What are the essential data structures and when to use them?
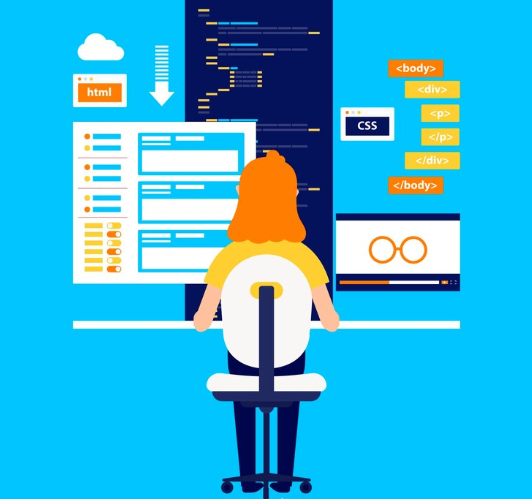
What Are the Essential Data Structures and When to Use Them? Comprehensive Guide
Understanding data structures is fundamental to effective programming and software development. They are the backbone of efficient algorithms and play a critical role in organizing and managing data. Whether you’re a budding developer, preparing for technical interviews, or aiming to enhance your coding skills, mastering essential data structures and knowing when to use them is crucial. This comprehensive guide delves into the most essential data structures, their characteristics, and their optimal use cases, ensuring you build a strong foundation in computer science. Optimized for SEO, this article seamlessly integrates key terms to provide valuable insights and enhance search engine visibility naturally.
Table of Contents
- Introduction
- Essential Data Structures
- 1. Arrays
- 2. Linked Lists
- 3. Stacks
- 4. Queues
- 5. Hash Tables
- 6. Trees
- 7. Graphs
- 8. Heaps
- 9. Tries
- Choosing the Right Data Structure
- Conclusion
- Additional SEO Tips for Your Data Structures Guide
Introduction
Data structures are specialized formats for organizing, processing, and storing data efficiently. They are pivotal in enabling programs to handle data effectively, optimize performance, and solve complex problems. From simple arrays to complex graphs, each data structure has its unique strengths and ideal use cases. By mastering these essential data structures, you enhance your ability to write optimized code, perform efficient data manipulation, and develop scalable applications.
Essential Data Structures
1. Arrays
Arrays are one of the most fundamental and widely used data structures in programming. They store elements in contiguous memory locations, allowing efficient access to elements using indices.
- Characteristics:
- Fixed size.
- Elements of the same data type.
- Direct access to elements via indices.
- When to Use Arrays:
- When you need fast access to elements using indices.
- For storing a collection of elements that do not change in size.
- In scenarios where memory usage needs to be minimal and predictable.
- Example:
# Python Array Example fruits = ["Apple", "Banana", "Cherry"] print(fruits[1]) # Output: Banana
2. Linked Lists
Linked Lists consist of nodes where each node contains data and a reference (or link) to the next node in the sequence. Unlike arrays, linked lists are dynamic and can easily grow or shrink in size.
- Characteristics:
- Dynamic size.
- Sequential access.
- Each node contains data and a pointer to the next node.
- When to Use Linked Lists:
- When frequent insertions and deletions are required.
- When the size of the data structure needs to be dynamic.
- In applications where memory allocation needs flexibility.
- Example:
// Java LinkedList Example LinkedList<String> fruits = new LinkedList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Cherry"); System.out.println(fruits.get(1)); // Output: Banana
3. Stacks
Stacks follow the Last-In-First-Out (LIFO) principle, where the last element added is the first one to be removed. They are used in scenarios that require reversing actions or maintaining history.
- Characteristics:
- LIFO order.
- Operations: Push (add), Pop (remove), Peek (retrieve top element).
- When to Use Stacks:
- Implementing undo mechanisms in applications.
- Parsing expressions (e.g., evaluating mathematical expressions).
- Managing function calls in recursion.
- Example:
// JavaScript Stack Example let stack = []; stack.push("Apple"); stack.push("Banana"); console.log(stack.pop()); // Output: Banana
4. Queues
Queues adhere to the First-In-First-Out (FIFO) principle, where the first element added is the first one to be removed. They are ideal for scenarios that require order preservation.
- Characteristics:
- FIFO order.
- Operations: Enqueue (add), Dequeue (remove), Front (retrieve first element).
- When to Use Queues:
- Managing tasks in scheduling systems.
- Handling requests in web servers.
- Implementing breadth-first search in graph algorithms.
- Example:
// C# Queue Example Queue<string> queue = new Queue<string>(); queue.Enqueue("Apple"); queue.Enqueue("Banana"); Console.WriteLine(queue.Dequeue()); // Output: Apple
5. Hash Tables
Hash Tables store key-value pairs and provide efficient insertion, deletion, and lookup operations. They use a hash function to compute an index into an array of buckets.
- Characteristics:
- Average-case time complexity of O(1) for insertions and lookups.
- Handles collisions using techniques like chaining or open addressing.
- Unordered data storage.
- When to Use Hash Tables:
- Implementing dictionaries or maps.
- Caching data for quick retrieval.
- Counting occurrences of items (e.g., word frequency).
- Example:
# Python Dictionary (Hash Table) Example fruits = {"Apple": 1, "Banana": 2, "Cherry": 3} print(fruits["Banana"]) # Output: 2
6. Trees
Trees are hierarchical data structures consisting of nodes connected by edges. Each tree has a root node and child nodes, forming a parent-child relationship.
- Characteristics:
- Hierarchical structure.
- No cycles.
- Efficient for representing hierarchical data.
- Types of Trees:
- Binary Trees: Each node has at most two children.
- Binary Search Trees (BST): A binary tree with ordered nodes for efficient searching.
- AVL Trees: Self-balancing binary search trees.
- Heaps: Specialized trees used for priority queues.
- When to Use Trees:
- Representing hierarchical data (e.g., file systems, organizational charts).
- Implementing efficient search and sort operations.
- Managing priority queues with heaps.
- Example:
// Java Binary Search Tree Example class Node { int key; Node left, right; public Node(int item) { key = item; left = right = null; } } class BinarySearchTree { Node root; void insert(int key) { root = insertRec(root, key); } Node insertRec(Node root, int key) { if (root == null) { root = new Node(key); return root; } if (key < root.key) root.left = insertRec(root.left, key); else if (key > root.key) root.right = insertRec(root.right, key); return root; } }
7. Graphs
Graphs are collections of nodes (vertices) connected by edges. They can be directed or undirected and are used to represent complex relationships between entities.
- Characteristics:
- Can represent many-to-many relationships.
- Directed or undirected edges.
- Can contain cycles.
- Types of Graphs:
- Directed Graphs (Digraphs): Edges have a direction.
- Undirected Graphs: Edges have no direction.
- Weighted Graphs: Edges carry weights.
- Unweighted Graphs: Edges do not carry weights.
- When to Use Graphs:
- Modeling networks (e.g., social networks, communication networks).
- Solving pathfinding problems (e.g., GPS navigation).
- Representing dependencies (e.g., task scheduling).
- Example:
// C++ Graph Representation Using Adjacency List #include <bits/stdc++.h> using namespace std; class Graph { int V; vector<vector<int>> adj; public: Graph(int V) { this->V = V; adj.resize(V); } void addEdge(int u, int v) { adj[u].push_back(v); adj[v].push_back(u); // For undirected graph } void printGraph() { for(int i = 0; i < V; ++i) { cout << "Vertex " << i << ": "; for(auto x : adj[i]) cout << x << " "; cout << endl; } } }; int main() { Graph g(5); g.addEdge(0, 1); g.addEdge(0, 4); g.addEdge(1, 2); g.addEdge(1, 3); g.addEdge(1, 4); g.addEdge(2, 3); g.addEdge(3, 4); g.printGraph(); return 0; }
8. Heaps
Heaps are specialized tree-based data structures that satisfy the heap property. In a max heap, for any given node C, if P is a parent node of C, then the key of P is greater than or equal to the key of C. In a min heap, the key of P is less than or equal to the key of C.
- Characteristics:
- Complete binary tree.
- Efficiently supports priority queue operations.
- Heap property ensures the root is either the maximum or minimum element.
- When to Use Heaps:
- Implementing priority queues.
- Heap sort algorithm.
- Finding the k-th largest or smallest elements.
- Example:
# Python Heap Example using heapq import heapq heap = [] heapq.heappush(heap, 3) heapq.heappush(heap, 1) heapq.heappush(heap, 4) heapq.heappush(heap, 2) while heap: print(heapq.heappop(heap)) # Output: 1, 2, 3, 4
9. Tries
Tries, also known as prefix trees, are tree-like data structures used to store a dynamic set of strings where keys are usually strings. They are highly efficient for retrieval operations.
- Characteristics:
- Each node represents a character of a string.
- Common prefixes are shared among strings.
- Can efficiently support operations like search, insert, and delete.
- When to Use Tries:
- Implementing autocomplete features.
- Spell checking.
- IP routing.
- Example:
// JavaScript Trie Implementation class TrieNode { constructor() { this.children = {}; this.isEndOfWord = false; } } class Trie { constructor() { this.root = new TrieNode(); } insert(word) { let node = this.root; for (let char of word) { if (!node.children[char]) { node.children[char] = new TrieNode(); } node = node.children[char]; } node.isEndOfWord = true; } search(word) { let node = this.root; for (let char of word) { if (!node.children[char]) { return false; } node = node.children[char]; } return node.isEndOfWord; } } // Usage let trie = new Trie(); trie.insert("apple"); console.log(trie.search("apple")); // Output: true console.log(trie.search("app")); // Output: false
Choosing the Right Data Structure
Selecting the appropriate data structure is pivotal for writing efficient and optimized code. Consider the following factors when making your choice:
- Data Size and Type: The nature and volume of data dictate which data structure is most suitable.
- Operation Requirements: Assess the frequency and types of operations (e.g., insertions, deletions, searches) needed.
- Performance Constraints: Consider time and space complexities relevant to your application’s performance needs.
- Memory Usage: Ensure the data structure aligns with your memory availability and constraints.
- Ease of Implementation: Some data structures are easier to implement and manage than others, depending on the programming language and available libraries.
Example Scenario:
- Fast Lookups: Use hash tables or binary search trees for quick data retrieval.
- Order Preservation: Utilize arrays or linked lists when maintaining the order of elements is crucial.
- Priority Queues: Implement heaps to efficiently manage elements based on priority.
- Prefix-Based Searches: Opt for tries when dealing with string-based data and prefix searches.
Conclusion
Mastering essential data structures is a cornerstone of effective programming and software development. From simple arrays to complex graphs, each data structure offers unique advantages tailored to specific use cases. By understanding their characteristics and optimal use cases, you can design and implement efficient algorithms, optimize performance, and solve complex problems with ease. Whether you’re preparing for technical interviews, developing scalable applications, or enhancing your coding skills, a solid grasp of data structures empowers you to excel in the ever-evolving field of computer science.
Additional SEO Tips for Your Data Structures Guide
To ensure this guide ranks well on Google and attracts your target audience naturally, implement the following SEO strategies:
1. Keyword Optimization
Integrate relevant keywords seamlessly within the content. Primary keywords include:
- “essential data structures”
- “key data structures in programming”
- “when to use data structures”
- “data structures and their uses”
- “programming data structures guide”
Secondary keywords can include:
- “arrays in programming”
- “linked lists explained”
- “hash tables vs. trees”
- “stack and queue uses”
- “trie data structure”
2. Meta Tags
Craft a compelling meta title and description incorporating primary keywords.
Example:
<head>
<title>What Are the Essential Data Structures and When to Use Them? Comprehensive Guide</title>
<meta name="description" content="Discover the essential data structures in programming and learn when to use each one effectively. Our comprehensive guide covers arrays, linked lists, stacks, queues, hash tables, trees, graphs, and more to enhance your coding skills.">
</head>
3. Header Tags
Use a clear hierarchy with header tags (H1, H2, H3) to structure the content, enhancing readability and SEO.
- H1: What Are the Essential Data Structures and When to Use Them? Comprehensive Guide
- H2: Arrays
- H3: When to Use Arrays
4. Internal and External Linking
- Internal Links: Link to related articles or guides on your website, such as “Introduction to Algorithms,” “Choosing Your First Programming Language,” or “Preparing for IT Job Interviews.”
- External Links: Reference authoritative sources like GeeksforGeeks, MDN Web Docs, or Wikipedia to add credibility.
5. Mobile Optimization
Ensure the guide is fully responsive, providing a seamless experience across all devices. Use responsive design principles and test on various screen sizes to enhance user experience.
6. Page Speed
Optimize your website’s loading speed to improve user experience and SEO rankings. Techniques include:
- Compressing Images: Use tools like TinyPNG to reduce image sizes without losing quality.
- Minifying CSS and JavaScript: Remove unnecessary characters to decrease file sizes.
- Leveraging Browser Caching: Store frequently accessed resources locally on users’ devices.
7. Readable URLs
Use clear and descriptive URLs that include relevant keywords.
Example:
https://yourwebsite.com/essential-data-structures
8. Engaging Content
Enhance user engagement by incorporating:
- Visuals: Use high-quality images, diagrams, and infographics to complement the text.
- Code Snippets: Provide clear and formatted code examples to illustrate data structures.
- Interactive Elements: Embed live coding platforms like CodePen or JSFiddle for hands-on practice.
9. Schema Markup
Implement structured data (Schema.org) to help search engines understand your content better, potentially enhancing search visibility.
Example:
{
"@context": "https://schema.org",
"@type": "Article",
"headline": "What Are the Essential Data Structures and When to Use Them? Comprehensive Guide",
"description": "A detailed guide outlining the essential data structures in programming, including arrays, linked lists, stacks, queues, hash tables, trees, graphs, and more. Learn when to use each data structure effectively to enhance your coding skills.",
"author": {
"@type": "Person",
"name": "Your Name"
},
"datePublished": "2024-04-27",
"publisher": {
"@type": "Organization",
"name": "Your Website Name",
"logo": {
"@type": "ImageObject",
"url": "https://yourwebsite.com/logo.png"
}
}
}
10. Regular Updates
Keep the content fresh and up-to-date by regularly reviewing and updating the guide with the latest best practices, tools, and trends in data structures and computer science.
By implementing these SEO strategies, your guide on essential data structures will not only provide valuable information to readers but also achieve higher visibility and ranking on search engines, effectively reaching and engaging your target audience.