What are the basic principles of programming?
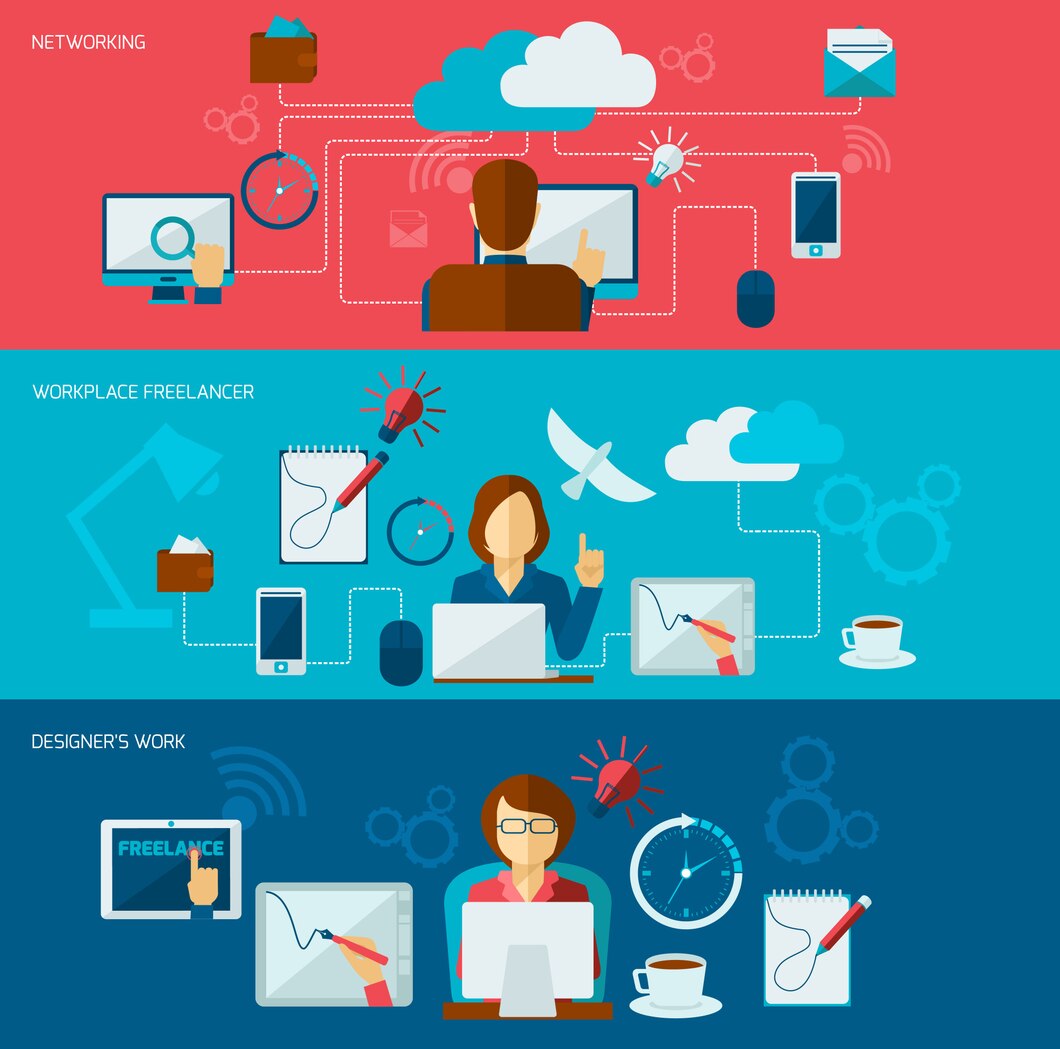
Understanding the Basic Principles of Programming: A Comprehensive Guide
Programming is the backbone of the digital world, enabling the creation of software, applications, and systems that drive our daily lives. Whether you’re a beginner embarking on your coding journey or someone looking to solidify your foundational knowledge, understanding the basic principles of programming is essential. This guide delves into these fundamental concepts, ensuring you have a robust framework to build upon.
Table of Contents
- Introduction to Programming
- 1. Abstraction and Modularity
- 2. Algorithms and Logic
- 3. Variables and Data Types
- 4. Control Structures
- 5. Data Structures
- 6. Syntax and Semantics
- 7. Debugging and Testing
- 8. Documentation and Commenting
- Conclusion
Introduction to Programming
Programming involves writing instructions that a computer can execute to perform specific tasks. These instructions are written in various programming languages, each with its syntax and semantics. At its core, programming is about problem-solving and creating efficient, effective solutions through code. Understanding the basic principles of programming not only makes you a better coder but also enhances your ability to think logically and systematically.
1. Abstraction and Modularity
Abstraction
Abstraction is the process of simplifying complex systems by breaking them down into more manageable parts. In programming, abstraction allows developers to focus on high-level operations without getting bogged down by intricate details. For example, when using a sorting function, you don’t need to understand the underlying algorithm; you just need to know how to call the function and pass the necessary parameters.
Modularity
Modularity refers to dividing a program into separate, interchangeable components or modules. Each module handles a specific aspect of the program’s functionality. This approach enhances code readability, maintainability, and reusability. For instance, in a web application, you might have separate modules for user authentication, data processing, and user interface management.
2. Algorithms and Logic
Algorithms
An algorithm is a step-by-step procedure or formula for solving a problem. In programming, algorithms are essential for performing tasks such as searching, sorting, and data manipulation. A well-designed algorithm ensures that a program runs efficiently and effectively.
Logical Thinking
Logical thinking is the ability to reason systematically and make connections between different pieces of information. In programming, logical thinking helps in constructing algorithms, debugging code, and optimizing performance. It involves understanding cause and effect, identifying patterns, and anticipating potential issues.
3. Variables and Data Types
Variables
Variables are storage locations in a program that hold data. They have names (identifiers) and can store different types of information, such as numbers, text, or more complex data structures. Variables allow programmers to manipulate and manage data dynamically.
Data Types
Data types define the kind of data a variable can hold. Common data types include:
- Integer: Whole numbers without decimals.
- Float/Double: Numbers with decimal points.
- String: Sequences of characters, such as text.
- Boolean: True or false values.
- Arrays and Lists: Collections of items.
- Objects: Complex data structures that can contain multiple data types.
Understanding data types is crucial for performing operations correctly and efficiently in a program.
4. Control Structures
Control structures dictate the flow of a program’s execution. They allow developers to implement decision-making, looping, and branching within code. The primary control structures include:
Conditional Statements
- If-Else Statements: Execute code blocks based on whether a condition is true or false.
if temperature > 30: print("It's hot outside!") else: print("It's comfortable outside.")
- Switch Statements: Handle multiple conditions by matching a variable against different cases (available in some languages).
Loops
- For Loops: Iterate over a sequence of elements a specific number of times.
for (let i = 0; i < 5; i++) { console.log(i); }
- While Loops: Continue executing a block of code as long as a condition remains true.
while (count < 10) { count++; }
Branching Statements
- Break: Exits the current loop or switch statement.
- Continue: Skips the current iteration and moves to the next one.
5. Data Structures
Data structures are ways of organizing and storing data to enable efficient access and modification. They are fundamental to designing effective algorithms and software solutions. Common data structures include:
Arrays
An array is a collection of elements identified by index or key. They are used to store multiple items of the same type.
int numbers[5] = {1, 2, 3, 4, 5};
Linked Lists
A linked list is a sequence of nodes where each node points to the next one, allowing for dynamic memory allocation.
Stacks and Queues
- Stack: Last-In-First-Out (LIFO) structure.
- Queue: First-In-First-Out (FIFO) structure.
Trees and Graphs
- Trees: Hierarchical structures with nodes connected by edges.
- Graphs: Collections of nodes connected by edges, used to represent networks.
Hash Tables
Hash tables store key-value pairs and provide efficient data retrieval based on keys.
6. Syntax and Semantics
Syntax
Syntax refers to the set of rules that define the structure of valid statements in a programming language. Proper syntax ensures that code is written in a way that the compiler or interpreter can understand and execute.
Example of correct syntax in Python:
def greet(name):
print(f"Hello, {name}!")
Semantics
Semantics deals with the meaning of the syntactical elements. It ensures that the code not only follows the structural rules but also performs the intended operations.
For example, the semantic meaning of the greet
function is to print a greeting message to the user.
7. Debugging and Testing
Debugging
Debugging is the process of identifying and fixing errors or bugs in a program. Effective debugging requires understanding the code, reproducing issues, and systematically isolating the problem.
Common debugging techniques include:
- Code Review: Examining code for mistakes.
- Print Statements: Outputting variable values at different stages.
- Debugging Tools: Using integrated development environment (IDE) features to step through code.
Testing
Testing ensures that a program behaves as expected. It involves verifying that the code meets requirements and identifying any defects.
Types of testing include:
- Unit Testing: Testing individual components or functions.
- Integration Testing: Ensuring that different modules work together.
- System Testing: Validating the complete and integrated software.
- Acceptance Testing: Confirming the software meets user needs.
Automated testing tools can streamline the testing process, making it more efficient and reliable.
8. Documentation and Commenting
Documentation
Documentation provides detailed information about the code, its functionality, and usage. It is crucial for maintaining and scaling software projects, especially when multiple developers are involved.
Effective documentation includes:
- Code Comments: Brief explanations within the code.
- API Documentation: Detailed descriptions of functions, classes, and modules.
- User Guides: Instructions for end-users on how to use the software.
Commenting
Commenting involves adding notes within the code to explain complex logic or decisions. Good comments enhance code readability and assist future developers in understanding the codebase.
Example of commenting in JavaScript:
// Function to calculate the factorial of a number
function factorial(n) {
if (n === 0) {
return 1;
}
return n * factorial(n - 1);
}
Conclusion
Mastering the basic principles of programming is essential for anyone looking to excel in the field of software development. From understanding abstraction and modularity to implementing effective algorithms and data structures, these foundational concepts pave the way for creating efficient, scalable, and maintainable software solutions. Additionally, embracing best practices in debugging, testing, and documentation ensures that your code not only works but also stands the test of time. Whether you’re coding for fun or building the next big application, a solid grasp of these programming principles will serve as your guide to success.
By adhering to these fundamental principles, programmers can create robust and efficient software while maintaining high standards of code quality. Whether you’re a novice or an experienced developer, continually reinforcing these basics will enhance your coding proficiency and adaptability in an ever-evolving technological landscape.