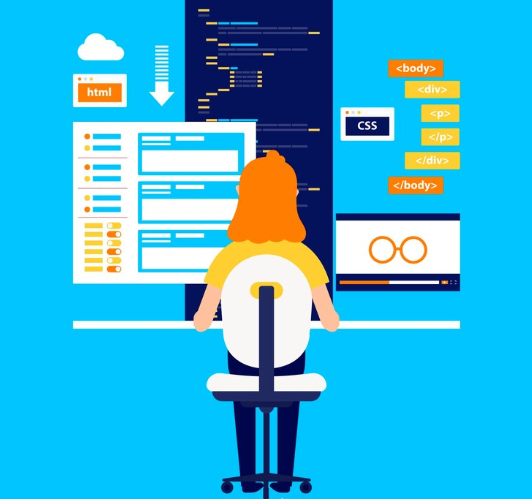
How to Install a Development Environment for Beginners: A Complete Guide
Setting up a development environment is one of the first and most crucial steps when starting to learn programming. Without a proper setup, coding can quickly become frustrating, and debugging or testing your code becomes nearly impossible. Whether you’re aiming to become a web developer, software engineer, or data scientist, this step-by-step guide will walk you through the essential process of installing a development environment tailored to your needs.
This comprehensive guide covers the basics of setting up a development environment for beginners, with detailed instructions for the most commonly used programming languages, such as Python, JavaScript (Node.js), and Java. By following this guide, you will be able to install and configure all the necessary tools and resources to begin writing, testing, and debugging code efficiently.
Table of Contents
- What is a Development Environment?
- Why Installing a Development Environment is Crucial for Beginners
- Step-by-Step Guide to Setting Up a Development Environment
- 3.1. Installing Python Development Environment
- 3.2. Installing JavaScript (Node.js) Development Environment
- 3.3. Installing Java Development Environment
- Setting Up Essential Tools: Editors and IDEs
- Testing Your Installation: Writing Your First Program
- Common Troubleshooting Tips
- Conclusion: Why a Proper Development Environment Matters
1. What is a Development Environment?
A development environment is a set of tools and software that programmers use to write, test, and debug their code. It typically includes:
- Programming language tools: Compilers, interpreters, or runtime environments.
- Text editor or Integrated Development Environment (IDE): A tool where you write and edit your code.
- Libraries and frameworks: Pre-written code that helps you build applications quickly.
- Package managers: Tools to manage external libraries and dependencies (e.g., npm for JavaScript, pip for Python).
Setting up a development environment correctly ensures that you have all the necessary components installed and configured on your computer to start coding efficiently.
2. Why Installing a Development Environment is Crucial for Beginners
Installing a proper development environment is vital for a variety of reasons:
1. Efficient Coding Process
Without the right tools, it can be difficult to compile and run your code. A development environment provides all the necessary components that make coding easier, from compiling code to running it and debugging issues.
2. Productivity Boost
An optimized environment helps you write code faster by providing features such as syntax highlighting, code completion, and error highlighting. Modern IDEs and text editors provide suggestions and catch common mistakes, helping you learn quicker and write cleaner code.
3. Cross-Platform Compatibility
Most development environments and tools are available across different operating systems like Windows, macOS, and Linux, ensuring that your code works on any platform you choose to develop on.
4. Collaboration
When working in teams, it’s essential that everyone is using a consistent development environment. Having a standardized setup ensures that your code is compatible with others’ environments, making collaboration smoother.
3. Step-by-Step Guide to Setting Up a Development Environment
In this section, we’ll break down the installation process for three popular programming languages: Python, JavaScript (Node.js), and Java. Each setup includes the necessary tools to start coding right away.
3.1. Installing Python Development Environment
Python is a great choice for beginners due to its simple syntax and versatility. Whether you’re interested in web development, data science, or automation, Python has something to offer.
Steps for Python Installation
- Install Python
- Go to the official Python website at https://www.python.org/downloads/.
- Download the latest version of Python for your operating system (Windows, macOS, or Linux).
- During the installation, ensure the option to “Add Python to PATH” is checked. This will make Python accessible from the command line.
- Install a Text Editor or IDE
- For Python development, Visual Studio Code (VSCode) is highly recommended due to its lightweight nature and broad extension support.
- Download VSCode from https://code.visualstudio.com/ and install it.
- Once installed, open VSCode and navigate to the Extensions tab to search for and install the Python extension.
- Install Python Libraries
- Python uses pip, its package manager, to install additional libraries. For instance, you can install libraries for data analysis, machine learning, and web frameworks:
pip install numpy pandas flask
- Python uses pip, its package manager, to install additional libraries. For instance, you can install libraries for data analysis, machine learning, and web frameworks:
- Verify Installation
- Open a terminal or command prompt and type:
python --version
This should display the installed version of Python.
- To test your installation, create a simple Python file (e.g.,
hello.py
):print("Hello, World!")
- Run the file using the command:
python hello.py
- Open a terminal or command prompt and type:
3.2. Installing JavaScript (Node.js) Development Environment
JavaScript is the most widely used language for web development. Node.js allows you to run JavaScript on the server side, while client-side JavaScript is executed in the browser.
Steps for Node.js Installation
- Install Node.js
- Visit the official Node.js website: https://nodejs.org/.
- Download the LTS (Long-Term Support) version for your operating system.
- Follow the installation instructions, which will automatically install npm (Node Package Manager) alongside Node.js.
- Install a Text Editor or IDE
- Visual Studio Code (VSCode) is also a great choice for JavaScript development.
- Install it from https://code.visualstudio.com/ and add the JavaScript/Node.js extensions to enhance your coding experience.
- Test Node.js Installation
- To verify that Node.js is installed correctly, open a terminal and run:
node --version
This will display the version of Node.js installed.
- You can create a simple JavaScript file (
app.js
) and run it:console.log("Hello, Node.js!");
- Run the file using the command:
node app.js
- To verify that Node.js is installed correctly, open a terminal and run:
- Install Additional Libraries
- With npm, you can easily install libraries such as Express (for web servers) or Axios (for HTTP requests):
npm install express axios
- With npm, you can easily install libraries such as Express (for web servers) or Axios (for HTTP requests):
3.3. Installing Java Development Environment
Java is a widely-used programming language, particularly in enterprise applications, Android development, and large-scale systems.
Steps for Java Installation
- Install Java Development Kit (JDK)
- Go to the official Oracle website: https://www.oracle.com/java/technologies/javase-jdk11-downloads.html.
- Download the latest version of the JDK for your operating system.
- Follow the installation prompts to install the JDK on your machine.
- Set Up Environment Variables (Windows)
- After installing the JDK, you may need to add its bin directory to your system’s PATH variable on Windows.
- To do this, go to Control Panel > System > Advanced System Settings > Environment Variables, and add the path to the
bin
folder (e.g.,C:\Program Files\Java\jdk-11\bin
).
- Install a Text Editor or IDE
- Popular IDEs for Java include Eclipse and IntelliJ IDEA. Eclipse is free and widely used for Java development.
- Download and install Eclipse from https://www.eclipse.org/downloads/.
- Verify Java Installation
- Open the terminal or command prompt and type:
java -version
This should display the version of Java installed.
- Create a simple Java program (
HelloWorld.java
):public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, Java!"); } }
- Compile and run the program using:
javac HelloWorld.java java HelloWorld
- Open the terminal or command prompt and type:
4. Setting Up Essential Tools: Editors and IDEs
A text editor or IDE (Integrated Development Environment) is a vital tool for writing and debugging your code. Popular choices include:
- Visual Studio Code (VSCode): A lightweight, free editor that supports Python