Object-oriented programming with Python
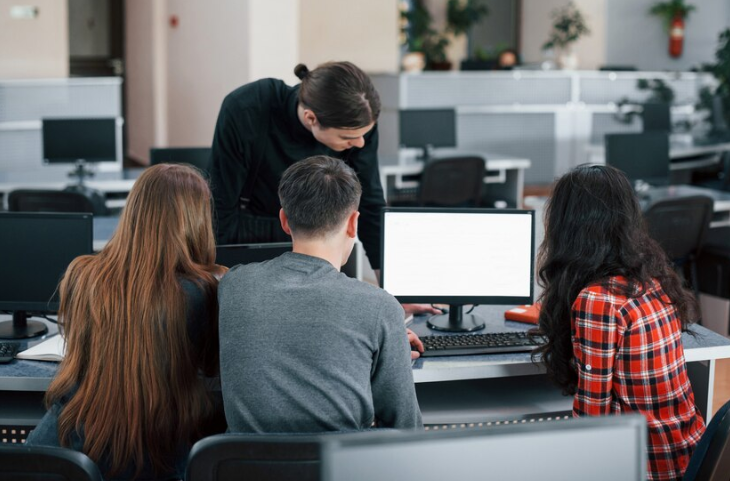
Object-Oriented Programming with Python
Object-Oriented Programming (OOP) is a fundamental programming paradigm that organizes software design around data, or objects, rather than functions and logic. Python, with its simplicity and readability, offers robust support for OOP, making it a preferred choice for developers aiming to build scalable and maintainable applications. This comprehensive guide delves into the principles of OOP in Python, providing detailed explanations, practical examples, and best practices to help you master object-oriented design and implementation.
Table of Contents
- Introduction to Object-Oriented Programming
- Classesand Objects in Python
- Defining a Class
- Creating Objects
- Attributes and Methods
- Instance Attributes
- Class Attributes
- Instance Methods
- Class Methods and Static Methods
- Inheritance
- Single Inheritance
- Multiple Inheritance
- Method Overriding
- The
super()
Function
- Encapsulation
- Public, Protected, and Private Attributes
- Getter and Setter Methods
- Polymorphism
- Duck Typing
- Method Overloading and Overriding
- Abstraction
- Abstract Base Classes
- Interfaces
- Special (Magic) Methods
- Initialization and Representation
- Operator Overloading
- Iteration Protocols
- Class vs. Instance Variables
- Composition vs. Inheritance
- Advanced OOP Concepts
- Mixins
- Multiple Inheritance and the Diamond Problem
- Design Patterns
- Best Practices in OOP with Python
- Conclusion
- Additional Resources
Introduction to Object-Oriented Programming
Object-Oriented Programming (OOP) is a paradigm that structures software around objects, which combine data and behavior. Instead of focusing solely on functions and logic, OOP emphasizes the creation of objects that interact with one another, promoting modularity, reusability, and scalability.
Key Principles of OOP:
- Encapsulation: Bundling data and methods that operate on that data within classes, restricting direct access to some of an object’s components.
- Abstraction: Simplifying complex reality by modeling classes appropriate to the problem.
- Inheritance: Allowing new classes to inherit attributes and methods from existing ones.
- Polymorphism: Enabling objects to be treated as instances of their parent class rather than their actual class.
Python’s flexible and dynamic nature makes it an excellent language for implementing OOP concepts effectively.
Classes and Objects in Python
At the heart of OOP are classes and objects. A class serves as a blueprint for creating objects, encapsulating data for the object and methods to manipulate that data.
Defining a Class
A class is defined using the class
keyword, followed by the class name and a colon. Conventionally, class names use the PascalCase
naming style.
Syntax:
class ClassName:
# Class body
pass
Example:
class Dog:
pass
Creating Objects
An object is an instance of a class. Once a class is defined, you can create multiple objects from it.
Example:
class Dog:
pass
# Creating an object of the Dog class
my_dog = Dog()
another_dog = Dog()
In this example, my_dog
and another_dog
are two distinct objects created from the Dog
class.
Attributes and Methods
Attributes and methods define the state and behavior of objects created from a class.
Instance Attributes
Instance attributes are specific to each object. They are defined within methods, typically the __init__
method.
Example:
class Dog:
def __init__(self, name, age):
self.name = name # Instance attribute
self.age = age # Instance attribute
# Creating an object with specific attributes
my_dog = Dog("Buddy", 3)
print(my_dog.name) # Output: Buddy
print(my_dog.age) # Output: 3
In this example, name
and age
are instance attributes unique to each Dog
object.
Class Attributes
Class attributes are shared across all instances of a class. They are defined directly within the class body, outside of any methods.
Example:
class Dog:
species = "Canis familiaris" # Class attribute
def __init__(self, name, age):
self.name = name
self.age = age
# All Dog instances share the 'species' attribute
dog1 = Dog("Buddy", 3)
dog2 = Dog("Charlie", 5)
print(dog1.species) # Output: Canis familiaris
print(dog2.species) # Output: Canis familiaris
Changing a class attribute affects all instances that haven’t overridden it.
Instance Methods
Instance methods are functions defined within a class that operate on instances of the class. They can access and modify instance attributes.
Example:
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
def bark(self):
print(f"{self.name} says woof!")
# Creating an object and calling its method
my_dog = Dog("Buddy", 3)
my_dog.bark() # Output: Buddy says woof!
Class Methods and Static Methods
Besides instance methods, Python supports class methods and static methods, which provide different ways to interact with class data.
Class Methods
Class methods receive the class itself as the first argument (cls
) and can modify class state that applies across all instances.
Syntax:
class ClassName:
@classmethod
def method_name(cls, parameters):
# Method body
pass
Example:
class Dog:
species = "Canis familiaris"
def __init__(self, name, age):
self.name = name
self.age = age
@classmethod
def change_species(cls, new_species):
cls.species = new_species
# Changing the class attribute using a class method
Dog.change_species("Canis lupus familiaris")
print(Dog.species) # Output: Canis lupus familiaris
Static Methods
Static methods do not receive an implicit first argument and cannot modify class or instance state. They are utility functions within a class.
Syntax:
class ClassName:
@staticmethod
def method_name(parameters):
# Method body
pass
Example:
class MathUtilities:
@staticmethod
def add(a, b):
return a + b
# Calling a static method without creating an instance
result = MathUtilities.add(5, 7)
print(result) # Output: 12
Inheritance
Inheritance allows a class (child or subclass) to inherit attributes and methods from another class (parent or superclass), promoting code reuse and hierarchical relationships.
Single Inheritance
In single inheritance, a subclass inherits from one superclass.
Example:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
pass
class Dog(Animal):
def speak(self):
return f"{self.name} says woof!"
# Creating an object of the Dog class
my_dog = Dog("Buddy")
print(my_dog.speak()) # Output: Buddy says woof!
Multiple Inheritance
A subclass can inherit from multiple superclasses, combining their attributes and methods.
Example:
class Walkable:
def walk(self):
print("Walking...")
class Swimmable:
def swim(self):
print("Swimming...")
class Duck(Walkable, Swimmable):
def quack(self):
print("Quack!")
# Creating an object of the Duck class
donald = Duck()
donald.walk() # Output: Walking...
donald.swim() # Output: Swimming...
donald.quack() # Output: Quack!
Caution: Multiple inheritance can lead to complexity, especially with the Diamond Problem, where a subclass inherits from two classes that share a common superclass. Python’s Method Resolution Order (MRO) helps manage this, but careful design is essential.
Method Overriding
Subclasses can override methods from their superclasses to provide specific implementations.
Example:
class Animal:
def speak(self):
return "Some generic sound"
class Cat(Animal):
def speak(self):
return "Meow!"
# Creating objects
generic_animal = Animal()
my_cat = Cat()
print(generic_animal.speak()) # Output: Some generic sound
print(my_cat.speak()) # Output: Meow!
The super()
Function
The super()
function allows subclasses to call methods from their superclass, enabling extended or modified behavior without completely overriding the method.
Example:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
return "Some generic sound"
class Dog(Animal):
def __init__(self, name, breed):
super().__init__(name) # Calling the superclass constructor
self.breed = breed
def speak(self):
return f"{self.name} says woof!"
# Creating an object of the Dog class
my_dog = Dog("Buddy", "Golden Retriever")
print(my_dog.name) # Output: Buddy
print(my_dog.breed) # Output: Golden Retriever
print(my_dog.speak())# Output: Buddy says woof!
Encapsulation
Encapsulation restricts access to certain components of an object, bundling data and methods that operate on that data within classes. It prevents external entities from modifying internal states directly, promoting data integrity and security.
Public, Protected, and Private Attributes
Python does not enforce strict access controls but follows naming conventions to indicate the intended level of access.
- Public Attributes: Accessible from anywhere. No leading underscores.
- Protected Attributes: Intended for internal use within the class and its subclasses. Single leading underscore (
_
). - Private Attributes: Intended for internal use only within the class. Double leading underscores (
__
), which triggers name mangling.
Example:
class Person:
def __init__(self, name, age):
self.name = name # Public attribute
self._age = age # Protected attribute
self.__ssn = "123-45-6789" # Private attribute
# Creating an object
john = Person("John Doe", 30)
# Accessing public attribute
print(john.name) # Output: John Doe
# Accessing protected attribute (by convention, not enforced)
print(john._age) # Output: 30
# Accessing private attribute (will raise AttributeError)
# print(john.__ssn) # Uncommenting this line will cause an error
# Accessing private attribute using name mangling
print(john._Person__ssn) # Output: 123-45-6789
Note: While Python’s name mangling makes it harder to access private attributes, it does not make them truly private. It’s a convention to signal intent rather than enforce access restrictions.
Getter and Setter Methods
To control access to private or protected attributes, getter and setter methods can be used. Python’s @property
decorator simplifies this process.
Example:
class Person:
def __init__(self, name, age):
self.name = name
self.__age = age # Private attribute
# Getter method
@property
def age(self):
return self.__age
# Setter method
@age.setter
def age(self, value):
if value >= 0:
self.__age = value
else:
raise ValueError("Age cannot be negative.")
# Creating an object
jane = Person("Jane Doe", 25)
# Accessing age using getter
print(jane.age) # Output: 25
# Modifying age using setter
jane.age = 26
print(jane.age) # Output: 26
# Attempting to set a negative age (will raise ValueError)
# jane.age = -5 # Uncommenting this line will cause an error
The @property
decorator allows methods to be accessed like attributes, providing a clean and intuitive interface.
Polymorphism
Polymorphism enables objects of different classes to be treated as instances of a common superclass, allowing for flexible and interchangeable code.
Duck Typing
Python follows the principle of duck typing, which emphasizes an object’s behavior rather than its actual type. If an object behaves like a certain type, it can be used as that type.
Example:
class Cat:
def speak(self):
return "Meow!"
class Dog:
def speak(self):
return "Woof!"
def make_animal_speak(animal):
print(animal.speak())
# Creating objects
cat = Cat()
dog = Dog()
# Both objects can be passed to the same function
make_animal_speak(cat) # Output: Meow!
make_animal_speak(dog) # Output: Woof!
In this example, both Cat
and Dog
have a speak
method, allowing them to be used interchangeably in the make_animal_speak
function, regardless of their actual class.
Method Overloading and Overriding
Method Overloading refers to defining multiple methods with the same name but different parameters. Python does not support traditional method overloading found in languages like Java or C++. Instead, default parameters or variable-length arguments are used to achieve similar functionality.
Example of Simulating Method Overloading:
class Math:
def add(self, a, b, c=0):
return a + b + c
math = Math()
print(math.add(2, 3)) # Output: 5
print(math.add(2, 3, 4)) # Output: 9
Method Overriding allows a subclass to provide a specific implementation of a method already defined in its superclass, as demonstrated earlier in the Inheritance section.
Abstraction
Abstraction involves simplifying complex systems by modeling classes appropriate to the problem, hiding unnecessary details, and exposing only relevant functionalities.
Abstract Base Classes
Python’s abc
module provides infrastructure for defining abstract base classes (ABCs). An ABC cannot be instantiated and typically includes one or more abstract methods that must be implemented by subclasses.
Example:
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
# Attempting to instantiate Shape will raise an error
# shape = Shape() # Uncommenting this line will cause an error
# Creating an instance of Rectangle
rect = Rectangle(5, 10)
print(rect.area()) # Output: 50
In this example, Shape
is an abstract base class with an abstract method area
. The Rectangle
class inherits from Shape
and provides an implementation for the area
method.
Interfaces
While Python does not have formal interfaces like some other languages, abstract base classes can be used to define interfaces by including only abstract methods.
Example:
from abc import ABC, abstractmethod
class Flyer(ABC):
@abstractmethod
def fly(self):
pass
class Bird(Flyer):
def fly(self):
print("Bird is flying.")
class Airplane(Flyer):
def fly(self):
print("Airplane is soaring.")
# Creating objects
bird = Bird()
airplane = Airplane()
bird.fly() # Output: Bird is flying.
airplane.fly() # Output: Airplane is soaring.
Both Bird
and Airplane
implement the Flyer
interface by providing their own fly
method.
Special (Magic) Methods
Python classes can define special methods, also known as magic methods, which enable the customization of class behavior. These methods are always surrounded by double underscores (__
).
Initialization and Representation
__init__
: Initializes a new instance of a class.__str__
: Returns a readable string representation of the object, used by theprint()
function.__repr__
: Returns an unambiguous string representation of the object, used in debugging.
Example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __str__(self):
return f"{self.name}, {self.age} years old."
def __repr__(self):
return f"Person(name='{self.name}', age={self.age})"
# Creating an object
john = Person("John Doe", 28)
# Using __str__
print(john) # Output: John Doe, 28 years old.
# Using __repr__
print(repr(john)) # Output: Person(name='John Doe', age=28)
Operator Overloading
Magic methods allow classes to define their behavior with respect to Python’s operators, enabling operator overloading.
Common Operator Overloading Methods:
- Arithmetic Operators:
__add__
,__sub__
,__mul__
, etc. - Comparison Operators:
__eq__
,__lt__
,__gt__
, etc. - Unary Operators:
__neg__
,__pos__
, etc.
Example:
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Vector(self.x + other.x, self.y + other.y)
def __str__(self):
return f"Vector({self.x}, {self.y})"
# Creating objects
v1 = Vector(2, 3)
v2 = Vector(4, 5)
# Using the + operator
v3 = v1 + v2
print(v3) # Output: Vector(6, 8)
Iteration Protocols
Magic methods like __iter__
and __next__
enable objects to be iterable.
Example:
class Countdown:
def __init__(self, start):
self.start = start
def __iter__(self):
self.current = self.start
return self
def __next__(self):
if self.current <= 0:
raise StopIteration
else:
num = self.current
self.current -= 1
return num
# Creating an iterable object
countdown = Countdown(3)
for number in countdown:
print(number)
# Output:
# 3
# 2
# 1
Class vs. Instance Variables
Understanding the difference between class variables and instance variables is crucial for managing shared and unique data within classes.
- Class Variables: Shared across all instances of a class. Defined within the class but outside any methods.
- Instance Variables: Unique to each instance. Defined within methods, typically the
__init__
method.
Example:
class Car:
# Class variable
wheels = 4
def __init__(self, make, model):
# Instance variables
self.make = make
self.model = model
# Creating objects
car1 = Car("Toyota", "Corolla")
car2 = Car("Honda", "Civic")
# Accessing class variable
print(car1.wheels) # Output: 4
print(car2.wheels) # Output: 4
# Modifying class variable
Car.wheels = 6
print(car1.wheels) # Output: 6
print(car2.wheels) # Output: 6
# Accessing instance variables
print(car1.make, car1.model) # Output: Toyota Corolla
print(car2.make, car2.model) # Output: Honda Civic
Note: Modifying a class variable affects all instances unless an instance variable with the same name overrides it.
Composition vs. Inheritance
Both composition and inheritance are ways to reuse code and create relationships between classes. However, they offer different approaches and have distinct use cases.
Inheritance
Inheritance models an “is-a” relationship, where a subclass inherits properties and behaviors from a superclass.
Example:
class Engine:
def start(self):
print("Engine started.")
class Car(Engine):
def drive(self):
print("Car is driving.")
# Creating an object
my_car = Car()
my_car.start() # Output: Engine started.
my_car.drive() # Output: Car is driving.
Composition
Composition models a “has-a” relationship, where a class contains instances of other classes as attributes.
Example:
class Engine:
def start(self):
print("Engine started.")
class Car:
def __init__(self):
self.engine = Engine() # Composition
def drive(self):
self.engine.start()
print("Car is driving.")
# Creating an object
my_car = Car()
my_car.drive()
# Output:
# Engine started.
# Car is driving.
Advantages of Composition:
- Promotes loose coupling between classes.
- Enhances flexibility by allowing dynamic changes to component parts.
- Avoids issues related to multiple inheritance.
Advanced OOP Concepts
Exploring advanced OOP concepts can further enhance your ability to design robust and flexible Python applications.
Mixins
Mixins are a form of multiple inheritance where a class is designed to provide additional functionality to other classes without being a base class itself.
Example:
class JSONSerializable:
def to_json(self):
import json
return json.dumps(self.__dict__)
class Person(JSONSerializable):
def __init__(self, name, age):
self.name = name
self.age = age
# Creating an object
john = Person("John Doe", 30)
print(john.to_json()) # Output: {"name": "John Doe", "age": 30}
In this example, JSONSerializable
is a mixin that provides JSON serialization capabilities to the Person
class.
Multiple Inheritance and the Diamond Problem
Multiple inheritance allows a subclass to inherit from multiple superclasses. However, it can lead to the Diamond Problem, where a class inherits from two classes that share a common superclass, causing ambiguity in method resolution.
Example of Diamond Problem:
class A:
def do_something(self):
print("A's method")
class B(A):
def do_something(self):
print("B's method")
class C(A):
def do_something(self):
print("C's method")
class D(B, C):
pass
# Creating an object
d = D()
d.do_something()
Output:
B's method
Python resolves this ambiguity using the Method Resolution Order (MRO), which determines the order in which base classes are searched for a method.
Viewing MRO:
print(D.__mro__)
# Output: (<class '__main__.D'>, <class '__main__.B'>, <class '__main__.C'>, <class '__main__.A'>, <class 'object'>)
In this case, Python looks for do_something
in D
, then B
, followed by C
, and finally A
.
Design Patterns
Design Patterns are reusable solutions to common software design problems. Incorporating design patterns can lead to more maintainable and scalable code.
Common OOP Design Patterns:
- Singleton: Ensures a class has only one instance and provides a global point of access to it.
- Factory: Creates objects without specifying the exact class of the object to be created.
- Observer: Allows objects to notify other objects about changes in their state.
- Decorator: Adds new functionality to objects dynamically without altering their structure.
Example: Singleton Pattern:
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super(Singleton, cls).__new__(cls)
return cls._instance
# Creating objects
s1 = Singleton()
s2 = Singleton()
print(s1 is s2) # Output: True
In this example, the Singleton
class ensures that only one instance of the class exists throughout the program.
Best Practices in OOP with Python
Adhering to best practices ensures that your OOP designs are clean, efficient, and maintainable.
- Use Meaningful Class and Method Names:
- Class names should typically be nouns (e.g.,
Car
,Employee
). - Method names should be verbs (e.g.,
drive()
,calculate_salary()
).
- Class names should typically be nouns (e.g.,
- Encapsulate Data Appropriately:
- Use private or protected attributes to restrict direct access.
- Provide getter and setter methods for controlled access.
- Favor Composition Over Inheritance:
- Use composition to create complex functionalities by combining simpler objects.
- Avoid deep inheritance hierarchies that can lead to maintenance challenges.
- Implement the SOLID Principles:
- Single Responsibility: A class should have only one responsibility.
- Open/Closed: Classes should be open for extension but closed for modification.
- Liskov Substitution: Subclasses should be substitutable for their base classes.
- Interface Segregation: Prefer many specific interfaces over a single general one.
- Dependency Inversion: Depend on abstractions, not concretions.
- Leverage Magic Methods Wisely:
- Use magic methods to implement operator overloading and custom behaviors.
- Avoid overusing magic methods, which can lead to less readable code.
- Document Your Classes and Methods:
- Use docstrings to explain the purpose and usage of classes and methods.
- Maintain clear and concise documentation for better maintainability.
- Avoid Circular Dependencies:
- Design classes to minimize interdependencies, reducing complexity and potential errors.
- Write Unit Tests for Your Classes:
- Ensure that each class and method behaves as expected.
- Facilitates easier refactoring and enhances code reliability.
Conclusion
Object-Oriented Programming is a powerful paradigm that, when effectively utilized in Python, can lead to the development of robust, scalable, and maintainable applications. By understanding and implementing the core principles of OOP—encapsulation, abstraction, inheritance, and polymorphism—you can design classes and objects that model real-world entities and their interactions seamlessly.
Python’s flexible syntax and dynamic features make it an excellent language for embracing OOP concepts. However, it’s essential to follow best practices and design patterns to ensure that your code remains clean, efficient, and adaptable to changing requirements.
As you continue to explore OOP in Python, practice by building diverse projects, refactoring existing codebases, and studying well-designed Python applications. Engaging with the Python community and leveraging resources like documentation, tutorials, and forums will further enhance your understanding and proficiency in object-oriented design.
Additional Resources
- Official Python Documentation (Classes): https://docs.python.org/3/tutorial/classes.html
- Python OOP Tutorial by Real Python: https://realpython.com/python3-object-oriented-programming/
- “Fluent Python” by Luciano Ramalho: A comprehensive book covering advanced Python features, including OOP.
- Design Patterns in Python: https://refactoring.guru/design-patterns/python
- Python Enhancement Proposals (PEP 8 – Style Guide): https://pep8.org/
- Stack Overflow Python OOP Questions: https://stackoverflow.com/questions/tagged/python-oop
- Python Object-Oriented Programming (Video Tutorial): https://www.youtube.com/watch?v=JeznW_7DlB0
- Python OOP Practice Problems: https://www.hackerrank.com/domains/python/oop
Mastering Object-Oriented Programming in Python opens doors to creating sophisticated software solutions, enabling you to build applications that are not only functional but also elegant and maintainable. Embrace the principles, practice diligently, and leverage the rich ecosystem of Python to excel in your programming endeavors.