="images/logo.png" alt="My Website Logo">
Creating Navigation Menus
A well-structured navigation menu improves user experience. The example in the basic HTML structure includes a simple navigation bar:
<nav>
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
Responsive Design Basics
Ensure your website looks good on all devices by making it responsive. Add the following media query to your styles.css
to adjust the layout for smaller screens:
@media (max-width: 600px) {
nav ul {
flex-direction: column;
}
nav ul li {
margin: 10px 0;
}
}
This media query changes the navigation menu to a vertical layout on screens narrower than 600px.
Testing Your Website
Browser Compatibility
Test your website on different browsers (Chrome, Firefox, Safari, Edge) to ensure consistent appearance and functionality.
Validating HTML and CSS
Use validation tools to check for errors and ensure your code adheres to web standards:
- HTML Validator: W3C Markup Validation Service
- CSS Validator: W3C CSS Validation Service
Deploying Your Website
Choosing a Hosting Service
Select a hosting provider to make your website accessible online. Popular options include:
- GitHub Pages: Free hosting for static websites.
- Netlify: Free tier with continuous deployment features.
- Bluehost: Paid hosting with domain registration services.
- Hostinger: Affordable hosting plans with good performance.
Uploading Your Files
Once you’ve chosen a hosting service, follow their instructions to upload your website files. For example, with GitHub Pages:
- Create a Repository: Name it
username.github.io
. - Push Your Files: Commit and push your
index.html
,styles.css
, and other assets. - Access Your Website: Navigate to
https://username.github.io
to view your site.
SEO Best Practices for Your Simple Website
Implementing basic SEO strategies ensures your website is discoverable by search engines and reaches your target audience effectively.
Use Semantic HTML
Semantic tags like <header>
, <nav>
, <main>
, <section>
, and <footer>
provide meaningful structure, helping search engines understand your content better.
Optimize Meta Tags
Include descriptive meta tags in your HTML to improve search engine visibility:
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My Simple Website</title>
<meta name="description" content="A simple website built using HTML and CSS. Learn how to create your own website with this step-by-step guide.">
<link rel="stylesheet" href="styles.css">
</head>
Improve Page Load Speed
Fast-loading websites rank better and provide a better user experience. Optimize images, minimize CSS and JavaScript files, and leverage browser caching.
Ensure Mobile-Friendliness
With the majority of users accessing websites via mobile devices, ensure your site is responsive and mobile-friendly. Use responsive design techniques and test your website on various devices.
Conclusion
Building a simple website using HTML and CSS is a foundational skill for anyone interested in web development. By following this step-by-step guide, you can create a functional and visually appealing website tailored to your needs. Remember to plan your site, write clean code, style effectively, test thoroughly, and implement basic SEO practices to ensure your website not only looks great but also reaches your intended audience.
Embark on your web development journey with confidence, and continue exploring more advanced topics and technologies to enhance your skills further. Happy coding!
Additional SEO Tips for Your Web Development Guide
To ensure this guide ranks well on Google and attracts your target audience, consider implementing the following SEO strategies:
1. Keyword Optimization
Integrate relevant keywords naturally throughout the content to improve search engine ranking. Primary keywords include:
- “build a simple website”
- “HTML and CSS”
- “how to create a website”
- “HTML CSS tutorial for beginners”
- “step-by-step website guide”
2. Meta Tags
Ensure each page has a unique and descriptive meta title and meta description incorporating primary keywords.
Example:
<title>How to Build a Simple Website Using HTML and CSS: Step-by-Step Guide</title>
<meta name="description" content="Learn how to build a simple website using HTML and CSS with our comprehensive step-by-step guide. Perfect for beginners in web development.">
3. Header Tags
Use a clear hierarchy with header tags (H1, H2, H3) to structure the content. This improves readability and SEO.
4. Internal and External Linking
- Internal Links: Link to other relevant articles or guides on your website, such as “Introduction to Web Development” or “Advanced CSS Techniques.”
- External Links: Reference authoritative sources like the W3C HTML Validator or MDN Web Docs to add credibility.
5. Mobile Optimization
Ensure the website is fully responsive and provides a seamless experience across all devices. Use responsive design principles and test on various screen sizes.
6. Page Speed
Optimize your website’s loading speed by:
- Compressing images using tools like TinyPNG.
- Minifying CSS and JavaScript files.
- Leveraging browser caching and using Content Delivery Networks (CDNs).
7. Readable URLs
Use clear and descriptive URLs that include relevant keywords. For example:
https://yourwebsite.com/build-simple-website-html-css
8. Engaging Content
Enhance user engagement by incorporating:
- Visuals: Use images, diagrams, and infographics to complement the text.
- Code Snippets: Provide clear and formatted code examples.
- Interactive Elements: Consider embedding live code editors like CodePen for hands-on practice.
9. Schema Markup
Implement structured data (Schema.org) to help search engines understand your content better, potentially enhancing search visibility.
Example:
{
"@context": "https://schema.org",
"@type": "Article",
"headline": "How to Build a Simple Website Using HTML and CSS: A Step-by-Step Guide for Beginners",
"description": "A comprehensive guide on building a simple website using HTML and CSS. Perfect for beginners looking to create their first website.",
"author": {
"@type": "Person",
"name": "Your Name"
},
"datePublished": "2024-04-27",
"publisher": {
"@type": "Organization",
"name": "Your Website Name",
"logo": {
"@type": "ImageObject",
"url": "https://yourwebsite.com/logo.png"
}
}
}
10. Regular Updates
Keep the content fresh and up-to-date by regularly reviewing and updating the guide with the latest best practices, tools, and technologies in web development.
Final Thoughts
Creating a simple website using HTML and CSS is an achievable goal with the right guidance and resources. This guide provides a structured approach to help you build a functional and attractive website from scratch. By following the steps outlined and implementing SEO best practices, you can ensure your website not only looks great but also reaches a broader audience effectively.
Continue exploring and expanding your web development skills by diving into more advanced topics, experimenting with JavaScript, and learning about frameworks and libraries that can enhance your websites further. Embrace the learning process, stay curious, and enjoy the journey of building and refining your digital presence.
Happy coding!