What are the guidelines for good coding (Code Style)?
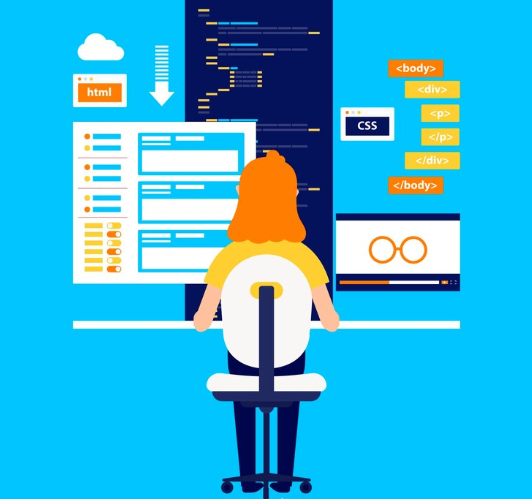
What Are the Guidelines for Good Coding (Code Style)? Comprehensive Guide
Maintaining a good coding style is essential for developing clean, efficient, and maintainable software. Whether you’re a novice programmer or an experienced developer, adhering to established coding guidelines enhances code readability, facilitates collaboration, and reduces the likelihood of errors. This comprehensive guide explores the key guidelines for good coding (code style), providing actionable insights and best practices to elevate your programming skills. Optimized for SEO, this article integrates relevant keywords naturally to ensure high visibility on search engines while delivering valuable information to readers.
Table of Contents
- Introduction
- Consistent Naming Conventions
- Proper Indentation and Formatting
- Commenting and Documentation
- Writing Readable Code
- Avoiding Code Duplication
- Writing Modular Code
- Proper Use of Variables and Data Structures
- Error Handling
- Following Language-Specific Best Practices
- Using Version Control Effectively
- Code Reviews and Testing
- Conclusion
- Additional SEO Tips for Your Code Style Guide
Introduction
Good coding style is more than just aesthetic appeal; it is a critical component of software development that ensures code is readable, maintainable, and scalable. Adhering to coding guidelines helps developers understand each other’s code, facilitates debugging, and streamlines the development process. This guide outlines essential guidelines for good coding style, offering practical examples and best practices that can be applied across various programming languages and projects.
Consistent Naming Conventions
Importance of Naming Conventions
Consistent naming conventions improve code readability and maintainability. Clear and descriptive names for variables, functions, classes, and other identifiers make it easier to understand the code’s purpose and functionality.
Best Practices
- Use Meaningful Names: Choose names that clearly describe the purpose of the variable or function.
# Good total_price = 100 # Bad tp = 100
- Follow Language Standards: Adhere to the naming conventions specific to the programming language you are using.
- CamelCase for class names in Java:
public class DataProcessor { // Class implementation }
- snake_case for variable and function names in Python:
def calculate_total(price, tax): return price + tax
- CamelCase for class names in Java:
- Avoid Abbreviations: Use full words to prevent confusion unless the abbreviation is widely recognized.
// Good let userCount = 10; // Bad let usrCnt = 10;
Proper Indentation and Formatting
Importance of Indentation
Proper indentation and formatting make the code structure clear and visually appealing, aiding in the understanding of the program’s flow.
Best Practices
- Consistent Indentation: Use spaces or tabs consistently throughout the codebase. Commonly, 4 spaces per indentation level is preferred.
# Good def greet(name): print(f"Hello, {name}!") # Bad def greet(name): print(f"Hello, {name}!")
- Limit Line Length: Keep lines of code within a reasonable length (e.g., 80-100 characters) to enhance readability.
// Good function calculateArea(width, height) { return width * height; } // Bad function calculateArea(width, height) { return width * height; }
- Use Blank Lines: Separate logical blocks of code with blank lines to improve clarity.
public void processData() { initialize(); fetchData(); executeProcessing(); finalize(); }
Commenting and Documentation
Importance of Comments
Comments and documentation provide context and explanations for the code, making it easier for others (and your future self) to understand the logic and purpose behind specific implementations.
Best Practices
- Write Clear Comments: Explain the “why” behind complex or non-obvious code segments.
// Calculate the total price including tax let totalPrice = price + (price * taxRate);
- Avoid Redundant Comments: Do not state the obvious; let the code speak for itself where possible.
# Good # Increment the counter by one counter += 1 # Bad counter += 1 # Increment counter
- Use Documentation Strings: Utilize docstrings or documentation comments to describe functions, classes, and modules.
def add(a, b): """ Adds two numbers and returns the result. Parameters: a (int): The first number. b (int): The second number. Returns: int: The sum of a and b. """ return a + b
Writing Readable Code
Importance of Readability
Readable code is easier to understand, debug, and maintain. It reduces the cognitive load on developers, enabling them to grasp the functionality quickly.
Best Practices
- Use Descriptive Variable and Function Names: As mentioned earlier, meaningful names enhance readability.
// Good int maxRetries = 5; // Bad int mr = 5;
- Break Down Complex Functions: Divide large functions into smaller, single-purpose functions.
// Good public void processOrder(Order order) { validateOrder(order); calculateTotal(order); saveOrder(order); notifyCustomer(order); } // Bad public void processOrder(Order order) { // Complex logic handling multiple tasks }
- Use Consistent Naming Styles: Maintain a uniform naming style across the entire codebase to avoid confusion.
Avoiding Code Duplication
Importance of DRY Principle
The DRY (Don’t Repeat Yourself) principle emphasizes reducing repetition in code. Eliminating duplicate code enhances maintainability and reduces the risk of inconsistencies.
Best Practices
- Refactor Common Code into Functions: Identify repetitive code patterns and abstract them into reusable functions or methods.
// Good function calculateArea(width, height) { return width * height; } let area1 = calculateArea(5, 10); let area2 = calculateArea(7, 3); // Bad let area1 = 5 * 10; let area2 = 7 * 3;
- Use Inheritance and Composition: Leverage object-oriented principles to share common functionality across classes.
class Animal: def eat(self): print("Eating") class Dog(Animal): def bark(self): print("Barking") # Avoid duplicating the eat method in Dog
- Utilize Libraries and Frameworks: Reuse existing libraries and frameworks to avoid reinventing the wheel.
Writing Modular Code
Importance of Modularity
Modular code is organized into separate, interchangeable components or modules, each handling a specific functionality. This approach enhances scalability, maintainability, and reusability.
Best Practices
- Single Responsibility Principle: Each module or class should have one responsibility or purpose.
// Good public class UserRepository { public void save(User user) { /* ... */ } } public class UserService { private UserRepository repository; public void createUser(User user) { repository.save(user); } } // Bad public class UserManager { public void createUser(User user) { /* Save user */ /* Send email */ } }
- Loose Coupling: Design modules that interact with each other through well-defined interfaces, minimizing dependencies.
# Good class Logger: def log(self, message): print(message) class UserService: def __init__(self, logger): self.logger = logger def create_user(self, user): # Create user logic self.logger.log("User created") # Bad class UserService: def create_user(self, user): # Create user logic print("User created")
- High Cohesion: Ensure that the elements within a module are closely related and work together to achieve a single goal.
Proper Use of Variables and Data Structures
Importance of Variable and Data Structure Selection
Choosing appropriate variables and data structures is crucial for writing efficient and effective code. It impacts performance, memory usage, and overall code clarity.
Best Practices
- Use Appropriate Data Types: Select data types that best fit the nature of the data and operations.
// Good let isActive = true; // Boolean for a true/false state // Bad let isActive = 1; // Using a number instead of a Boolean
- Choose the Right Data Structures: Utilize data structures that optimize performance for specific operations.
# Good from collections import deque queue = deque() # Bad queue = []
- Initialize Variables Properly: Assign meaningful initial values to variables to prevent undefined behaviors.
// Good int count = 0; // Bad int count;
Error Handling
Importance of Robust Error Handling
Effective error handling ensures that your program can gracefully handle unexpected situations, preventing crashes and providing meaningful feedback to users or developers.
Best Practices
- Use Try-Catch Blocks: Implement try-catch (or equivalent) blocks to manage exceptions and errors.
try { int result = divide(a, b); } catch (ArithmeticException e) { System.out.println("Cannot divide by zero."); }
- Provide Meaningful Error Messages: Offer clear and actionable error messages that help diagnose issues.
try: with open('file.txt', 'r') as file: data = file.read() except FileNotFoundError: print("Error: 'file.txt' not found. Please ensure the file exists in the directory.")
- Avoid Silent Failures: Do not suppress errors without handling them appropriately.
// Good try { riskyOperation(); } catch (error) { console.error("An error occurred:", error); } // Bad try { riskyOperation(); } catch (error) { // Do nothing }
Following Language-Specific Best Practices
Importance of Adhering to Language Standards
Each programming language has its own set of conventions and best practices that promote optimal coding standards. Following these guidelines ensures that your code aligns with community expectations and leverages language-specific features effectively.
Best Practices
- Understand Language Idioms: Familiarize yourself with idiomatic expressions and patterns specific to the language.
# Ruby Idiom for Iteration [1, 2, 3].each do |number| puts number end
- Leverage Language Features: Utilize built-in functions, libraries, and language constructs to write efficient code.
// Good const numbers = [1, 2, 3]; const doubled = numbers.map(num => num * 2); // Bad const numbers = [1, 2, 3]; let doubled = []; for (let i = 0; i < numbers.length; i++) { doubled.push(numbers[i] * 2); }
- Adhere to Style Guides: Follow official or widely-accepted style guides for consistency and clarity.
- PEP 8 for Python:
# Good def calculate_area(radius): return 3.14 * radius ** 2
- PEP 8 for Python:
Using Version Control Effectively
Importance of Version Control
Version control systems (VCS) like Git allow developers to track changes, collaborate with team members, and manage different versions of a codebase efficiently. Proper use of VCS enhances project management and code integrity.
Best Practices
- Commit Frequently with Clear Messages: Make small, incremental commits with descriptive messages that explain the changes.
git commit -m "Fix issue with user authentication flow"
- Use Branching Strategically: Create separate branches for features, bug fixes, and experiments to maintain a clean main codebase.
git checkout -b feature/user-login
- Merge Responsibly: Review and test code before merging branches to prevent conflicts and ensure code quality.
git merge feature/user-login
- Leverage Pull Requests: Use pull requests for code reviews and collaborative development, fostering quality and knowledge sharing.
# Pull Request Template ## Description - Added user login feature - Implemented validation checks ## Related Issues - Closes #42
Code Reviews and Testing
Importance of Code Reviews and Testing
Code reviews and testing are essential for maintaining code quality, identifying bugs, and ensuring that the software meets the desired requirements. They foster collaboration and knowledge sharing within development teams.
Best Practices
- Conduct Regular Code Reviews: Have peers review your code to catch issues early and gain diverse perspectives.
# Code Review Checklist - Does the code follow the project’s coding standards? - Are there any obvious bugs or errors? - Is the code efficient and optimized? - Are all functions and classes documented?
- Write Unit Tests: Develop tests for individual components to ensure they work as intended.
import unittest def add(a, b): return a + b class TestAddFunction(unittest.TestCase): def test_add_positive_numbers(self): self.assertEqual(add(2, 3), 5) def test_add_negative_numbers(self): self.assertEqual(add(-2, -3), -5) if __name__ == '__main__': unittest.main()
- Implement Continuous Integration (CI): Use CI tools to automate testing and integration, ensuring that code changes do not break the existing codebase.
# .github/workflows/python-app.yml name: Python application on: [push] jobs: build: runs-on: ubuntu-latest steps: - uses: actions/checkout@v2 - name: Set up Python uses: actions/setup-python@v2 with: python-version: '3.8' - name: Install dependencies run: | python -m pip install --upgrade pip pip install -r requirements.txt - name: Run tests run: | python -m unittest discover
- Adopt Test-Driven Development (TDD): Write tests before developing functionality to ensure that the code meets the specified requirements from the outset.
Conclusion
Adhering to guidelines for good coding (code style) is fundamental to producing high-quality, maintainable, and efficient software. By implementing consistent naming conventions, proper indentation, comprehensive commenting, and modular design, you enhance the readability and reliability of your code. Moreover, effective use of version control, regular code reviews, and robust testing practices ensure that your projects remain scalable and free from critical bugs. Embracing these best practices not only improves your individual coding skills but also fosters a collaborative and productive development environment. Continuously refining your coding style and staying updated with industry standards will propel you towards becoming a proficient and respected programmer.
Additional SEO Tips for Your Code Style Guide
To ensure this guide ranks well on Google and attracts your target audience naturally, implement the following SEO strategies:
1. Keyword Optimization
Integrate relevant keywords seamlessly within the content. Primary keywords include:
- “guidelines for good coding”
- “code style best practices”
- “good coding standards”
- “programming code style guidelines”
- “best coding practices”
Secondary keywords can include:
- “clean code principles”
- “naming conventions in programming”
- “code readability tips”
- “modular programming techniques”
- “version control best practices”
2. Meta Tags
Craft a compelling meta title and description incorporating primary keywords.
Example:
<head>
<title>What Are the Guidelines for Good Coding (Code Style)? Comprehensive Guide</title>
<meta name="description" content="Discover the essential guidelines for good coding (code style) with our comprehensive guide. Learn best practices, naming conventions, commenting strategies, and more to enhance your programming skills effectively.">
</head>
3. Header Tags
Use a clear hierarchy with header tags (H1, H2, H3) to structure the content, enhancing readability and SEO.
- H1: What Are the Guidelines for Good Coding (Code Style)? Comprehensive Guide
- H2: Consistent Naming Conventions
- H3: Use Meaningful Names
4. Internal and External Linking
- Internal Links: Link to related articles or guides on your website, such as “Essential Tools for Programmers,” “Popular Platforms for Learning Programming,” or “How to Effectively Use Technical Documentation.”
- External Links: Reference authoritative sources like Google’s Java Style Guide, PEP 8 for Python, or Clean Code by Robert C. Martin to add credibility.
5. Mobile Optimization
Ensure the guide is fully responsive, providing a seamless experience across all devices. Use responsive design principles and test on various screen sizes to enhance user experience.
6. Page Speed
Optimize your website’s loading speed to improve user experience and SEO rankings. Techniques include:
- Compressing Images: Use tools like TinyPNG to reduce image sizes without losing quality.
- Minifying CSS and JavaScript: Remove unnecessary characters to decrease file sizes.
- Leveraging Browser Caching: Store frequently accessed resources locally on users’ devices.
7. Readable URLs
Use clear and descriptive URLs that include relevant keywords.
Example:
https://yourwebsite.com/guidelines-good-coding-style
8. Engaging Content
Enhance user engagement by incorporating:
- Visuals: Use high-quality images, diagrams, and infographics to complement the text.
- Code Snippets: Provide clear and formatted code examples to illustrate concepts.
- Interactive Elements: Embed live coding platforms like CodePen or JSFiddle for hands-on practice.
9. Schema Markup
Implement structured data (Schema.org) to help search engines understand your content better, potentially enhancing search visibility.
Example:
{
"@context": "https://schema.org",
"@type": "Article",
"headline": "What Are the Guidelines for Good Coding (Code Style)? Comprehensive Guide",
"description": "A detailed guide outlining the essential guidelines for good coding (code style), including best practices, naming conventions, commenting strategies, and more to enhance your programming skills effectively.",
"author": {
"@type": "Person",
"name": "Your Name"
},
"datePublished": "2024-04-27",
"publisher": {
"@type": "Organization",
"name": "Your Website Name",
"logo": {
"@type": "ImageObject",
"url": "https://yourwebsite.com/logo.png"
}
}
}
10. Regular Updates
Keep the content fresh and up-to-date by regularly reviewing and updating the guide with the latest best practices, tools, and trends in coding standards and software development.
By implementing these SEO strategies, your guide on the guidelines for good coding (code style) will not only provide valuable information to readers but also achieve higher visibility and ranking on search engines, effectively reaching and engaging your target audience.