What are the key concepts in algorithms?
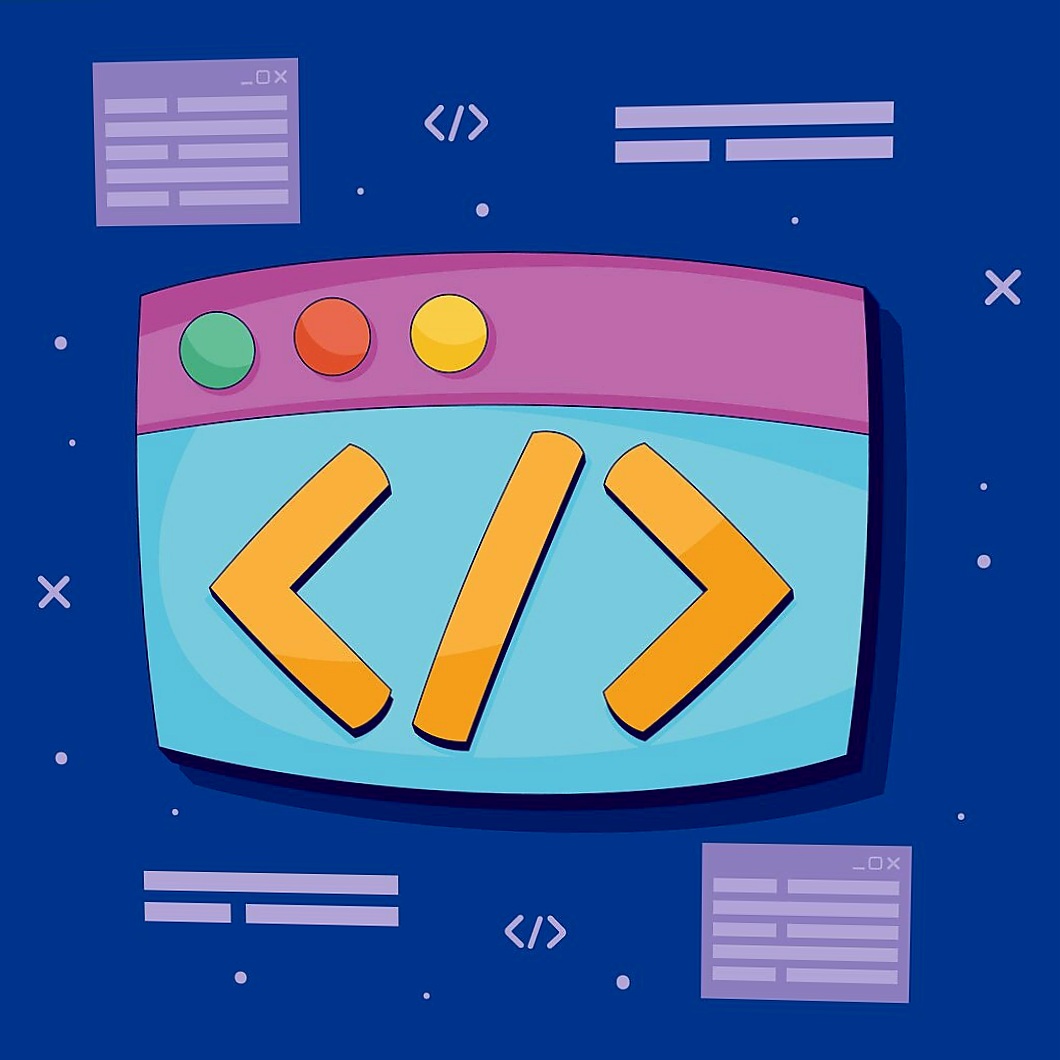
What Are the Key Concepts in Algorithms? A Comprehensive Guide
Algorithms are the heart of computer science, enabling us to solve complex problems efficiently and effectively. Whether you’re a budding programmer, a seasoned developer, or simply curious about how things work under the hood, understanding the key concepts in algorithms is essential. This comprehensive guide delves into the fundamental concepts of algorithms, providing you with the knowledge to design, analyze, and implement efficient solutions. Optimized for SEO, this article ensures you gain valuable insights into the world of algorithms naturally and seamlessly.
Table of Contents
- Introduction
- What is an Algorithm?
- Key Concepts in Algorithms
- 1. Time and Space Complexity
- 2. Big O Notation
- 3. Data Structures
- 4. Recursion
- 5. Sorting Algorithms
- 6. Searching Algorithms
- 7. Graph Algorithms
- 8. Dynamic Programming
- 9. Greedy Algorithms
- 10. Divide and Conquer
- 11. Backtracking
- 12. Hashing
- Algorithm Design Techniques
- 1. Brute Force
- 2. Heuristics
- 3. Randomized Algorithms
- Common Algorithm Problems
- 1. Sorting and Searching
- 2. Pathfinding
- 3. Optimization Problems
- 4. Combinatorial Problems
- Implementing Algorithms
- 1. Choosing the Right Language
- 2. Writing Pseudocode
- 3. Testing and Debugging
- Advanced Algorithm Concepts
- 1. Amortized Analysis
- 2. NP-Completeness
- 3. Approximation Algorithms
- Conclusion
- Additional SEO Tips for Your Algorithm Guide
Introduction
Algorithms form the backbone of computer programming and software development. They are step-by-step procedures or formulas for solving problems, performing tasks, and making decisions. From the simplest calculations to the most complex data processing tasks, algorithms are integral to the functionality of software applications. Understanding the key concepts in algorithms not only enhances your problem-solving skills but also empowers you to write efficient and optimized code.
What is an Algorithm?
An algorithm is a finite sequence of well-defined instructions typically used to solve a class of problems or perform a computation. Algorithms are fundamental to all aspects of computer science and are used in various applications, including data processing, automated reasoning, and machine learning. They can be expressed in natural language, pseudocode, or programming languages.
Example of a Simple Algorithm:
Algorithm to Find the Maximum Number in a List:
- Initialize
max
with the first element of the list. - Iterate through each number in the list.
- If the current number is greater than
max
, updatemax
. - After the iteration,
max
holds the largest number in the list.
Key Concepts in Algorithms
1. Time and Space Complexity
Time Complexity refers to the amount of time an algorithm takes to complete as a function of the input size. It helps in evaluating the efficiency of an algorithm.
Space Complexity measures the amount of memory an algorithm uses relative to the input size.
Importance:
- Helps in selecting the most efficient algorithm for a given problem.
- Critical for applications where resources are limited.
2. Big O Notation
Big O Notation is a mathematical notation used to describe the upper bound of an algorithm’s running time or space requirements in terms of input size (n).
Common Big O Classifications:
- O(1): Constant time
- O(log n): Logarithmic time
- O(n): Linear time
- O(n log n): Linearithmic time
- O(n²): Quadratic time
Example:
- Linear Search: O(n)
- Binary Search: O(log n)
3. Data Structures
Data Structures are ways of organizing and storing data to enable efficient access and modification.
Common Data Structures:
- Arrays
- Linked Lists
- Stacks
- Queues
- Trees
- Graphs
- Hash Tables
Importance:
- Choosing the right data structure can significantly impact the performance of an algorithm.
4. Recursion
Recursion is a programming technique where a function calls itself to solve smaller instances of a problem.
Key Components:
- Base Case: Condition to terminate the recursion.
- Recursive Case: Portion of the problem solved by recursive calls.
Example:
- Factorial Calculation
def factorial(n): if n == 0: return 1 else: return n * factorial(n-1)
5. Sorting Algorithms
Sorting Algorithms arrange elements in a particular order (ascending or descending).
Common Sorting Algorithms:
- Bubble Sort: O(n²)
- Selection Sort: O(n²)
- Insertion Sort: O(n²)
- Merge Sort: O(n log n)
- Quick Sort: O(n log n) average
- Heap Sort: O(n log n)
Importance:
- Fundamental for optimizing the efficiency of other algorithms (e.g., search algorithms).
6. Searching Algorithms
Searching Algorithms are used to retrieve information stored within data structures.
Common Searching Algorithms:
- Linear Search: O(n)
- Binary Search: O(log n)
Example:
- Binary Search Implementation
int binarySearch(int[] arr, int target) { int left = 0, right = arr.length - 1; while (left <= right) { int mid = left + (right - left) / 2; if (arr[mid] == target) return mid; if (arr[mid] < target) left = mid + 1; else right = mid - 1; } return -1; }
7. Graph Algorithms
Graph Algorithms are used to solve problems related to graph theory, such as finding the shortest path or detecting cycles.
Common Graph Algorithms:
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
- Dijkstra’s Algorithm
- Bellman-Ford Algorithm
- Kruskal’s and Prim’s Algorithms for Minimum Spanning Trees
Importance:
- Applicable in network routing, social networks, and recommendation systems.
8. Dynamic Programming
Dynamic Programming (DP) is an optimization technique used to solve problems by breaking them down into simpler subproblems and storing the results of these subproblems to avoid redundant computations.
Key Concepts:
- Overlapping Subproblems
- Optimal Substructure
Example:
- Fibonacci Sequence Calculation
def fibonacci(n, memo={}): if n in memo: return memo[n] if n <= 1: return n memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo) return memo[n]
9. Greedy Algorithms
Greedy Algorithms make the locally optimal choice at each step with the hope of finding a global optimum.
Common Greedy Algorithms:
- Kruskal’s Algorithm for Minimum Spanning Tree
- Prim’s Algorithm for Minimum Spanning Tree
- Dijkstra’s Algorithm for Shortest Path
- Huffman Coding
Importance:
- Efficient for optimization problems where a global optimum can be reached by choosing local optima.
10. Divide and Conquer
Divide and Conquer is an algorithm design paradigm that breaks a problem into smaller subproblems, solves each subproblem recursively, and combines their solutions to solve the original problem.
Common Divide and Conquer Algorithms:
- Merge Sort
- Quick Sort
- Binary Search
- Strassen’s Matrix Multiplication
Importance:
- Enhances algorithm efficiency by reducing problem size with each recursion.
11. Backtracking
Backtracking is a refinement of the brute force approach, where the algorithm incrementally builds candidates to the solutions and abandons a candidate (“backtracks”) as soon as it determines that the candidate cannot possibly lead to a valid solution.
Common Backtracking Problems:
- N-Queens Problem
- Sudoku Solver
- Maze Solving
Example:
- N-Queens Problem Solution
def solve_n_queens(n): def backtrack(row, diagonals, anti_diagonals, cols, state): if row == n: result.append(state.copy()) return for col in range(n): diag = row - col anti_diag = row + col if col in cols or diag in diagonals or anti_diag in anti_diagonals: continue cols.add(col) diagonals.add(diag) anti_diagonals.add(anti_diag) state.append(col) backtrack(row + 1, diagonals, anti_diagonals, cols, state) cols.remove(col) diagonals.remove(diag) anti_diagonals.remove(anti_diag) state.pop() result = [] backtrack(0, set(), set(), set(), []) return result
12. Hashing
Hashing involves mapping data of arbitrary size to fixed-size values using hash functions. It is widely used in data retrieval, encryption, and data integrity.
Common Hashing Applications:
- Hash Tables
- Cryptographic Hash Functions
- Data Deduplication
Example:
- Implementing a Simple Hash Table in Python
class HashTable: def __init__(self): self.size = 10 self.table = [[] for _ in range(self.size)] def hash_function(self, key): return hash(key) % self.size def insert(self, key, value): index = self.hash_function(key) for pair in self.table[index]: if pair[0] == key: pair[1] = value return self.table[index].append([key, value]) def get(self, key): index = self.hash_function(key) for pair in self.table[index]: if pair[0] == key: return pair[1] return None
Algorithm Design Techniques
1. Brute Force
Brute Force involves trying all possible solutions to find the correct one. While simple, it is often inefficient for large datasets.
Example:
- Finding All Subsets of a Set
2. Heuristics
Heuristics are problem-solving methods that use practical approaches to find satisfactory solutions, often faster than classic methods but without guarantees of optimality.
Example:
- A Search Algorithm in Pathfinding*
3. Randomized Algorithms
Randomized Algorithms use randomness as part of their logic, which can lead to simpler or more efficient solutions for certain problems.
Example:
- Randomized Quick Sort
Common Algorithm Problems
1. Sorting and Searching
- Sorting: Arrange data in a particular order (ascending or descending).
- Searching: Retrieve information stored within data structures.
2. Pathfinding
- Shortest Path: Finding the shortest route between two points in a graph.
- Graph Traversal: Visiting all the nodes in a graph systematically.
3. Optimization Problems
- Knapsack Problem: Maximizing value within a weight limit.
- Traveling Salesman Problem: Finding the shortest possible route visiting each city once.
4. Combinatorial Problems
- Permutations and Combinations: Arranging and selecting items.
- Subset Sum Problem: Determining if a subset of numbers sums to a target value.
Implementing Algorithms
1. Choosing the Right Language
Select a programming language that best suits the algorithm’s requirements and your proficiency. Languages like Python, Java, C++, and JavaScript are commonly used for implementing algorithms.
2. Writing Pseudocode
Before coding, write pseudocode to outline the algorithm’s logic. This helps in understanding the flow and identifying potential issues.
3. Testing and Debugging
Thoroughly test your algorithm with various test cases to ensure correctness and efficiency. Use debugging tools to identify and fix issues.
Advanced Algorithm Concepts
1. Amortized Analysis
Amortized Analysis evaluates the average time per operation over a sequence of operations, providing a more accurate measure of an algorithm’s performance in certain cases.
2. NP-Completeness
NP-Complete Problems are a class of problems for which no efficient solving algorithm is known. Understanding these helps in recognizing the complexity of certain algorithmic challenges.
3. Approximation Algorithms
Approximation Algorithms find near-optimal solutions for complex problems where finding the exact solution is computationally infeasible.
Conclusion
Understanding the key concepts in algorithms is crucial for anyone aspiring to excel in computer science and software development. From grasping the basics of time and space complexity to mastering advanced techniques like dynamic programming and graph algorithms, these concepts form the foundation of efficient problem-solving. By continually practicing and applying these concepts, you can enhance your ability to design, analyze, and implement effective algorithms that drive innovative solutions in the tech industry.
Additional SEO Tips for Your Algorithm Guide
To ensure this guide ranks well on Google and attracts your target audience naturally, implement the following SEO strategies:
1. Keyword Optimization
Integrate relevant keywords seamlessly within the content. Primary keywords include:
- “key concepts in algorithms”
- “algorithm fundamentals”
- “understanding algorithms”
- “algorithm key concepts guide”
- “essential algorithm concepts”
Secondary keywords can include:
- “time complexity”
- “data structures in algorithms”
- “sorting algorithms”
- “searching algorithms”
- “dynamic programming”
2. Meta Tags
Craft a compelling meta title and description incorporating primary keywords.
Example:
<head>
<title>What Are the Key Concepts in Algorithms? Comprehensive Guide</title>
<meta name="description" content="Explore the key concepts in algorithms with our comprehensive guide. Learn about time complexity, data structures, sorting and searching algorithms, dynamic programming, and more to enhance your programming skills.">
</head>
3. Header Tags
Use a clear hierarchy with header tags (H1, H2, H3) to structure the content, enhancing readability and SEO.
- H1: What Are the Key Concepts in Algorithms? A Comprehensive Guide
- H2: Time and Space Complexity
- H3: Big O Notation
4. Internal and External Linking
- Internal Links: Link to related articles or guides on your website, such as “How to Choose Your First Programming Language” or “Building a Developer Portfolio.”
- External Links: Reference authoritative sources like GeeksforGeeks, MIT OpenCourseWare, or Stack Overflow to add credibility.
5. Mobile Optimization
Ensure the guide is fully responsive, providing a seamless experience across all devices. Use responsive design principles and test on various screen sizes.
6. Page Speed
Optimize your website’s loading speed to improve user experience and SEO rankings. Techniques include:
- Compressing Images: Use tools like TinyPNG to reduce image sizes without losing quality.
- Minifying CSS and JavaScript: Remove unnecessary characters to decrease file sizes.
- Leveraging Browser Caching: Store frequently accessed resources locally on users’ devices.
7. Readable URLs
Use clear and descriptive URLs that include relevant keywords.
Example:
https://yourwebsite.com/key-concepts-algorithms
8. Engaging Content
Enhance user engagement by incorporating:
- Visuals: Use high-quality images, diagrams, and infographics to complement the text.
- Code Snippets: Provide clear and formatted code examples where applicable.
- Interactive Elements: Embed live code editors like CodePen or JSFiddle for hands-on practice.
9. Schema Markup
Implement structured data (Schema.org) to help search engines understand your content better, potentially enhancing search visibility.
Example:
{
"@context": "https://schema.org",
"@type": "Article",
"headline": "What Are the Key Concepts in Algorithms? A Comprehensive Guide",
"description": "A detailed guide outlining the key concepts in algorithms, including time and space complexity, data structures, sorting and searching algorithms, dynamic programming, and more to enhance your programming skills.",
"author": {
"@type": "Person",
"name": "Your Name"
},
"datePublished": "2024-04-27",
"publisher": {
"@type": "Organization",
"name": "Your Website Name",
"logo": {
"@type": "ImageObject",
"url": "https://yourwebsite.com/logo.png"
}
}
}
10. Regular Updates
Keep the content fresh and up-to-date by regularly reviewing and updating the guide with the latest best practices, tools, and trends in algorithms and computer science.
Final Thoughts
Mastering the key concepts in algorithms equips you with the tools to tackle complex problems, optimize solutions, and innovate in the field of computer science. Whether you’re preparing for technical interviews, enhancing your programming skills, or pursuing a career in software development, a solid understanding of algorithmic principles is indispensable. Embrace these concepts, apply them in your projects, and continue exploring the vast landscape of algorithms to achieve excellence in your programming endeavors.
Happy coding!