Functional Programming
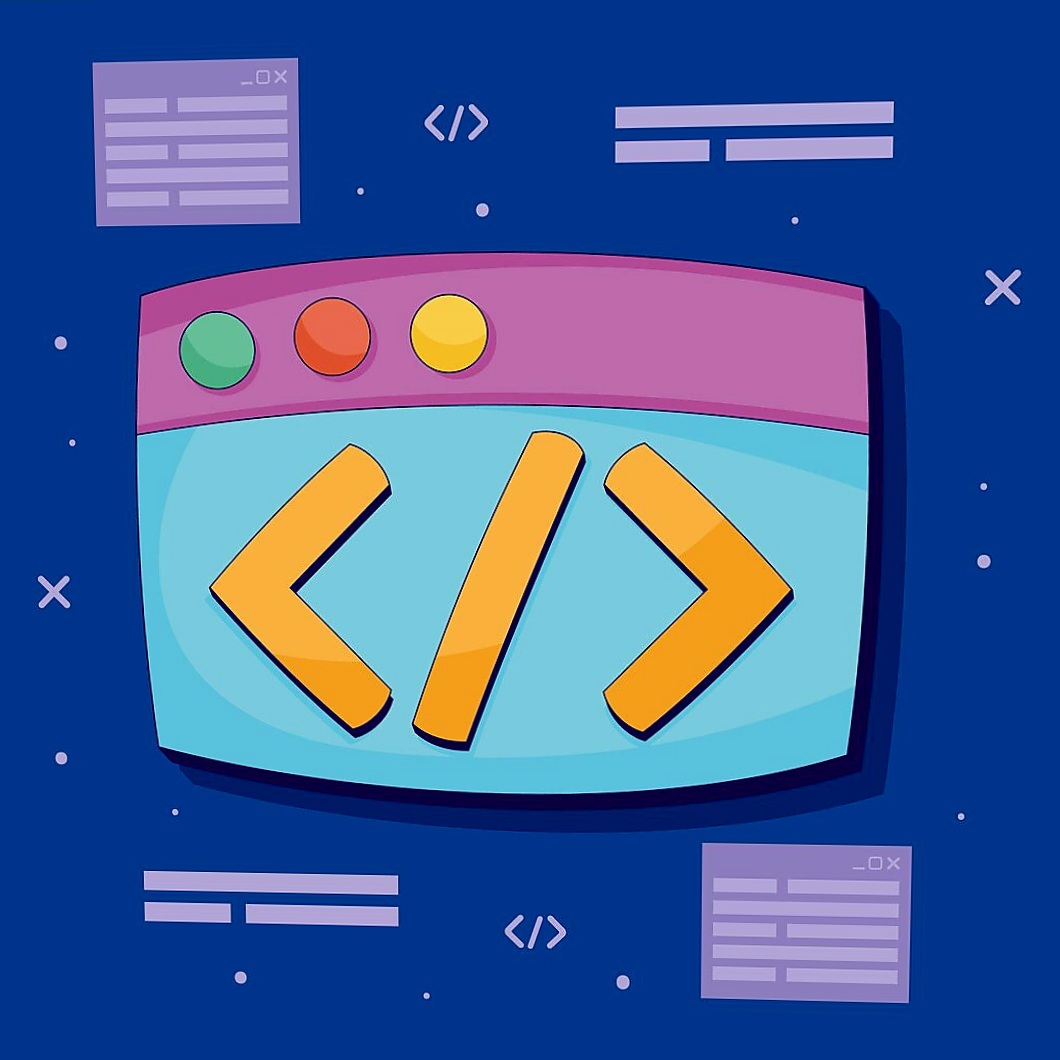
Functional programming (FP)
is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state or mutable data. Scratch, while primarily designed for beginners and teaching the basics of programming, does have elements that can be aligned with functional programming principles. Here’s an overview of functional programming concepts and how they can be related to Scratch:
Key Concepts of Functional Programming
1. First-Class Functions:
In functional programming, functions are treated as first-class citizens, meaning they can be passed as arguments to other functions, returned from functions, and assigned to variables. While Scratch doesn’t directly support first-class functions in the traditional sense, you can still create custom blocks (which are essentially named functions) that can be reused throughout your project.
Example in Scratch:
– You can define a custom block (a function) called `multiplyByTwo` and then use it in various places to reuse logic, similar to how functions in functional programming avoid duplication.
2. Pure Functions:
A pure function is a function where the output depends only on the input and has no side effects (it doesn’t modify any state or variables outside the function). In Scratch, you can mimic pure functions by ensuring that your custom blocks only return values without altering global variables or other parts of the program.
Example:
– A custom block that calculates the square of a number would always return the same result for the same input without changing anything else in the program.
3. Immutability:
In functional programming, once a data value is created, it cannot be changed (it’s immutable). While Scratch does not enforce immutability directly, you can write your code in such a way that variables are not altered unexpectedly. For example, instead of changing the value of a variable, you might create new variables that store modified values.
Example:
– Instead of modifying a variable like `score`, you could create a new variable called `newScore` when you want to make a change, thereby maintaining the original state intact.
4. Higher-Order Functions:
Higher-order functions are functions that take other functions as arguments or return functions as results. While Scratch doesn’t support passing functions as arguments in the traditional sense, you can simulate this behavior by using custom blocks with parameters that define different logic and then reusing those blocks throughout your program.
Example:
– You might have a block that calculates a result based on different conditions, where each condition is represented by a different custom block passed as an argument.
5. Recursion:
Recursion is a technique where a function calls itself. In Scratch, you can implement recursion using custom blocks. However, Scratch has limitations with deep recursion due to its stack-based execution model, so it’s best used with caution and for relatively simple tasks.
Example:
– You can create a recursive block to calculate the factorial of a number by calling the block with smaller values each time until it reaches a base case.
6. Function Composition:
In functional programming, function composition is the process of combining two or more functions to produce a new function. In Scratch, you can simulate this by combining multiple custom blocks in a sequence to achieve more complex behavior.
Example:
– You could create one block to increment a number and another block to square a number, then combine them in a script to increment a number and then square the result.
How Functional Programming Principles Apply to Scratch
While Scratch is not a purely functional language, you can still apply functional programming principles to make your code more modular, reusable, and predictable. Here are some ways you can do this:
– Use Custom Blocks: Creating custom blocks for specific tasks (e.g., calculating values, moving characters, checking conditions) helps organize your code, much like functions in functional programming.
– Avoid State Changes: Try to limit the use of global variables or states that change over time. Instead, create new variables when you need different values, and use them immutably within your blocks.
– Embrace Recursion: For tasks like counting down or finding patterns, recursion can be a clean and elegant solution. Just ensure that you manage the base case carefully to avoid infinite loops.
– Refactor Reusable Code: Similar to functional programming
, if you find yourself repeating the same logic, consider creating a custom block to handle that logic, promoting reusability.
In conclusion, while Scratch may not fully support all functional programming concepts, it’s a great platform to start thinking about them. Using custom blocks, avoiding side effects, and focusing on reusable, clean code can help you develop a more functional approach to programming even in Scratch.