Writing secure and scalable web applications
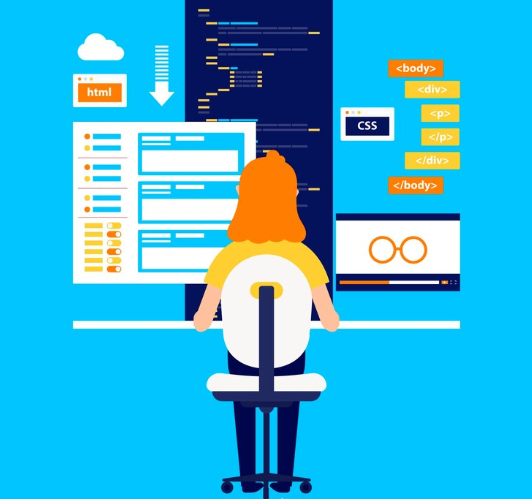
Writing Secure and Scalable Web Applications
In the rapidly evolving digital landscape, web applications serve as the backbone of countless businesses and services. As these applications handle sensitive data and cater to a growing number of users, ensuring their security and scalability becomes paramount. This comprehensive guide explores best practices, strategies, and methodologies for developing web applications that are both secure against threats and capable of handling increasing loads efficiently.
Table of Contents
- Introduction
- Security in Web Applications
- Authentication and Authorization
- Input Validation and Output Encoding
- Secure Data Storage and Transmission
- Error Handling and Logging
- Protecting Against Common Vulnerabilities
- Scalability in Web Applications
- Architectural Design for Scalability
- Load Balancing
- Caching Strategies
- Database Scalability
- Microservices vs. Monolithic Architectures
- Development Practices for Security and Scalability
- Utilizing Secure and Scalable Frameworks
- Continuous Integration and Continuous Deployment (CI/CD)
- Automated Testing
- Monitoring and Maintenance
- Performance Monitoring
- Security Monitoring
- Regular Updates and Patch Management
- Incident Response Planning
- Conclusion
- Additional Resources
Introduction
Building web applications that are both secure and scalable is a multifaceted challenge. Security ensures that the application protects sensitive data and withstands malicious attacks, while scalability guarantees that the application can handle increased traffic and data growth without compromising performance. Neglecting either aspect can lead to data breaches, service outages, and loss of user trust, which can be detrimental to any organization.
This guide aims to provide a structured approach to developing web applications that meet high standards of security and scalability. By integrating best practices from both domains, developers can create robust, reliable, and user-friendly applications.
Security in Web Applications
Ensuring the security of web applications involves implementing measures that protect against unauthorized access, data breaches, and various forms of cyberattacks. Below are key areas to focus on:
Authentication and Authorization
Authentication verifies the identity of users, while authorization determines their access levels.
- Implement Strong Authentication Mechanisms:
- Use multi-factor authentication (MFA) to add layers of security.
- Enforce strong password policies requiring complexity and regular updates.
- Role-Based Access Control (RBAC):
- Define roles and permissions clearly to restrict access based on user roles.
- Avoid using default administrative privileges for regular users.
- Token-Based Authentication:
- Utilize secure tokens (e.g., JWT) for maintaining user sessions.
- Ensure tokens are securely stored and transmitted.
Input Validation and Output Encoding
Proper handling of user inputs and outputs is crucial to prevent injection attacks and data corruption.
- Input Validation:
- Validate all incoming data against defined schemas.
- Reject or sanitize inputs that do not meet validation criteria.
- Output Encoding:
- Encode data before rendering it in the browser to prevent Cross-Site Scripting (XSS) attacks.
- Use context-specific encoding methods (e.g., HTML, JavaScript, URL encoding).
Secure Data Storage and Transmission
Protecting data both at rest and in transit safeguards against data breaches and interception.
- Encryption:
- Encrypt sensitive data in databases using strong encryption algorithms.
- Use HTTPS with TLS to secure data transmission between clients and servers.
- Secure Key Management:
- Store encryption keys securely, separate from the encrypted data.
- Rotate keys regularly and manage access controls effectively.
- Data Minimization:
- Collect only the necessary data required for application functionality.
- Implement data retention policies to delete unnecessary data.
Error Handling and Logging
Effective error handling and logging practices help in identifying and mitigating security threats without exposing sensitive information.
- Graceful Error Handling:
- Display generic error messages to users to avoid revealing system details.
- Handle exceptions securely to prevent application crashes.
- Secure Logging:
- Log security-relevant events, such as failed login attempts and suspicious activities.
- Protect log files from unauthorized access and ensure they are tamper-proof.
- Monitoring and Alerting:
- Implement real-time monitoring to detect and respond to security incidents promptly.
- Set up alerts for unusual activities or potential breaches.
Protecting Against Common Vulnerabilities
Understanding and mitigating common web vulnerabilities is essential for robust security.
- OWASP Top 10:
- Familiarize yourself with the OWASP Top 10 security risks.
- Implement recommended practices to mitigate these vulnerabilities, such as preventing SQL injection, XSS, and CSRF attacks.
- Regular Security Audits:
- Conduct periodic security assessments and penetration testing.
- Use automated tools for vulnerability scanning and remediation.
Scalability in Web Applications
Scalability ensures that web applications can handle increased loads and data growth without performance degradation. Here are key strategies to achieve scalability:
Architectural Design for Scalability
Designing the architecture with scalability in mind from the outset is crucial.
- Modular Design:
- Break down the application into smaller, manageable modules or services.
- Facilitates independent scaling and maintenance of different components.
- Stateless Services:
- Design services to be stateless, allowing them to scale horizontally without dependency on session data.
- Microservices Architecture:
- Adopt a microservices approach to enable independent deployment and scaling of services.
- Enhances flexibility and fault isolation.
Load Balancing
Distributing incoming traffic across multiple servers ensures optimal resource utilization and prevents bottlenecks.
- Implement Load Balancers:
- Use hardware or software load balancers to manage traffic distribution.
- Supports high availability and fault tolerance.
- Round-Robin and Least Connections:
- Employ algorithms like round-robin or least connections to evenly distribute load.
- Health Monitoring:
- Continuously monitor server health and reroute traffic from unhealthy instances.
Caching Strategies
Caching reduces server load and improves response times by storing frequently accessed data.
- Client-Side Caching:
- Utilize browser caching for static assets like images, CSS, and JavaScript files.
- Server-Side Caching:
- Implement caching mechanisms like Redis or Memcached for dynamic data.
- Content Delivery Networks (CDNs):
- Use CDNs to cache and serve content from geographically distributed servers, reducing latency.
Database Scalability
Scaling databases is critical for applications with large volumes of data and high transaction rates.
- Horizontal Scaling (Sharding):
- Distribute data across multiple database instances or shards to balance the load.
- Vertical Scaling:
- Upgrade existing database servers with more resources (CPU, RAM) to handle increased demand.
- Replication:
- Implement master-slave or master-master replication to enhance read performance and availability.
- NoSQL Databases:
- Consider NoSQL databases like MongoDB or Cassandra for applications requiring flexible schemas and horizontal scalability.
Microservices vs. Monolithic Architectures
Choosing the right architectural style impacts both scalability and maintainability.
- Monolithic Architecture:
- Single, unified codebase where all components are interconnected.
- Easier to develop initially but can become cumbersome to scale and maintain as the application grows.
- Microservices Architecture:
- Comprises independent services that communicate through APIs.
- Enables individual scaling, easier deployment, and better fault isolation.
- Requires robust inter-service communication and management.
Development Practices for Security and Scalability
Integrating security and scalability into the development lifecycle ensures that these aspects are not treated as afterthoughts but as integral parts of the application.
Utilizing Secure and Scalable Frameworks
Choosing the right frameworks and libraries can significantly influence the security and scalability of your application.
- Framework Selection:
- Opt for frameworks that have built-in security features and support scalable architectures, such as Django, Ruby on Rails, or Node.js with Express.
- Regular Updates:
- Keep frameworks and libraries up to date to benefit from security patches and performance improvements.
- Community and Support:
- Choose frameworks with active communities and extensive documentation to facilitate troubleshooting and enhancements.
Continuous Integration and Continuous Deployment (CI/CD)
Implementing CI/CD pipelines automates the process of building, testing, and deploying applications, ensuring consistency and efficiency.
- Automated Builds and Testing:
- Integrate automated testing (unit, integration, security) into the CI pipeline to catch issues early.
- Deployment Automation:
- Use CI/CD tools like Jenkins, GitLab CI, or GitHub Actions to automate deployments, reducing manual errors.
- Rollback Mechanisms:
- Implement strategies to quickly revert to previous stable versions in case of deployment failures.
Automated Testing
Automated testing ensures that both security and scalability are continuously validated throughout the development process.
- Security Testing:
- Incorporate static application security testing (SAST) and dynamic application security testing (DAST) tools to identify vulnerabilities.
- Performance Testing:
- Use load testing tools like JMeter or LoadRunner to assess how the application performs under various loads.
- Continuous Feedback:
- Provide developers with immediate feedback on test results to facilitate prompt issue resolution.
Monitoring and Maintenance
Ongoing monitoring and maintenance are essential to sustain security and scalability post-deployment.
Performance Monitoring
Tracking the application’s performance helps in identifying bottlenecks and ensuring smooth operation.
- Application Performance Monitoring (APM) Tools:
- Utilize tools like New Relic, Datadog, or Prometheus to monitor metrics such as response times, throughput, and error rates.
- Real-Time Alerts:
- Set up alerts for performance thresholds to enable proactive issue resolution.
- Resource Utilization Tracking:
- Monitor CPU, memory, and disk usage to optimize resource allocation and plan for scaling.
Security Monitoring
Continuous security monitoring detects and responds to threats in real-time.
- Intrusion Detection Systems (IDS):
- Implement IDS to identify and alert on suspicious activities or potential breaches.
- Log Analysis:
- Analyze logs for unusual patterns or unauthorized access attempts.
- Regular Security Audits:
- Conduct periodic reviews of security measures and policies to ensure ongoing protection.
Regular Updates and Patch Management
Keeping software components up to date mitigates vulnerabilities and enhances performance.
- Patch Management Process:
- Establish a systematic approach for applying security patches and updates promptly.
- Dependency Management:
- Monitor and update third-party libraries and frameworks to address known vulnerabilities.
- Automated Updates:
- Where feasible, automate the update process to reduce the risk of human error.
Incident Response Planning
Preparing for potential security incidents ensures a swift and effective response, minimizing damage.
- Incident Response Plan:
- Develop a comprehensive plan outlining steps to take during a security breach, including communication strategies and roles.
- Regular Drills:
- Conduct simulated incidents to test and refine the response plan.
- Post-Incident Analysis:
- Analyze incidents to identify root causes and implement measures to prevent recurrence.
Conclusion
Developing secure and scalable web applications is a dynamic and ongoing process that requires careful planning, execution, and maintenance. By prioritizing security and scalability from the early stages of development and integrating best practices throughout the lifecycle, developers can create robust applications that not only withstand cyber threats but also gracefully handle growth and increased demand.
Balancing security and scalability involves making informed architectural decisions, adopting the right tools and frameworks, implementing rigorous testing and monitoring, and fostering a culture of continuous improvement. As technology and threats evolve, staying informed and adaptable is essential to maintaining the integrity and performance of web applications.
Additional Resources
- OWASP Official Website: https://owasp.org/
- OWASP Top Ten Project: https://owasp.org/www-project-top-ten/
- NIST Cybersecurity Framework: https://www.nist.gov/cyberframework
- Mozilla Developer Network (MDN) Web Docs: https://developer.mozilla.org/
- Web Security Testing Guide (WSTG): https://owasp.org/www-project-web-security-testing-guide/
- High Scalability: http://highscalability.com/
- AWS Well-Architected Framework: https://aws.amazon.com/architecture/well-architected/
Building secure and scalable web applications is a continuous journey. By embracing best practices and leveraging the right resources, developers can create applications that are resilient, efficient, and trusted by users worldwide.