Learning Kotlin for Android development
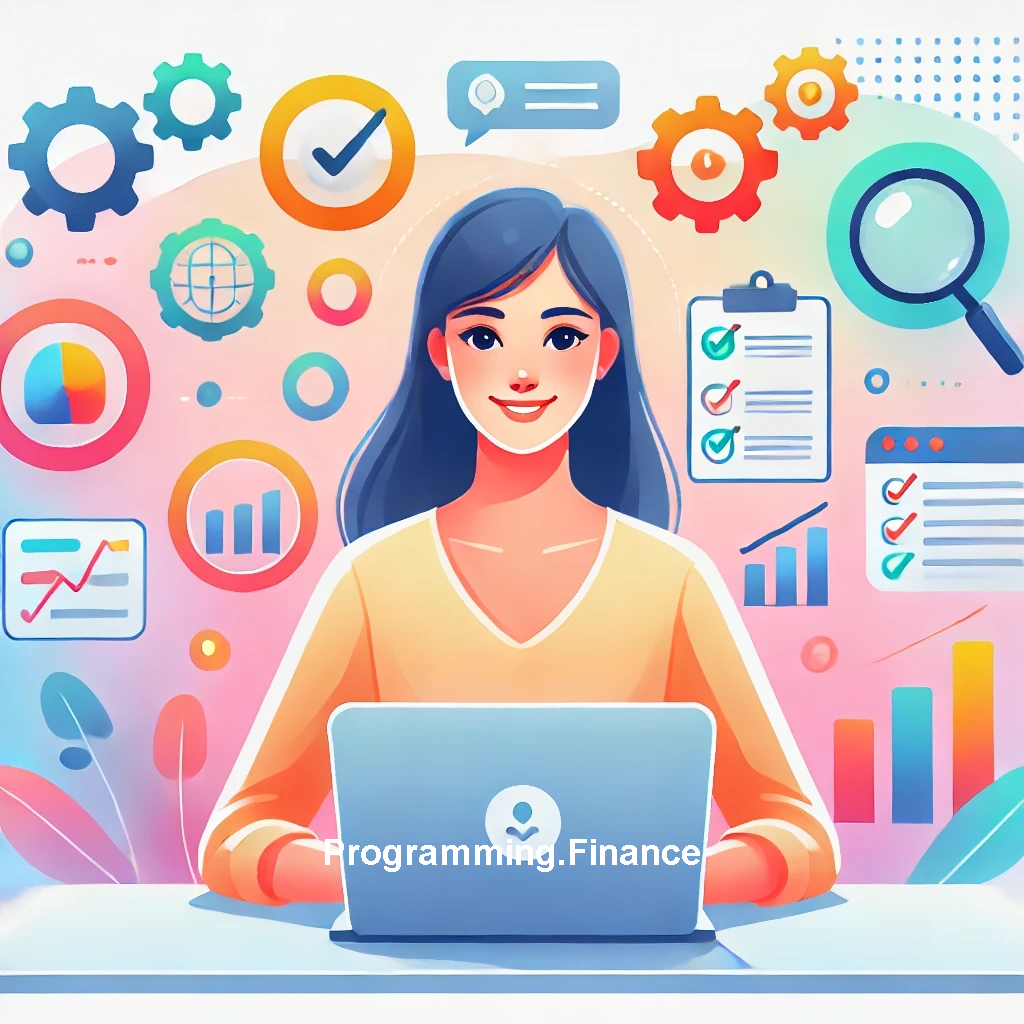
Learning Kotlin for Android Development: A Comprehensive Guide
Kotlin has swiftly become the preferred language for Android development, celebrated for its modern features, safety, and seamless interoperability with Java. Since Google announced official support for Kotlin in 2017, developers worldwide have embraced it to build robust, efficient, and maintainable Android applications. This comprehensive guide delves into the fundamentals of Learning Kotlin for Android Development, equipping you with the knowledge and skills to excel in the dynamic world of mobile app creation.
Table of Contents
- Introduction to Kotlin
- What is Kotlin?
- Why Choose Kotlin for Android Development?
- Kotlin vs. Java
- Setting Up the Development Environment
- Installing Android Studio
- Configuring Kotlin in Android Studio
- Creating Your First Kotlin Project
- Kotlin Basics
- Variables and Data Types
- Functions
- Control Flow
- Collections
- Object-Oriented Programming in Kotlin
- Classes and Objects
- Inheritance
- Interfaces and Abstract Classes
- Data Classes
- Advanced Kotlin Features
- Null Safety
- Extension Functions
- Lambda Expressions and Higher-Order Functions
- Coroutines for Asynchronous Programming
- Kotlin for Android Development
- Understanding Android Architecture Components
- Building User Interfaces with Kotlin
- Handling User Input and Events
- Networking and Data Persistence
- Building Your First Android App with Kotlin
- Project Structure
- Creating Activities and Fragments
- Implementing Navigation
- Connecting to APIs
- Persisting Data with Room
- Best Practices for Kotlin Android Development
- Writing Clean and Readable Code
- Leveraging Kotlin’s Features
- Optimizing Performance
- Testing and Debugging
- Deploying Your Kotlin Android App
- Preparing for Release
- Publishing to the Google Play Store
- Conclusion
- Additional Resources
Introduction to Kotlin
What is Kotlin?
Kotlin is a statically-typed, modern programming language developed by JetBrains, the creators of the renowned IntelliJ IDEA IDE. Released in 2011, Kotlin has gained immense popularity, especially after Google announced official support for it in Android development in 2017. Designed to be concise, expressive, and safe, Kotlin addresses many of the shortcomings of Java, making it an ideal choice for developing Android applications.
Key Features of Kotlin:
- Conciseness: Reduces boilerplate code, allowing developers to write more with less.
- Safety: Eliminates entire classes of errors, such as null pointer exceptions.
- Interoperability: Seamlessly integrates with Java, allowing the use of existing Java libraries and frameworks.
- Modern Syntax: Incorporates features like lambda expressions, extension functions, and coroutines.
- Tool-Friendly: Fully supported by major IDEs like Android Studio and IntelliJ IDEA.
Why Choose Kotlin for Android Development?
Choosing Kotlin for Android development offers numerous advantages that enhance productivity and code quality:
- Official Support by Google:
- Kotlin is officially supported by Google for Android development, ensuring robust tooling and integration with Android APIs.
- Concise Syntax:
- Kotlin’s concise syntax reduces the amount of boilerplate code, making development faster and codebases easier to maintain.
- Null Safety:
- Kotlin’s type system distinguishes between nullable and non-nullable types, significantly reducing the risk of null pointer exceptions.
- Interoperability with Java:
- Kotlin is 100% interoperable with Java, allowing developers to leverage existing Java libraries and frameworks without issues.
- Modern Features:
- Features like extension functions, lambda expressions, and coroutines enable writing expressive and efficient code.
- Enhanced Productivity:
- Kotlin’s features and tooling support increase developer productivity, enabling faster development cycles.
Kotlin vs. Java
While Java has been the cornerstone of Android development for decades, Kotlin brings several improvements that address Java’s limitations:
Feature | Java | Kotlin |
---|---|---|
Conciseness | Verbose, requires more boilerplate code | Concise, reduces boilerplate |
Null Safety | Prone to NullPointerExceptions | Built-in null safety features |
Extension Functions | Not supported | Supported, allows adding methods to classes |
Lambda Expressions | Supported (since Java 8) | Fully integrated and more flexible |
Coroutines | Not natively supported | Supported for asynchronous programming |
Interoperability | Natively supported | 100% interoperable with Java |
Data Classes | Not supported | Supported, automatically provides boilerplate |
Type Inference | Limited type inference | Advanced type inference |
Example: Null Safety in Kotlin vs. Java
Java:
String name = null;
System.out.println(name.length()); // Throws NullPointerException
Kotlin:
var name: String? = null
println(name?.length) // Safely prints null without throwing an exception
Kotlin’s approach to null safety enhances code reliability and reduces runtime errors, making it a superior choice for modern Android development.
Setting Up the Development Environment
To begin your journey in Learning Kotlin for Android Development, setting up a robust and efficient development environment is crucial. This section guides you through installing and configuring the necessary tools, ensuring a smooth start to your Kotlin programming endeavors.
Installing Android Studio
Android Studio is the official Integrated Development Environment (IDE) for Android development, providing comprehensive tools for building, testing, and deploying Android applications. It is built on IntelliJ IDEA and offers robust support for Kotlin.
Steps to Install Android Studio:
- Download Android Studio:
- Visit the Android Studio Download Page.
- Click on the Download Android Studio button and follow the prompts to download the installer suitable for your operating system (Windows, macOS, or Linux).
- Run the Installer:
- Windows:
- Double-click the downloaded
.exe
file. - Follow the installation wizard, accepting the terms and selecting the installation location.
- Double-click the downloaded
- macOS:
- Open the downloaded
.dmg
file. - Drag and drop Android Studio into the Applications folder.
- Open the downloaded
- Linux:
- Extract the downloaded
.tar.gz
file. - Move the extracted folder to a desired location (e.g.,
/usr/local/android-studio
).
- Extract the downloaded
- Windows:
- Complete the Installation:
- Launch Android Studio.
- On the first run, it will prompt you to import previous settings. Choose Do not import settings if setting up for the first time.
- Follow the setup wizard, which includes downloading the Android SDK, configuring the emulator, and installing necessary plugins.
- Update Android Studio:
- Ensure that you have the latest version by checking Help > Check for Updates (Windows/Linux) or Android Studio > Check for Updates (macOS).
Key Features of Android Studio:
- Intelligent Code Editor: Offers code completion, refactoring, and analysis.
- Layout Editor: Drag-and-drop interface for designing user interfaces.
- Emulator: Test your apps on a variety of virtual devices.
- Profiler: Analyze app performance, memory usage, and network activity.
- Version Control Integration: Seamlessly integrates with Git and other version control systems.
Configuring Kotlin in Android Studio
Android Studio comes with built-in support for Kotlin, making it easy to integrate Kotlin into your Android projects.
Steps to Enable Kotlin:
- Create a New Project:
- Open Android Studio.
- Click on „Start a new Android Studio project”.
- Choose a project template (e.g., Empty Activity) and click Next.
- Configure Your Project:
- Name: Enter your application name (e.g., MyKotlinApp).
- Package Name: Define a unique package name.
- Save Location: Choose where to save your project.
- Language: Select Kotlin from the dropdown menu.
- Minimum SDK: Choose the lowest Android version you want to support.
- Click Finish.
- Verify Kotlin Setup:
- Android Studio automatically sets up Kotlin for new projects. To verify:
- Open a Kotlin file (e.g.,
MainActivity.kt
). - Ensure that the Kotlin syntax is highlighted correctly.
- Check the
build.gradle
(Module: app) file to see Kotlin dependencies.
- Open a Kotlin file (e.g.,
- Android Studio automatically sets up Kotlin for new projects. To verify:
Example: build.gradle (Module: app)
plugins {
id 'com.android.application'
id 'kotlin-android'
}
android {
// Configuration settings
}
dependencies {
implementation "org.jetbrains.kotlin:kotlin-stdlib:$kotlin_version"
// Other dependencies
}
Kotlin’s seamless integration with Android Studio ensures that you can leverage Kotlin’s features without additional configuration, streamlining your development workflow.
Creating Your First Kotlin Project
Creating your first Kotlin-based Android project is a straightforward process, thanks to Android Studio’s intuitive interface and built-in Kotlin support.
Steps to Create Your First Kotlin Project:
- Launch Android Studio:
- Open Android Studio and select „Start a new Android Studio project”.
- Select a Project Template:
- Choose „Empty Activity” for simplicity and click Next.
- Configure Project Details:
- Name: Enter a project name (e.g., HelloKotlin).
- Package Name: Ensure it follows the standard naming convention (e.g., com.example.hellokotlin).
- Save Location: Choose a directory to save your project.
- Language: Select Kotlin.
- Minimum SDK: Choose the minimum Android version you intend to support.
- Click Finish.
- Explore the Project Structure:
- MainActivity.kt: Contains the main activity code in Kotlin.
- activity_main.xml: Defines the UI layout using XML.
- AndroidManifest.xml: Configures essential app metadata and components.
- Run the App:
- Connect an Android device or start an emulator.
- Click the Run button or press Shift + F10.
- The app will launch, displaying the default layout.
Example: Modifying MainActivity.kt
package com.example.hellokotlin
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.TextView
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// Set the layout for the activity
setContentView(R.layout.activity_main)
// Find the TextView by its ID and set a custom message
val textView: TextView = findViewById(R.id.text_view)
textView.text = "Hello, Kotlin!"
}
}
Explanation:
- Imports: Imports necessary classes from Android libraries.
- MainActivity Class: Inherits from
AppCompatActivity
and overrides theonCreate
method. - setContentView: Sets the XML layout for the activity.
- findViewById: Locates the
TextView
by its ID and updates its text to „Hello, Kotlin!”.
Modifying the MainActivity.kt
file allows you to personalize your app, reinforcing the foundational concepts of Kotlin programming within an Android context.
Kotlin Basics
Mastering the basics of Kotlin is essential for developing effective Android applications. This section covers fundamental concepts, including variables, data types, functions, control flow structures, and collections.
Variables and Data Types
Variables are containers for storing data values. In Kotlin, variables can be declared using val
(immutable) or var
(mutable), promoting code safety and flexibility.
Declaring Variables:
- Immutable Variables (
val
): Once assigned, their values cannot be changed. - Mutable Variables (
var
): Their values can be updated after initialization.
Example:
val name: String = "Alice"
var age: Int = 25
// name = "Bob" // Error: Val cannot be reassigned
age = 26 // Valid
Data Types in Kotlin:
Kotlin is a statically-typed language, meaning variable types are checked at compile-time. Common data types include:
- Numeric Types:
Byte
,Short
,Int
,Long
for integer values.Float
,Double
for floating-point numbers.
- Character and Boolean:
Char
for single characters.Boolean
for true/false values.
- String:
String
for sequences of characters.
Type Inference:
Kotlin can automatically infer the type of a variable based on its assigned value, reducing the need for explicit type declarations.
Example:
val greeting = "Hello, Kotlin!" // Inferred as String
var temperature = 23.5 // Inferred as Double
Type Conversion:
Kotlin requires explicit type conversions; it does not perform implicit casting.
Example:
val intVal: Int = 10
val doubleVal: Double = intVal.toDouble() // Explicit conversion
Example: Working with Variables and Data Types
fun main() {
val firstName: String = "John"
var lastName: String = "Doe"
val fullName = "$firstName $lastName"
var score: Int = 85
score += 10 // score is now 95
println(fullName) // Output: John Doe
println("Score: $score") // Output: Score: 95
}
Explanation:
- String Templates: Use
$variable
or${expression}
to embed variables and expressions within strings. - Variable Mutation: Demonstrates updating a mutable variable (
score
).
Functions
Functions are reusable blocks of code that perform specific tasks. In Kotlin, functions are declared using the fun
keyword.
Function Syntax:
fun functionName(parameters): ReturnType {
// Function body
}
Example:
fun greet(name: String): String {
return "Hello, $name!"
}
fun main() {
val message = greet("Alice")
println(message) // Output: Hello, Alice!
}
Single-Expression Functions:
For functions that consist of a single expression, Kotlin allows concise syntax using the =
symbol.
Example:
fun add(a: Int, b: Int): Int = a + b
fun main() {
println(add(5, 3)) // Output: 8
}
Default and Named Parameters:
Kotlin supports default parameter values and named arguments, enhancing function flexibility.
Example:
fun createUser(name: String, age: Int = 18, email: String = ""): String {
return "Name: $name, Age: $age, Email: $email"
}
fun main() {
println(createUser("Bob")) // Output: Name: Bob, Age: 18, Email:
println(createUser(name = "Carol", email = "[email protected]")) // Output: Name: Carol, Age: 18, Email: [email protected]
}
Explanation:
- Default Parameters:
age
defaults to18
andemail
defaults to an empty string if not provided. - Named Arguments: Allows specifying arguments by name, improving readability.
Control Flow
Control flow structures determine the execution path of your program based on certain conditions or repeated actions.
Conditional Statements
1. If-Else Statement:
Executes a block of code if a condition is true; otherwise, executes the alternative block.
Example:
fun checkNumber(num: Int) {
if (num > 0) {
println("$num is positive.")
} else if (num < 0) {
println("$num is negative.")
} else {
println("The number is zero.")
}
}
fun main() {
checkNumber(10) // Output: 10 is positive.
checkNumber(-5) // Output: -5 is negative.
checkNumber(0) // Output: The number is zero.
}
2. When Statement:
A versatile conditional statement that replaces the switch-case found in other languages.
Example:
fun describeNumber(num: Int) {
when {
num % 2 == 0 -> println("$num is even.")
else -> println("$num is odd.")
}
}
fun main() {
describeNumber(4) // Output: 4 is even.
describeNumber(7) // Output: 7 is odd.
}
Explanation:
- When without Argument: Evaluates boolean expressions.
- Multiple Conditions: Allows complex condition checks within cases.
3. Ternary Operator:
Kotlin does not have a ternary operator; instead, if-else
can be used as an expression.
Example:
fun max(a: Int, b: Int): Int {
return if (a > b) a else b
}
fun main() {
println(max(10, 20)) // Output: 20
}
Loops
1. For Loop:
Iterates over a range, array, or collection.
Example:
fun printNumbers() {
for (i in 1..5) {
println(i)
}
}
fun main() {
printNumbers()
// Output:
// 1
// 2
// 3
// 4
// 5
}
2. While Loop:
Executes a block of code while a condition is true.
Example:
fun countdown() {
var count = 5
while (count > 0) {
println("Countdown: $count")
count--
}
}
fun main() {
countdown()
// Output:
// Countdown: 5
// Countdown: 4
// Countdown: 3
// Countdown: 2
// Countdown: 1
}
3. Do-While Loop:
Executes a block of code at least once before checking the condition.
Example:
fun repeatAction() {
var number = 0
do {
println("Number is $number")
number++
} while (number < 3)
}
fun main() {
repeatAction()
// Output:
// Number is 0
// Number is 1
// Number is 2
}
4. Foreach Loop:
Iterates over elements in a collection.
Example:
fun listFruits() {
val fruits = listOf("Apple", "Banana", "Cherry")
for (fruit in fruits) {
println(fruit)
}
}
fun main() {
listFruits()
// Output:
// Apple
// Banana
// Cherry
}
Explanation:
- List Iteration: Loops through each fruit in the
fruits
list and prints it.
Collections
Kotlin offers powerful collection types for managing groups of related data. Understanding collections is vital for efficient data manipulation and storage.
1. Arrays:
Fixed-size collections that store elements of the same type.
Example:
fun arrayExample() {
val numbers = arrayOf(1, 2, 3, 4, 5)
println(numbers[0]) // Output: 1
// Iterating through an array
for (number in numbers) {
println(number)
}
}
fun main() {
arrayExample()
// Output:
// 1
// 1
// 2
// 3
// 4
// 5
}
2. Lists:
Dynamic collections that can grow or shrink in size.
Example:
fun listExample() {
val fruits = mutableListOf("Apple", "Banana", "Cherry")
fruits.add("Date")
fruits.remove("Banana")
for (fruit in fruits) {
println(fruit)
}
}
fun main() {
listExample()
// Output:
// Apple
// Cherry
// Date
}
3. Sets:
Collections that contain unique elements, preventing duplicates.
Example:
fun setExample() {
val numbers = mutableSetOf(1, 2, 3, 2, 1)
numbers.add(4)
numbers.remove(3)
for (number in numbers) {
println(number)
}
}
fun main() {
setExample()
// Output:
// 1
// 2
// 4
}
4. Maps:
Collections of key-value pairs, allowing efficient data retrieval based on keys.
Example:
fun mapExample() {
val userAges = mutableMapOf("Alice" to 30, "Bob" to 25)
userAges["Carol"] = 28
userAges.remove("Bob")
for ((name, age) in userAges) {
println("$name is $age years old.")
}
}
fun main() {
mapExample()
// Output:
// Alice is 30 years old.
// Carol is 28 years old.
}
Explanation:
- Adding Elements: Use
add
,remove
, and direct assignment to manage map entries. - Iteration: Destructure key-value pairs for easy access during iteration.
5. Other Collections:
Kotlin also supports other collection types like Queues
, Stacks
, and more through the standard library and additional frameworks.
Object-Oriented Programming in Kotlin
Kotlin is inherently an object-oriented programming (OOP) language, enabling developers to create modular, reusable, and maintainable code through classes and objects. This section explores the core OOP principles in Kotlin, including classes, inheritance, interfaces, and polymorphism.
Classes and Objects
Classes are blueprints for creating objects, encapsulating data (properties) and behaviors (methods).
Defining a Class:
class Car {
// Properties
var make: String = ""
var model: String = ""
var year: Int = 0
// Method
fun startEngine() {
println("The engine of $make $model has started.")
}
}
Creating Objects:
fun main() {
val myCar = Car()
myCar.make = "Toyota"
myCar.model = "Camry"
myCar.year = 2020
myCar.startEngine() // Output: The engine of Toyota Camry has started.
}
Primary Constructor:
Kotlin allows defining a primary constructor within the class header.
Example:
class Person(val name: String, var age: Int) {
fun introduce() {
println("Hi, I'm $name and I'm $age years old.")
}
}
fun main() {
val person = Person("Alice", 30)
person.introduce() // Output: Hi, I'm Alice and I'm 30 years old.
}
Explanation:
- Primary Constructor: Declared in the class header, initializing
name
andage
. - Properties:
name
is immutable (val
), whileage
is mutable (var
). - Method:
introduce
prints a personalized message.
Inheritance
Inheritance allows a class (subclass) to inherit properties and methods from another class (superclass), promoting code reuse and hierarchical relationships.
Example:
open class Animal(val name: String) {
open fun makeSound() {
println("$name makes a sound.")
}
}
class Dog(name: String) : Animal(name) {
override fun makeSound() {
println("$name barks.")
}
}
class Cat(name: String) : Animal(name) {
override fun makeSound() {
println("$name meows.")
}
}
fun main() {
val dog = Dog("Buddy")
val cat = Cat("Whiskers")
dog.makeSound() // Output: Buddy barks.
cat.makeSound() // Output: Whiskers meows.
}
Explanation:
open
Keyword: Allows a class or method to be inherited or overridden.- Subclassing:
Dog
andCat
inherit fromAnimal
and override themakeSound
method. - Polymorphism: Subclasses provide specific implementations of methods defined in the superclass.
Interfaces and Abstract Classes
Interfaces define a contract of methods and properties that implementing classes must fulfill. Unlike abstract classes, interfaces cannot hold state (properties with backing fields).
Defining an Interface:
interface Drivable {
fun drive()
fun stop()
}
Implementing an Interface:
class Motorcycle : Drivable {
override fun drive() {
println("Motorcycle is driving.")
}
override fun stop() {
println("Motorcycle has stopped.")
}
}
fun main() {
val bike = Motorcycle()
bike.drive() // Output: Motorcycle is driving.
bike.stop() // Output: Motorcycle has stopped.
}
Abstract Classes:
Abstract classes can hold state and provide implementations for some methods while leaving others abstract for subclasses to implement.
Example:
abstract class Vehicle(val brand: String) {
abstract fun start()
fun displayBrand() {
println("Brand: $brand")
}
}
class Truck(brand: String, val capacity: Int) : Vehicle(brand) {
override fun start() {
println("Truck $brand with capacity $capacity tons is starting.")
}
}
fun main() {
val truck = Truck("Volvo", 15)
truck.displayBrand() // Output: Brand: Volvo
truck.start() // Output: Truck Volvo with capacity 15 tons is starting.
}
Explanation:
- Abstract Class:
Vehicle
cannot be instantiated and contains an abstract methodstart
. - Concrete Class:
Truck
inherits fromVehicle
and provides an implementation forstart
. - Reusable Code:
displayBrand
is implemented in the abstract class and reused by subclasses.
Data Classes
Data classes in Kotlin are specialized classes designed to hold data. They automatically provide implementations for common methods like toString()
, equals()
, hashCode()
, and copy()
.
Defining a Data Class:
data class User(val id: Int, val name: String, val email: String)
Using Data Classes:
fun main() {
val user1 = User(1, "Alice", "[email protected]")
val user2 = user1.copy(name = "Alicia")
println(user1) // Output: User(id=1, name=Alice, [email protected])
println(user2) // Output: User(id=1, name=Alicia, [email protected])
}
Explanation:
- Automatic Methods:
toString
,equals
,hashCode
, andcopy
are auto-generated. - Immutability: While properties can be mutable (
var
) or immutable (val
), data classes promote immutability by default.
Benefits of Data Classes:
- Less Boilerplate Code: Reduces the need to manually implement common methods.
- Destructuring Declarations: Allows easy extraction of properties.
Example:
fun main() {
val user = User(2, "Bob", "[email protected]")
val (id, name, email) = user
println("ID: $id, Name: $name, Email: $email")
// Output: ID: 2, Name: Bob, Email: [email protected]
}
Advanced Kotlin Features
Kotlin offers a suite of advanced features that enhance code safety, readability, and performance. Understanding these features is essential for building sophisticated Android applications.
Null Safety
Null pointer exceptions are a common source of runtime errors in many programming languages. Kotlin addresses this issue with its robust null safety system, distinguishing between nullable and non-nullable types.
Nullable Types:
By default, types in Kotlin are non-nullable. To allow a variable to hold null
, append a ?
to its type.
Example:
var name: String = "Alice"
// name = null // Error: Null can not be a value of a non-null type String
var nullableName: String? = "Bob"
nullableName = null // Valid
Safe Calls (?.
):
Safely accesses properties or methods on nullable variables without throwing exceptions.
Example:
val length: Int? = nullableName?.length
println(length) // Output: null
Elvis Operator (?:
):
Provides a default value if the expression on the left is null
.
Example:
val safeName: String = nullableName ?: "Unknown"
println(safeName) // Output: Unknown
Not-Null Assertion (!!
):
Forces a nullable variable to be treated as non-null, throwing an exception if it is null
.
Example:
val nonNullName: String = nullableName!!
println(nonNullName) // Throws NullPointerException if nullableName is null
Example:
fun main() {
var name: String? = "Charlie"
println(name?.length) // Output: 7
name = null
println(name?.length) // Output: null
val safeName = name ?: "Guest"
println(safeName) // Output: Guest
// Uncommenting the next line will throw a NullPointerException
// println(name!!.length)
}
Explanation:
- Safe Calls: Prevents null pointer exceptions by safely accessing properties.
- Elvis Operator: Ensures a fallback value if the original value is
null
. - Not-Null Assertion: Use with caution, as it can lead to runtime exceptions.
Extension Functions
Extension functions allow you to add new functions to existing classes without modifying their source code. This feature enhances code readability and reusability.
Defining Extension Functions:
fun String.isEmailValid(): Boolean {
return this.contains("@") && this.contains(".")
}
Using Extension Functions:
fun main() {
val email = "[email protected]"
println(email.isEmailValid()) // Output: true
val invalidEmail = "userexample.com"
println(invalidEmail.isEmailValid()) // Output: false
}
Explanation:
- Function Definition:
isEmailValid
is added to theString
class. - Usage: Can be called as if it were a native method of the
String
class.
Benefits:
- Enhanced Readability: Makes code more intuitive and expressive.
- Reusability: Promotes DRY (Don’t Repeat Yourself) principles by centralizing common functionality.
Lambda Expressions and Higher-Order Functions
Lambda expressions are anonymous functions that can be treated as values—passed as arguments, returned from other functions, or assigned to variables. Higher-order functions are functions that take other functions as parameters or return them.
Lambda Expression Syntax:
val sum: (Int, Int) -> Int = { a, b -> a + b }
println(sum(5, 3)) // Output: 8
Higher-Order Function Example:
fun operate(a: Int, b: Int, operation: (Int, Int) -> Int): Int {
return operation(a, b)
}
fun main() {
val multiply = { x: Int, y: Int -> x * y }
println(operate(4, 5, multiply)) // Output: 20
}
Using Lambdas with Collections:
Kotlin’s collection API extensively uses lambdas for operations like map
, filter
, and reduce
.
Example:
fun main() {
val numbers = listOf(1, 2, 3, 4, 5)
val doubled = numbers.map { it * 2 }
val evenNumbers = numbers.filter { it % 2 == 0 }
println(doubled) // Output: [2, 4, 6, 8, 10]
println(evenNumbers) // Output: [2, 4]
}
Explanation:
map
: Transforms each element using the provided lambda.filter
: Selects elements that satisfy the lambda condition.
Example: Chaining Higher-Order Functions
fun main() {
val words = listOf("Kotlin", "Java", "Swift", "Python")
val result = words
.filter { it.length > 4 }
.map { it.toUpperCase() }
.sorted()
println(result) // Output: [KOTLIN, PYTHON, SWIFT]
}
Explanation:
filter
: Retains words with more than 4 characters.map
: Converts each word to uppercase.sorted
: Sorts the list alphabetically.
Coroutines for Asynchronous Programming
Coroutines are a powerful feature in Kotlin that simplify asynchronous programming by allowing code to be written sequentially while performing non-blocking operations. They are essential for tasks like network requests, database operations, and handling user input without freezing the UI.
Basic Coroutine Example:
import kotlinx.coroutines.*
fun main() = runBlocking {
launch {
delay(1000L)
println("World!")
}
println("Hello,")
}
Output:
Hello,
World!
Explanation:
runBlocking
: Blocks the main thread until all coroutines inside it complete.launch
: Starts a new coroutine without blocking the main thread.delay
: Suspends the coroutine for the specified time without blocking the thread.
Example: Performing Network Requests with Coroutines
import kotlinx.coroutines.*
import java.net.URL
fun main() = runBlocking {
val data = async { fetchData("https://api.example.com/data") }
println("Fetching data...")
println("Data received: ${data.await()}")
}
suspend fun fetchData(url: String): String {
return withContext(Dispatchers.IO) {
URL(url).readText()
}
}
Explanation:
async
: Starts a coroutine that returns a result.await
: Retrieves the result of theasync
coroutine.withContext(Dispatchers.IO)
: Switches the coroutine context to perform I/O operations.
Benefits of Coroutines:
- Simplicity: Enables writing asynchronous code in a sequential manner.
- Performance: Efficiently manages multiple tasks without overwhelming system resources.
- Scalability: Easily scales to handle complex asynchronous workflows.
Kotlin for Android Development
Kotlin’s integration with Android development enhances the efficiency and quality of app creation. This section explores how Kotlin aligns with Android’s architecture components, UI building, event handling, and data management, providing a solid foundation for developing feature-rich Android applications.
Understanding Android Architecture Components
Android Architecture Components provide a set of libraries that help you design robust, testable, and maintainable apps. They integrate seamlessly with Kotlin, promoting best practices and modern app architectures.
Key Architecture Components:
- ViewModel:
- Manages UI-related data in a lifecycle-conscious way.
- Survives configuration changes, such as screen rotations.
- LiveData:
- A lifecycle-aware data holder that allows UI components to observe changes.
- Ensures updates occur only when the UI is active.
- Room:
- An abstraction layer over SQLite, facilitating database management.
- Integrates with Kotlin coroutines for asynchronous operations.
- Navigation Component:
- Simplifies in-app navigation, handling fragment transactions and back stack management.
Example: Using ViewModel and LiveData
// UserViewModel.kt
import androidx.lifecycle.LiveData
import androidx.lifecycle.MutableLiveData
import androidx.lifecycle.ViewModel
class UserViewModel : ViewModel() {
private val _username = MutableLiveData<String>()
val username: LiveData<String> = _username
fun updateUsername(newName: String) {
_username.value = newName
}
}
// MainActivity.kt
import androidx.activity.viewModels
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import androidx.lifecycle.Observer
import android.widget.TextView
class MainActivity : AppCompatActivity() {
private val userViewModel: UserViewModel by viewModels()
private lateinit var usernameTextView: TextView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// Assume activity_main.xml has a TextView with ID 'username_text_view'
setContentView(R.layout.activity_main)
usernameTextView = findViewById(R.id.username_text_view)
// Observe LiveData
userViewModel.username.observe(this, Observer { newName ->
usernameTextView.text = newName
})
// Update username
userViewModel.updateUsername("Alice")
}
}
Explanation:
- ViewModel:
UserViewModel
holds theusername
LiveData. - LiveData Observation:
MainActivity
observes changes tousername
and updates the UI accordingly. - Lifecycle Awareness: LiveData ensures updates occur only when the activity is in an active state.
Building User Interfaces with Kotlin
Kotlin enhances Android UI development by providing expressive syntax and seamless integration with Android’s XML-based layouts. Additionally, Kotlin extensions and Android’s Jetpack Compose (a modern toolkit for building native UI) further streamline the UI creation process.
1. XML Layouts with Kotlin:
Traditional Android UI development involves defining layouts in XML and interacting with them in Kotlin code.
Example: activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:orientation="vertical"
android:layout_height="match_parent"
android:padding="16dp">
<TextView
android:id="@+id/username_text_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Username"
android:textSize="18sp" />
<Button
android:id="@+id/update_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Update Username" />
</LinearLayout>
Interacting with XML Layouts in Kotlin:
// MainActivity.kt
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.Button
import android.widget.TextView
class MainActivity : AppCompatActivity() {
private lateinit var usernameTextView: TextView
private lateinit var updateButton: Button
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// Inflate the XML layout
setContentView(R.layout.activity_main)
// Initialize views
usernameTextView = findViewById(R.id.username_text_view)
updateButton = findViewById(R.id.update_button)
// Set button click listener
updateButton.setOnClickListener {
usernameTextView.text = "Updated Username"
}
}
}
Explanation:
- View Binding: Using
findViewById
to link XML views with Kotlin code. - Event Handling: Setting up click listeners to respond to user interactions.
2. Jetpack Compose: A Modern Toolkit for UI Development
Jetpack Compose is Android’s modern toolkit for building native UI, offering a declarative approach that simplifies and accelerates UI development.
Example: Basic Jetpack Compose UI
// MainActivity.kt
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.material.*
import androidx.compose.runtime.*
import androidx.compose.ui.tooling.preview.Preview
import com.example.myapp.ui.theme.MyAppTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// Set the Compose content
setContent {
MyAppTheme {
Surface(color = MaterialTheme.colors.background) {
Greeting("Android")
}
}
}
}
}
@Composable
fun Greeting(name: String) {
var text by remember { mutableStateOf("Hello, $name!") }
Column {
Text(text = text, style = MaterialTheme.typography.h5)
Button(onClick = { text = "Hello, Jetpack Compose!" }) {
Text("Update Greeting")
}
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
MyAppTheme {
Greeting("Android")
}
}
Explanation:
- Composable Functions:
@Composable
functions define UI components. - State Management:
remember
andmutableStateOf
manage UI state reactively. - Declarative UI: Describes what the UI should look like, allowing Compose to handle the rendering.
Benefits of Jetpack Compose:
- Declarative Syntax: Simplifies UI development by focusing on the desired outcome.
- Less Boilerplate: Reduces the need for XML layouts and findViewById.
- Real-Time Previews: Enables instant previews of UI components within Android Studio.
- Integration with Kotlin: Leverages Kotlin’s language features for more expressive and concise code.
Handling User Input and Events
Managing user interactions is pivotal for creating responsive and intuitive Android applications. Kotlin, combined with Android’s frameworks, offers robust mechanisms for handling various types of user input and events.
1. Text Input:
Capturing and processing user input through text fields.
Example:
// activity_main.xml
<EditText
android:id="@+id/input_edit_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Enter your name" />
<Button
android:id="@+id/submit_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Submit" />
<TextView
android:id="@+id/display_text_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello!" />
// MainActivity.kt
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.Button
import android.widget.EditText
import android.widget.TextView
class MainActivity : AppCompatActivity() {
private lateinit var inputEditText: EditText
private lateinit var submitButton: Button
private lateinit var displayTextView: TextView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// Inflate the XML layout
setContentView(R.layout.activity_main)
// Initialize views
inputEditText = findViewById(R.id.input_edit_text)
submitButton = findViewById(R.id.submit_button)
displayTextView = findViewById(R.id.display_text_view)
// Set button click listener
submitButton.setOnClickListener {
val name = inputEditText.text.toString()
displayTextView.text = "Hello, $name!"
}
}
}
Explanation:
- EditText: Captures user input.
- Button: Triggers an action upon being clicked.
- TextView: Displays the processed input.
2. Gesture Handling:
Responding to touch gestures like taps, swipes, and drags.
Example:
// MainActivity.kt
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.view.GestureDetector
import android.view.MotionEvent
import android.widget.Toast
class MainActivity : AppCompatActivity(), GestureDetector.OnGestureListener {
private lateinit var gestureDetector: GestureDetector
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// Set a simple view
setContentView(R.layout.activity_main)
gestureDetector = GestureDetector(this, this)
}
override fun onTouchEvent(event: MotionEvent?): Boolean {
gestureDetector.onTouchEvent(event)
return super.onTouchEvent(event)
}
override fun onDown(e: MotionEvent?): Boolean {
Toast.makeText(this, "Down", Toast.LENGTH_SHORT).show()
return true
}
override fun onShowPress(e: MotionEvent?) {}
override fun onSingleTapUp(e: MotionEvent?): Boolean {
Toast.makeText(this, "Single Tap Up", Toast.LENGTH_SHORT).show()
return true
}
override fun onScroll(e1: MotionEvent?, e2: MotionEvent?, distanceX: Float, distanceY: Float): Boolean {
Toast.makeText(this, "Scrolling", Toast.LENGTH_SHORT).show()
return true
}
override fun onLongPress(e: MotionEvent?) {
Toast.makeText(this, "Long Press", Toast.LENGTH_SHORT).show()
}
override fun onFling(e1: MotionEvent?, e2: MotionEvent?, velocityX: Float, velocityY: Float): Boolean {
Toast.makeText(this, "Fling", Toast.LENGTH_SHORT).show()
return true
}
}
Explanation:
- GestureDetector: Detects various touch gestures.
- Gesture Listener: Implements
OnGestureListener
to respond to gestures like down, tap, scroll, and fling. - Toast Messages: Provide immediate feedback upon gesture detection.
3. Handling Click Events:
Responding to button clicks and other UI interactions.
Example:
fun main() {
// Assume button is initialized
button.setOnClickListener {
// Handle button click
println("Button clicked!")
}
}
Explanation:
setOnClickListener
: Registers a callback to be invoked when the button is clicked.- Lambda Expression: Defines the action to perform upon the click event.
4. Implementing RecyclerView Clicks:
Handling clicks on items within a RecyclerView, a common UI component for displaying lists.
Example:
// ItemAdapter.kt
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import androidx.recyclerview.widget.RecyclerView
class ItemAdapter(
private val items: List<String>,
private val clickListener: (String) -> Unit
) : RecyclerView.Adapter<ItemAdapter.ViewHolder>() {
inner class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
// Assume itemView has a TextView with ID 'item_text_view'
val textView: TextView = itemView.findViewById(R.id.item_text_view)
fun bind(item: String) {
textView.text = item
itemView.setOnClickListener { clickListener(item) }
}
}
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ViewHolder {
val view = LayoutInflater.from(parent.context)
.inflate(R.layout.item_layout, parent, false)
return ViewHolder(view)
}
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
holder.bind(items[position])
}
override fun getItemCount(): Int = items.size
}
// MainActivity.kt
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import androidx.recyclerview.widget.LinearLayoutManager
import androidx.recyclerview.widget.RecyclerView
import android.widget.Toast
class MainActivity : AppCompatActivity() {
private lateinit var recyclerView: RecyclerView
private lateinit var adapter: ItemAdapter
private val items = listOf("Item 1", "Item 2", "Item 3")
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// Inflate the layout containing RecyclerView
setContentView(R.layout.activity_main)
recyclerView = findViewById(R.id.recycler_view)
adapter = ItemAdapter(items) { item ->
Toast.makeText(this, "Clicked: $item", Toast.LENGTH_SHORT).show()
}
recyclerView.layoutManager = LinearLayoutManager(this)
recyclerView.adapter = adapter
}
}
Explanation:
- ItemAdapter: Binds data to RecyclerView items and handles click events via a lambda function.
- MainActivity: Sets up the RecyclerView with the adapter and defines the click behavior.
Building Your First Android App with Kotlin
Putting theory into practice, building your first Android app with Kotlin solidifies your understanding and equips you with hands-on experience. This section guides you through the process of creating a simple Android application, covering project structure, activities, fragments, navigation, API integration, and data persistence.
Project Structure
Understanding the Android project structure is essential for efficient development. An Android project comprises various directories and files, each serving a specific purpose.
Key Components:
app/src/main/java/
: Contains Kotlin source files organized by package names.app/src/main/res/
: Holds resources like layouts (.xml
), drawables (images), and values (strings.xml
,colors.xml
).AndroidManifest.xml
: Declares essential information about the app, such as activities, permissions, and services.build.gradle
: Gradle build scripts manage dependencies and project configurations.
Example: Basic Project Structure
MyKotlinApp/
├── app/
│ ├── src/
│ │ ├── main/
│ │ │ ├── java/com/example/mykotlinapp/
│ │ │ │ ├── MainActivity.kt
│ │ │ ├── res/
│ │ │ │ ├── layout/
│ │ │ │ │ ├── activity_main.xml
│ │ │ │ ├── values/
│ │ │ │ │ ├── strings.xml
│ │ │ ├── AndroidManifest.xml
├── build.gradle
Creating Activities and Fragments
Activities and Fragments are fundamental components for building user interfaces in Android. An Activity represents a single screen, while a Fragment is a modular section of an Activity, promoting reusable UI components.
1. Creating an Activity:
// MainActivity.kt
package com.example.mykotlinapp
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// Set the XML layout for the activity
setContentView(R.layout.activity_main)
}
}
2. Creating a Fragment:
// ExampleFragment.kt
package com.example.mykotlinapp
import androidx.fragment.app.Fragment
import android.os.Bundle
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
class ExampleFragment : Fragment() {
override fun onCreateView(
inflater: LayoutInflater, container: ViewGroup?,
savedInstanceState: Bundle?
): View? {
// Inflate the XML layout for the fragment
return inflater.inflate(R.layout.fragment_example, container, false)
}
}
Explanation:
- MainActivity: Hosts the main UI defined in
activity_main.xml
. - ExampleFragment: Represents a reusable UI component defined in
fragment_example.xml
.
Implementing Navigation
Efficient navigation enhances user experience by enabling seamless transitions between different parts of the app. Android’s Navigation Component simplifies implementing navigation patterns.
1. Adding Navigation Dependencies:
Add the following dependencies to your build.gradle
(Module: app) file:
dependencies {
// Other dependencies
def nav_version = "2.5.3"
implementation "androidx.navigation:navigation-fragment-ktx:$nav_version"
implementation "androidx.navigation:navigation-ui-ktx:$nav_version"
}
2. Creating a Navigation Graph:
Create a navigation graph XML file (nav_graph.xml
) in the res/navigation/
directory to define navigation paths.
Example: nav_graph.xml
<?xml version="1.0" encoding="utf-8"?>
<navigation xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
app:startDestination="@id/firstFragment">
<fragment
android:id="@+id/firstFragment"
android:name="com.example.mykotlinapp.FirstFragment"
android:label="First Fragment"
tools:layout="@layout/fragment_first">
<action
android:id="@+id/action_firstFragment_to_secondFragment"
app:destination="@id/secondFragment" />
</fragment>
<fragment
android:id="@+id/secondFragment"
android:name="com.example.mykotlinapp.SecondFragment"
android:label="Second Fragment"
tools:layout="@layout/fragment_second" />
</navigation>
3. Setting Up Navigation in MainActivity:
// MainActivity.kt
import androidx.navigation.findNavController
import androidx.navigation.ui.setupActionBarWithNavController
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// Inflate the main layout containing NavHostFragment
setContentView(R.layout.activity_main)
// Setup ActionBar with NavController
val navController = findNavController(R.id.nav_host_fragment)
setupActionBarWithNavController(navController)
}
// Handle Up button behavior
override fun onSupportNavigateUp(): Boolean {
val navController = findNavController(R.id.nav_host_fragment)
return navController.navigateUp() || super.onSupportNavigateUp()
}
}
Explanation:
- NavHostFragment: Hosts the navigation graph within the activity layout.
- Navigation Actions: Define transitions between fragments.
4. Navigating Between Fragments:
// FirstFragment.kt
package com.example.mykotlinapp
import androidx.fragment.app.Fragment
import android.os.Bundle
import android.view.*
import android.widget.Button
import androidx.navigation.fragment.findNavController
class FirstFragment : Fragment() {
override fun onCreateView(
inflater: LayoutInflater, container: ViewGroup?,
savedInstanceState: Bundle?
): View? {
return inflater.inflate(R.layout.fragment_first, container, false)
}
override fun onViewCreated(view: View, savedInstanceState: Bundle?) {
super.onViewCreated(view, savedInstanceState)
val navigateButton: Button = view.findViewById(R.id.navigate_button)
navigateButton.setOnClickListener {
findNavController().navigate(R.id.action_firstFragment_to_secondFragment)
}
}
}
Explanation:
findNavController()
: Obtains the NavController to handle navigation actions.- Button Click Listener: Triggers navigation to the second fragment upon clicking the button.
Connecting to APIs
Fetching data from external APIs is a common requirement in Android apps. Kotlin, combined with libraries like Retrofit and OkHttp, simplifies network operations.
1. Adding Retrofit Dependencies:
Add the following dependencies to your build.gradle
(Module: app) file:
dependencies {
// Other dependencies
implementation 'com.squareup.retrofit2:retrofit:2.9.0'
implementation 'com.squareup.retrofit2:converter-gson:2.9.0'
}
2. Defining a Data Model:
// Post.kt
data class Post(
val userId: Int,
val id: Int,
val title: String,
val body: String
)
3. Creating a Retrofit Service Interface:
// ApiService.kt
import retrofit2.http.GET
interface ApiService {
@GET("posts")
suspend fun getPosts(): List<Post>
}
4. Setting Up Retrofit Instance:
// RetrofitInstance.kt
import retrofit2.Retrofit
import retrofit2.converter.gson.GsonConverterFactory
object RetrofitInstance {
private const val BASE_URL = "https://jsonplaceholder.typicode.com/"
val api: ApiService by lazy {
Retrofit.Builder()
.baseUrl(BASE_URL)
.addConverterFactory(GsonConverterFactory.create())
.build()
.create(ApiService::class.java)
}
}
5. Fetching Data Using Coroutines:
// MainActivity.kt
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.Toast
import kotlinx.coroutines.*
import retrofit2.HttpException
class MainActivity : AppCompatActivity() {
private val coroutineScope = CoroutineScope(Dispatchers.Main + Job())
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// Inflate your layout
setContentView(R.layout.activity_main)
fetchPosts()
}
private fun fetchPosts() {
coroutineScope.launch {
try {
val posts = RetrofitInstance.api.getPosts()
// Handle the fetched posts (e.g., display in RecyclerView)
Toast.makeText(this@MainActivity, "Fetched ${posts.size} posts", Toast.LENGTH_SHORT).show()
} catch (e: HttpException) {
Toast.makeText(this@MainActivity, "Error: ${e.message()}", Toast.LENGTH_SHORT).show()
} catch (e: Throwable) {
Toast.makeText(this@MainActivity, "Unexpected error", Toast.LENGTH_SHORT).show()
}
}
}
override fun onDestroy() {
super.onDestroy()
coroutineScope.cancel()
}
}
Explanation:
- Retrofit Setup: Configures Retrofit with the base URL and Gson converter.
- ApiService: Defines the endpoint for fetching posts.
- Coroutines: Utilizes Kotlin coroutines to perform network requests asynchronously.
- Error Handling: Catches HTTP and unexpected exceptions, providing user feedback.
Persisting Data with Room
Room is a persistence library that provides an abstraction layer over SQLite, simplifying database management in Android apps. It integrates seamlessly with Kotlin, offering compile-time checks and reducing boilerplate code.
1. Adding Room Dependencies:
Add the following dependencies to your build.gradle
(Module: app) file:
dependencies {
// Other dependencies
implementation "androidx.room:room-runtime:2.4.3"
kapt "androidx.room:room-compiler:2.4.3"
implementation "androidx.room:room-ktx:2.4.3"
}
2. Defining an Entity:
// User.kt
import androidx.room.Entity
import androidx.room.PrimaryKey
@Entity(tableName = "users")
data class User(
@PrimaryKey val id: Int,
val name: String,
val email: String
)
3. Creating a DAO (Data Access Object):
// UserDao.kt
import androidx.room.*
@Dao
interface UserDao {
@Insert(onConflict = OnConflictStrategy.REPLACE)
suspend fun insert(user: User)
@Query("SELECT * FROM users WHERE id = :userId")
suspend fun getUserById(userId: Int): User?
@Delete
suspend fun delete(user: User)
}
4. Setting Up the Database:
// AppDatabase.kt
import androidx.room.Database
import androidx.room.Room
import androidx.room.RoomDatabase
import android.content.Context
@Database(entities = [User::class], version = 1)
abstract class AppDatabase : RoomDatabase() {
abstract fun userDao(): UserDao
companion object {
@Volatile private var INSTANCE: AppDatabase? = null
fun getDatabase(context: Context): AppDatabase =
INSTANCE ?: synchronized(this) {
INSTANCE ?: Room.databaseBuilder(
context.applicationContext,
AppDatabase::class.java,
"app_database"
).build().also { INSTANCE = it }
}
}
}
5. Using Room in Your Activity:
// MainActivity.kt
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.Button
import android.widget.Toast
import kotlinx.coroutines.*
class MainActivity : AppCompatActivity() {
private lateinit var insertButton: Button
private lateinit var fetchButton: Button
private lateinit var deleteButton: Button
private val coroutineScope = CoroutineScope(Dispatchers.Main + Job())
private lateinit var db: AppDatabase
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// Inflate your layout containing buttons
setContentView(R.layout.activity_main)
insertButton = findViewById(R.id.insert_button)
fetchButton = findViewById(R.id.fetch_button)
deleteButton = findViewById(R.id.delete_button)
db = AppDatabase.getDatabase(this)
insertButton.setOnClickListener {
coroutineScope.launch {
val user = User(1, "Alice", "[email protected]")
db.userDao().insert(user)
Toast.makeText(this@MainActivity, "User inserted", Toast.LENGTH_SHORT).show()
}
}
fetchButton.setOnClickListener {
coroutineScope.launch {
val user = db.userDao().getUserById(1)
if (user != null) {
Toast.makeText(this@MainActivity, "User: ${user.name}, ${user.email}", Toast.LENGTH_SHORT).show()
} else {
Toast.makeText(this@MainActivity, "User not found", Toast.LENGTH_SHORT).show()
}
}
}
deleteButton.setOnClickListener {
coroutineScope.launch {
val user = db.userDao().getUserById(1)
if (user != null) {
db.userDao().delete(user)
Toast.makeText(this@MainActivity, "User deleted", Toast.LENGTH_SHORT).show()
} else {
Toast.makeText(this@MainActivity, "User not found", Toast.LENGTH_SHORT).show()
}
}
}
}
override fun onDestroy() {
super.onDestroy()
coroutineScope.cancel()
}
}
Explanation:
- Entity: Defines the
User
data model mapped to theusers
table. - DAO: Provides methods for inserting, fetching, and deleting users.
- Database: Singleton instance ensures a single database throughout the app.
- Activity: Implements buttons to perform CRUD operations using coroutines.
Benefits of Room:
- Compile-Time Verification: Ensures SQL queries are correct.
- Ease of Use: Simplifies database interactions with annotated methods.
- Integration with Coroutines: Facilitates asynchronous database operations.
Example: Building a Simple To-Do App
To solidify your understanding, let’s build a simple To-Do application using Kotlin, incorporating the concepts discussed.
1. Project Setup:
- Dependencies:
- AndroidX libraries for modern Android development.
- Room for data persistence.
- Retrofit for networking (optional for advanced features).
2. Defining the Data Model:
// Todo.kt
import androidx.room.Entity
import androidx.room.PrimaryKey
@Entity(tableName = "todos")
data class Todo(
@PrimaryKey(autoGenerate = true) val id: Int = 0,
val task: String,
val isCompleted: Boolean = false
)
3. Creating the DAO:
// TodoDao.kt
import androidx.room.*
@Dao
interface TodoDao {
@Insert(onConflict = OnConflictStrategy.REPLACE)
suspend fun insert(todo: Todo)
@Query("SELECT * FROM todos ORDER BY id DESC")
suspend fun getAllTodos(): List<Todo>
@Update
suspend fun update(todo: Todo)
@Delete
suspend fun delete(todo: Todo)
}
4. Setting Up the Database:
// AppDatabase.kt
import androidx.room.Database
import androidx.room.Room
import androidx.room.RoomDatabase
import android.content.Context
@Database(entities = [Todo::class], version = 1)
abstract class AppDatabase : RoomDatabase() {
abstract fun todoDao(): TodoDao
companion object {
@Volatile private var INSTANCE: AppDatabase? = null
fun getDatabase(context: Context): AppDatabase =
INSTANCE ?: synchronized(this) {
INSTANCE ?: Room.databaseBuilder(
context.applicationContext,
AppDatabase::class.java,
"todo_database"
).build().also { INSTANCE = it }
}
}
}
5. Creating the Repository:
// TodoRepository.kt
class TodoRepository(private val todoDao: TodoDao) {
suspend fun insert(todo: Todo) = todoDao.insert(todo)
suspend fun getAllTodos(): List<Todo> = todoDao.getAllTodos()
suspend fun update(todo: Todo) = todoDao.update(todo)
suspend fun delete(todo: Todo) = todoDao.delete(todo)
}
6. Implementing ViewModel:
// TodoViewModel.kt
import androidx.lifecycle.ViewModel
import androidx.lifecycle.viewModelScope
import kotlinx.coroutines.launch
class TodoViewModel(private val repository: TodoRepository) : ViewModel() {
var todos = mutableListOf<Todo>()
fun addTodo(task: String) {
viewModelScope.launch {
val todo = Todo(task = task)
repository.insert(todo)
todos.add(0, todo.copy(id = getLastId()))
}
}
fun toggleTodoCompletion(todo: Todo) {
viewModelScope.launch {
val updatedTodo = todo.copy(isCompleted = !todo.isCompleted)
repository.update(updatedTodo)
val index = todos.indexOf(todo)
if (index != -1) {
todos[index] = updatedTodo
}
}
}
fun removeTodo(todo: Todo) {
viewModelScope.launch {
repository.delete(todo)
todos.remove(todo)
}
}
private suspend fun getLastId(): Int {
val allTodos = repository.getAllTodos()
return allTodos.firstOrNull()?.id ?: 0
}
}
Explanation:
- Repository: Acts as a mediator between the ViewModel and the DAO, handling data operations.
- ViewModel: Manages UI-related data, performing CRUD operations through the repository.
7. Building the UI:
Implementing a simple UI using RecyclerView to display the list of To-Dos.
Example: MainActivity with RecyclerView
// MainActivity.kt
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.Button
import android.widget.EditText
import androidx.lifecycle.ViewModelProvider
import androidx.recyclerview.widget.LinearLayoutManager
import androidx.recyclerview.widget.RecyclerView
class MainActivity : AppCompatActivity() {
private lateinit var todoViewModel: TodoViewModel
private lateinit var recyclerView: RecyclerView
private lateinit var adapter: TodoAdapter
private lateinit var addButton: Button
private lateinit var inputEditText: EditText
private lateinit var db: AppDatabase
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// Inflate your layout containing RecyclerView, EditText, and Button
setContentView(R.layout.activity_main)
db = AppDatabase.getDatabase(this)
val repository = TodoRepository(db.todoDao())
todoViewModel = ViewModelProvider(this, ViewModelFactory(repository)).get(TodoViewModel::class.java)
recyclerView = findViewById(R.id.recycler_view)
addButton = findViewById(R.id.add_button)
inputEditText = findViewById(R.id.input_edit_text)
adapter = TodoAdapter(todoViewModel.todos, onToggle = { todo ->
todoViewModel.toggleTodoCompletion(todo)
}, onDelete = { todo ->
todoViewModel.removeTodo(todo)
})
recyclerView.layoutManager = LinearLayoutManager(this)
recyclerView.adapter = adapter
addButton.setOnClickListener {
val task = inputEditText.text.toString()
if (task.isNotEmpty()) {
todoViewModel.addTodo(task)
inputEditText.text.clear()
adapter.notifyDataSetChanged()
}
}
// Load existing todos
loadTodos()
}
private fun loadTodos() {
lifecycleScope.launch {
todoViewModel.todos.addAll(todoViewModel.repository.getAllTodos())
adapter.notifyDataSetChanged()
}
}
}
Explanation:
- ViewModelProvider: Initializes the
TodoViewModel
with the repository. - RecyclerView Setup: Configures the RecyclerView with a
TodoAdapter
to display the list of To-Dos. - Add Button: Adds a new To-Do when clicked, updating the RecyclerView accordingly.
8. Implementing the Adapter:
// TodoAdapter.kt
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.widget.CheckBox
import android.widget.ImageButton
import android.widget.TextView
import androidx.recyclerview.widget.RecyclerView
class TodoAdapter(
private val todos: List<Todo>,
private val onToggle: (Todo) -> Unit,
private val onDelete: (Todo) -> Unit
) : RecyclerView.Adapter<TodoAdapter.ViewHolder>() {
inner class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
val taskCheckBox: CheckBox = itemView.findViewById(R.id.task_check_box)
val taskTextView: TextView = itemView.findViewById(R.id.task_text_view)
val deleteButton: ImageButton = itemView.findViewById(R.id.delete_button)
fun bind(todo: Todo) {
taskCheckBox.isChecked = todo.isCompleted
taskTextView.text = todo.task
taskTextView.paint.isStrikeThruText = todo.isCompleted
taskCheckBox.setOnCheckedChangeListener { _, _ ->
onToggle(todo)
}
deleteButton.setOnClickListener {
onDelete(todo)
}
}
}
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ViewHolder {
val view = LayoutInflater.from(parent.context)
.inflate(R.layout.item_todo, parent, false)
return ViewHolder(view)
}
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
holder.bind(todos[position])
}
override fun getItemCount(): Int = todos.size
}
Explanation:
- ViewHolder: Holds references to the UI components for each To-Do item.
- Binding Data: Updates the UI elements based on the To-Do’s properties.
- Event Handling: Triggers callbacks for toggle and delete actions.
9. Finalizing the App:
With the core components in place, running the app allows users to add, complete, and delete To-Do items, demonstrating a fully functional Android application built with Kotlin.
Best Practices for Kotlin Android Development
Adhering to best practices ensures that your Kotlin-based Android applications are robust, maintainable, and efficient. This section outlines key guidelines to elevate your development workflow and code quality.
Writing Clean and Readable Code
1. Follow Naming Conventions:
Consistent naming enhances code readability and maintainability.
- Classes and Objects: PascalCase
class UserProfile { }
- Functions and Methods: camelCase
fun calculateTotal() { }
- Variables: camelCase
val userName = "Alice"
- Constants: UPPER_SNAKE_CASE
const val MAX_USERS = 100
2. Use Descriptive Names:
Choose names that clearly describe the purpose of variables, functions, and classes.
// Good
val totalPrice: Double = 49.99
fun fetchUserData() { }
// Bad
val tp: Double = 49.99
fun fUD() { }
3. Keep Functions Short and Focused:
Each function should perform a single task, promoting reusability and easier testing.
fun calculateDiscount(price: Double, discountRate: Double): Double {
return price * discountRate
}
fun applyDiscount() {
val discountedPrice = calculateDiscount(100.0, 0.2)
println("Discounted Price: $discountedPrice")
}
4. Utilize Kotlin’s Features for Conciseness:
Leverage features like type inference, smart casts, and data classes to write more concise code.
// Using type inference
val userName = "Bob"
// Smart casts
fun getStringLength(obj: Any): Int? {
if (obj is String) {
return obj.length
}
return null
}
Leveraging Kotlin’s Features
1. Extension Functions:
Enhance existing classes with new functionalities without modifying their source code.
fun String.isPalindrome(): Boolean {
return this == this.reversed()
}
fun main() {
println("madam".isPalindrome()) // Output: true
println("hello".isPalindrome()) // Output: false
}
2. Higher-Order Functions and Lambdas:
Create more abstract and flexible code by passing functions as parameters or returning them.
fun performOperation(a: Int, b: Int, operation: (Int, Int) -> Int): Int {
return operation(a, b)
}
fun main() {
val sum = performOperation(5, 3) { x, y -> x + y }
println("Sum: $sum") // Output: Sum: 8
}
3. Coroutines for Asynchronous Tasks:
Handle asynchronous operations efficiently, avoiding callback hell and improving code readability.
import kotlinx.coroutines.*
fun main() = runBlocking {
launch {
delay(1000L)
println("World!")
}
println("Hello,")
}
// Output:
// Hello,
// World!
4. Data Classes for Data Representation:
Simplify the creation of classes that primarily hold data, automatically generating common methods.
data class Product(val id: Int, val name: String, val price: Double)
fun main() {
val product = Product(1, "Laptop", 999.99)
println(product) // Output: Product(id=1, name=Laptop, price=999.99)
}
Optimizing Performance
1. Efficient Resource Management:
- Use
val
Overvar
: Immutable variables (val
) can lead to better performance and thread safety. - Avoid Unnecessary Object Creation: Reuse objects when possible to reduce memory overhead.
2. Utilize Kotlin’s Lazy Initialization:
Delay the creation of objects until they are needed, saving resources.
val heavyObject by lazy {
// Initialization code
HeavyClass()
}
3. Leverage Kotlin’s Inline Functions:
Improve performance by reducing the overhead of higher-order functions.
inline fun perform(action: () -> Unit) {
action()
}
4. Optimize Collection Operations:
Use efficient data structures and avoid unnecessary iterations.
val numbers = listOf(1, 2, 3, 4, 5)
val evenNumbers = numbers.filter { it % 2 == 0 }
5. Profile Your App:
Regularly use profiling tools like Android Profiler to identify and address performance bottlenecks.
Testing and Debugging
1. Write Unit Tests:
Ensure individual components function as expected using testing frameworks like JUnit.
// Calculator.kt
class Calculator {
fun add(a: Int, b: Int): Int = a + b
}
// CalculatorTest.kt
import org.junit.Test
import org.junit.Assert.*
class CalculatorTest {
private val calculator = Calculator()
@Test
fun testAdd() {
val sum = calculator.add(2, 3)
assertEquals(5, sum)
}
}
2. Utilize Android’s Testing Frameworks:
Employ frameworks like Espresso for UI testing to validate user interactions.
// Example of an Espresso test
@RunWith(AndroidJUnit4::class)
class MainActivityTest {
@Test
fun testButtonClick() {
onView(withId(R.id.submit_button)).perform(click())
onView(withId(R.id.display_text_view)).check(matches(withText("Hello, Kotlin!")))
}
}
3. Debugging with Android Studio:
Use breakpoints, watch variables, and the debugger to identify and fix issues in your code.
- Setting Breakpoints: Click the gutter next to the code line to set a breakpoint.
- Inspecting Variables: Hover over variables during debugging to see their current values.
- Step Through Code: Navigate through your code execution using step over, step into, and step out actions.
4. Handle Exceptions Gracefully:
Implement proper exception handling to prevent app crashes and provide meaningful feedback to users.
try {
// Code that may throw an exception
} catch (e: Exception) {
// Handle the exception
Log.e("Error", "An error occurred: ${e.message}")
}
Deploying Your Kotlin Android App
After developing and thoroughly testing your Android application, the final step is deployment. Deploying your app effectively ensures that it reaches your target audience, performs reliably, and maintains user satisfaction. This section outlines the key steps involved in preparing your app for release and publishing it to the Google Play Store.
Preparing for Release
1. App Optimization:
- Minimize APK Size: Remove unused resources and use ProGuard or R8 for code shrinking and obfuscation.
- Optimize Images: Compress and resize images to reduce the app’s footprint.
- Enable Multidex: If your app exceeds the 64K method limit, enable multidex to support additional methods.
2. Versioning:
- Version Code: An integer value that represents the version of the application code, relative to other versions.
- Version Name: A string value representing the release version (e.g., „1.0.0”).
Example:
android {
defaultConfig {
applicationId "com.example.mykotlinapp"
minSdkVersion 21
targetSdkVersion 31
versionCode 1
versionName "1.0.0"
}
}
3. App Signing:
Sign your app with a secure key before releasing it to the Play Store. This ensures the authenticity and integrity of your app.
Steps to Sign Your App:
- Generate a Keystore:
- Open Android Studio.
- Navigate to Build > Generate Signed Bundle / APK.
- Select APK and click Next.
- Click Create new to generate a new keystore.
- Fill in the required details and save the keystore file securely.
- Configure Signing in Gradle:
android {
signingConfigs {
release {
keyAlias "your-key-alias"
keyPassword "your-key-password"
storeFile file("path/to/keystore.jks")
storePassword "your-store-password"
}
}
buildTypes {
release {
signingConfig signingConfigs.release
minifyEnabled true
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
}
4. ProGuard/R8 Configuration:
Use ProGuard or R8 to obfuscate and optimize your code, enhancing security and reducing app size.
Example: proguard-rules.pro
# Preserve annotations
-keepattributes *Annotation*
# Keep model classes
-keep class com.example.mykotlinapp.model.** { *; }
# Keep Retrofit interfaces
-keep interface com.example.mykotlinapp.network.** { *; }
Publishing to the Google Play Store
Publishing your app to the Google Play Store involves several steps to ensure compliance with Google’s policies and to make your app discoverable to users.
1. Create a Developer Account:
- Registration Fee: A one-time fee of $25.
- Process:
- Visit the Google Play Console.
- Sign in with your Google account.
- Complete the registration process, providing necessary information and payment details.
2. Prepare Store Listing:
- App Details:
- Title: The name of your app.
- Short Description: A brief overview of your app.
- Full Description: Detailed information about features and functionalities.
- Graphics:
- App Icon: High-resolution icon adhering to Google’s specifications.
- Screenshots: Capture app screenshots for various device sizes.
- Feature Graphic: A promotional image displayed on the store.
- Categorization:
- Application Type: Choose between App or Game.
- Category: Select an appropriate category (e.g., Productivity, Education).
- Contact Details:
- Email Address: For user inquiries and support.
- Website: Optional, but recommended for providing additional information.
3. Upload the APK or App Bundle:
- App Bundle vs. APK:
- App Bundle (.aab): Recommended as it allows Google Play to optimize the APK for each device configuration.
- APK (.apk): The traditional format for Android apps.
- Steps:
- Navigate to the „Release” section in the Play Console.
- Select „Production” and click „Create Release”.
- Upload your signed App Bundle or APK.
- Enter release notes detailing the updates or features.
4. Content Rating:
Provide accurate content ratings to ensure your app is suitable for the intended audience.
Steps:
- Navigate to „Store Presence > Content Rating”.
- Fill out the questionnaire to determine the appropriate rating.
5. App Pricing and Distribution:
- Pricing:
- Choose between Free or Paid.
- Note that once an app is free, it cannot be changed to paid.
- Distribution:
- Select the countries where you want your app to be available.
- Opt-in for distribution to specific devices or Android versions if necessary.
6. Submit for Review:
Once all sections are complete, review your app to ensure compliance with Google’s policies. Click „Review” and then „Start Rollout to Production” to submit your app for review.
7. Monitor Release:
After submission, Google reviews your app for policy compliance. This process typically takes a few hours to a few days. Monitor the release status in the Play Console and address any issues if notified.
8. Post-Release Management:
- User Feedback: Respond to user reviews and address feedback.
- Updates: Regularly update your app with new features, improvements, and bug fixes.
- Analytics: Utilize Google Play Console’s analytics to track app performance and user engagement.
Conclusion
Learning Kotlin for Android Development empowers you to build modern, efficient, and high-quality Android applications. Kotlin’s concise syntax, robust features, and seamless interoperability with Java make it an indispensable tool in the Android developer’s arsenal. By mastering the basics of Kotlin—from variables and data types to advanced features like coroutines and extension functions—you lay a solid foundation for developing sophisticated and responsive Android apps.
Throughout this guide, we’ve explored essential aspects of Kotlin programming tailored for Android development, including setting up the development environment, understanding core language constructs, leveraging object-oriented principles, and utilizing Android Architecture Components. Additionally, practical examples and best practices have been provided to enhance your coding proficiency and ensure your applications are performant, maintainable, and user-friendly.
Embrace Kotlin’s capabilities, stay updated with the latest advancements, and continuously refine your skills to excel in the ever-evolving landscape of Android development. Whether you’re building simple utilities or complex enterprise solutions, Kotlin equips you with the tools and paradigms necessary to create impactful and innovative mobile applications.
Additional Resources
To further enhance your understanding and proficiency in Learning Kotlin for Android Development, the following resources are invaluable:
Official Documentation and Tutorials
- Kotlin Official Documentation: Comprehensive guide covering all aspects of Kotlin. Visit Documentation
- Android Developer Kotlin Guides: Official Android guides and best practices using Kotlin. Explore Kotlin on Android
- Jetpack Compose Documentation: Learn about Jetpack Compose for modern UI development. Visit Compose Docs
Books
- „Kotlin in Action” by Dmitry Jemerov and Svetlana Isakova: An in-depth exploration of Kotlin features and best practices.
- „Head First Kotlin” by Dawn Griffiths and David Griffiths: A beginner-friendly book with interactive exercises.
- „Android Programming with Kotlin for Beginners” by John Horton: Guides newcomers through Android app development using Kotlin.
Online Courses
- Udemy’s „Kotlin for Android Developers”: Comprehensive course covering Kotlin fundamentals and Android development. Enroll Now
- Coursera’s „Android App Development with Kotlin”: Structured program from top universities. Start Learning
- Pluralsight’s Kotlin Path: A series of courses focused on Kotlin programming. Explore Courses
Community and Support
- Kotlin Slack: Join the official Kotlin Slack workspace for real-time discussions. Invite Link
- Stack Overflow Kotlin Tag: Ask questions and find answers related to Kotlin. Browse Questions
- Reddit’s r/Kotlin: Engage with the Kotlin community, share projects, and seek advice. Visit r/Kotlin
- Kotlin Forums: Participate in discussions about Kotlin language features and usage. Visit Kotlin Forums
Tools and Utilities
- IntelliJ IDEA: JetBrains’ powerful IDE with advanced Kotlin support. Download IntelliJ
- Kotlin Playground: An online tool to write and run Kotlin code snippets. Try Kotlin Playground
- Retrofit: A type-safe HTTP client for Android and Java. Learn More
- Room Persistence Library: Abstraction layer over SQLite for database management. Explore Room
Blogs and Articles
- Kotlin Blog: Official blog with updates, tutorials, and best practices. Visit Kotlin Blog
- Android Developers Blog: Insights and announcements related to Android development. Read Android Blog
- Kotlin by Example: Practical examples to learn Kotlin programming. Explore Examples
Podcasts
- Talking Kotlin: Discussions on Kotlin features, usage, and community news. Listen Here
- Kotlin Podcast: Interviews with Kotlin developers and experts. Listen Here
- Android Developers Backstage: Deep dives into Android development topics. Listen Here
Conferences and Events
- KotlinConf: The official Kotlin conference featuring talks from language creators and community experts. Learn More
- Droidcon: Global Android developer conference with sessions on Kotlin and Android development. Visit Droidcon
- Google I/O: Annual developer conference with sessions on Android and Kotlin. Attend Google I/O
Sample Projects
- To-Do App: A comprehensive example integrating Room, ViewModel, LiveData, and Coroutines. View on GitHub
- Kotlin Coroutines Samples: Explore various coroutine-based examples. Visit GitHub
- Jetpack Compose Samples: Learn UI development with Jetpack Compose. Explore Samples
Embarking on Learning Kotlin for Android Development equips you with the tools and knowledge to create impactful and modern Android applications. Kotlin’s synergy with Android’s architecture components, combined with its advanced language features, fosters the development of efficient, maintainable, and user-friendly apps. Utilize the insights and resources provided in this guide to deepen your Kotlin proficiency and excel in the competitive landscape of Android development.