Programming in Swift for iOS apps
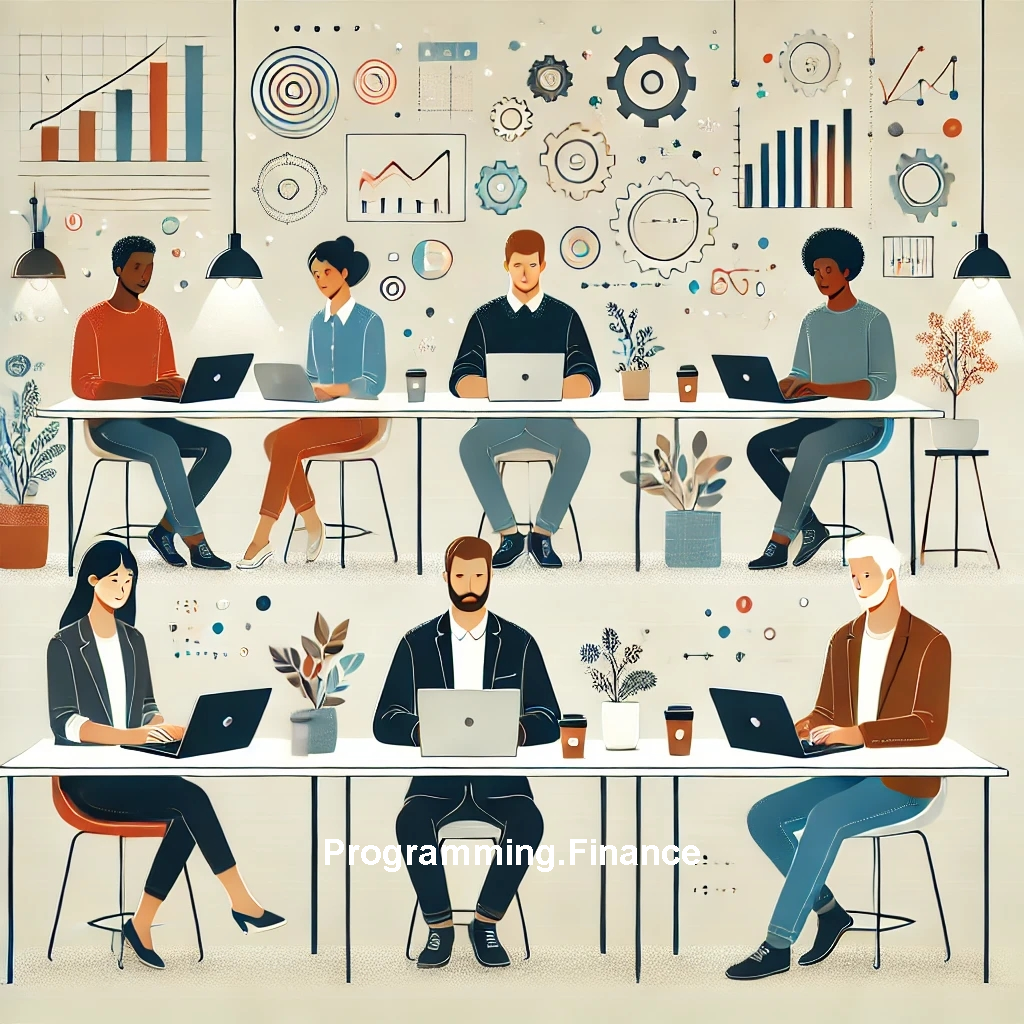
Programming in Swift for iOS Apps: A Comprehensive Guide
Swift has revolutionized iOS app development with its modern syntax, safety features, and performance optimizations. Introduced by Apple in 2014, Swift has quickly become the go-to language for building robust, efficient, and user-friendly iOS applications. Whether you’re a seasoned developer transitioning from Objective-C or a newcomer eager to dive into mobile app development, Programming in Swift for iOS Apps offers an in-depth exploration of Swift’s capabilities and best practices for creating exceptional iOS applications.
Table of Contents
- Introduction to Swift
- Setting Up the Development Environment
- Installing Xcode
- Swift Playgrounds
- Swift Basics
- Variables and Constants
- Data Types
- Operators
- Control Flow
- Advanced Swift Concepts
- Optionals
- Closures
- Protocols and Delegation
- Generics
- iOS App Development with Swift
- Understanding MVC Architecture
- SwiftUI vs. UIKit
- Building User Interfaces with SwiftUI
- Handling User Input and Events
- Working with Data
- Networking with URLSession
- Persisting Data with Core Data
- Debugging and Testing
- Using Xcode Debugger
- Writing Unit Tests
- Best Practices in Swift Programming
- Code Readability and Style
- Memory Management
- Performance Optimization
- Publishing Your iOS App
- App Store Guidelines
- App Submission Process
- Conclusion
- Additional Resources
Introduction to Swift
Swift is a powerful and intuitive programming language developed by Apple for iOS, macOS, watchOS, tvOS, and beyond. Designed to be both easy to use and highly performant, Swift combines the best of modern language features with a focus on safety and speed.
Key Features of Swift:
- Modern Syntax: Swift’s clean and expressive syntax makes it easier to read and write code, reducing the likelihood of errors.
- Safety: Features like optionals and strong type inference help prevent common programming mistakes.
- Performance: Swift is optimized for performance, often outperforming Objective-C in benchmarks.
- Interoperability: Swift seamlessly interoperates with Objective-C, allowing developers to integrate existing Objective-C codebases.
- Open Source: Swift’s open-source nature fosters a vibrant community and continuous improvements.
Hello, Swift! Example:
import UIKit
print("Hello, Swift!")
Explanation:
- The
import
statement includes the UIKit framework, essential for iOS app development. print
outputs the string “Hello, Swift!” to the console.
Swift’s design prioritizes both beginner-friendly learning curves and the needs of experienced developers, making it an ideal choice for a wide range of applications.
Setting Up the Development Environment
To start programming in Swift for iOS apps, you need to set up your development environment effectively. This involves installing the necessary tools and familiarizing yourself with the primary development platforms.
Installing Xcode
Xcode is Apple’s integrated development environment (IDE) for macOS, iOS, watchOS, and tvOS app development. It provides all the tools necessary to design, develop, test, and submit your apps to the App Store.
Steps to Install Xcode:
- System Requirements:
- Ensure your Mac is running the latest version of macOS.
- Xcode requires a substantial amount of disk space (approximately 40GB).
- Download from the Mac App Store:
- Open the App Store on your Mac.
- Search for Xcode.
- Click Get and then Install.
- Command Line Tools (Optional but Recommended):
- Open Terminal.
- Run the command:
xcode-select --install
- Follow the on-screen instructions to complete the installation.
- Launch Xcode:
- Open Xcode from the Applications folder.
- Agree to the license agreement and allow Xcode to install additional components.
Key Features of Xcode:
- Interface Builder: Drag-and-drop interface for designing user interfaces.
- Simulator: Test your apps on various iOS devices without needing physical hardware.
- Debugging Tools: Powerful debugger to inspect and fix issues in your code.
- Version Control Integration: Seamlessly integrates with Git for source code management.
Swift Playgrounds
Swift Playgrounds is an innovative app for iPad and Mac that makes learning Swift interactive and fun. It’s an excellent tool for beginners to experiment with Swift code in a playful environment.
Benefits of Using Swift Playgrounds:
- Immediate Feedback: See the results of your code in real-time.
- Interactive Learning: Solve puzzles and challenges to reinforce Swift concepts.
- Experimentation: Test snippets of Swift code without setting up a full project.
Getting Started with Swift Playgrounds:
- Download Swift Playgrounds:
- Available on the Mac App Store and iPad App Store.
- Search for Swift Playgrounds and install it.
- Explore Lessons:
- Open Swift Playgrounds and browse the available lessons.
- Start with the basics and progressively tackle more complex challenges.
- Create Your Own Playgrounds:
- Use the Blank Playground option to write and test your own Swift code.
Example: Simple Playground Code
import UIKit
var greeting = "Hello, Swift Playgrounds!"
print(greeting)
Explanation:
- The
greeting
variable stores a string. print
outputs the greeting to the console.
Swift Playgrounds bridges the gap between learning and application, making it an indispensable tool for aspiring iOS developers.
Swift Basics
Before diving into iOS app development, it’s essential to grasp the fundamental concepts of Swift. This section covers the basics, including variables, data types, operators, and control flow structures.
Variables and Constants
In Swift, variables and constants are used to store and manage data.
- Variables (
var
): Mutable storage that can be changed after initialization. - Constants (
let
): Immutable storage that cannot be altered once set.
Example:
var name = "Alice"
name = "Bob" // Valid
let birthYear = 1990
// birthYear = 1991 // Error: Cannot assign to value: 'birthYear' is a 'let' constant
Best Practices:
- Use
let
by default to promote immutability and enhance code safety. - Switch to
var
only when you need to modify the stored value.
Data Types
Swift is a strongly typed language, meaning each variable and constant must have a specific data type.
Common Data Types:
- Int: Integer values.
var age: Int = 25
- Double: 64-bit floating-point numbers.
var price: Double = 19.99
- Float: 32-bit floating-point numbers.
var temperature: Float = 23.5
- String: Textual data.
var greeting: String = "Hello, World!"
- Bool: Boolean values (
true
orfalse
).var isActive: Bool = true
Type Inference:
Swift can automatically infer the type based on the assigned value, reducing the need for explicit type declarations.
Example:
var city = "New York" // Inferred as String
var score = 95 // Inferred as Int
Operators
Operators perform operations on variables and values. Swift supports a wide range of operators, including arithmetic, assignment, comparison, and logical operators.
1. Arithmetic Operators:
+
: Addition-
: Subtraction*
: Multiplication/
: Division%
: Modulus
Example:
let a = 10
let b = 3
let sum = a + b // 13
let product = a * b // 30
let remainder = a % b // 1
2. Assignment Operators:
=
: Assigns the value on the right to the variable on the left.+=
,-=
,*=
,/=
,%=
: Perform arithmetic operation and assignment in one step.
Example:
var total = 50
total += 25 // total is now 75
3. Comparison Operators:
==
: Equal to!=
: Not equal to>
: Greater than<
: Less than>=
: Greater than or equal to<=
: Less than or equal to
Example:
let x = 5
let y = 10
print(x < y) // true
print(x == y) // false
4. Logical Operators:
&&
: Logical AND||
: Logical OR!
: Logical NOT
Example:
let isLoggedIn = true
let hasPremium = false
print(isLoggedIn && hasPremium) // false
print(isLoggedIn || hasPremium) // true
print(!isLoggedIn) // false
Control Flow
Control flow statements dictate the execution path of your code based on certain conditions or repeated actions.
Conditional Statements
1. If-Else Statement:
Executes code blocks based on whether a condition is true or false.
Example:
let temperature = 25
if temperature > 30 {
print("It's hot outside.")
} else if temperature > 20 {
print("The weather is pleasant.")
} else {
print("It's cold outside.")
}
// Output: The weather is pleasant.
2. Switch Statement:
Matches the value of a variable against multiple cases.
Example:
let day = "Monday"
switch day {
case "Monday":
print("Start of the work week.")
case "Friday":
print("End of the work week.")
default:
print("Midweek day.")
}
// Output: Start of the work week.
3. Ternary Operator:
A shorthand for simple if-else statements.
Syntax:
condition ? expressionIfTrue : expressionIfFalse
Example:
let isMember = true
let discount = isMember ? 10 : 0
print("Discount: \(discount)%") // Discount: 10%
Loops
1. For-In Loop:
Iterates over a sequence, such as arrays, ranges, or dictionaries.
Example:
let fruits = ["Apple", "Banana", "Cherry"]
for fruit in fruits {
print(fruit)
}
// Output:
// Apple
// Banana
// Cherry
2. While Loop:
Continues to execute a block of code as long as a condition remains true.
Example:
var count = 3
while count > 0 {
print("Countdown: \(count)")
count -= 1
}
// Output:
// Countdown: 3
// Countdown: 2
// Countdown: 1
3. Repeat-While Loop:
Executes a block of code at least once before checking the condition.
Example:
var number = 0
repeat {
print("Number is \(number)")
number += 1
} while number < 3
// Output:
// Number is 0
// Number is 1
// Number is 2
4. For-Each Loop:
Provides a more functional approach to iterating over collections.
Example:
let numbers = [1, 2, 3, 4, 5]
numbers.forEach { number in
print(number * number)
}
// Output:
// 1
// 4
// 9
// 16
// 25
Advanced Swift Concepts
To build sophisticated iOS applications, it’s crucial to understand advanced Swift concepts that enhance code flexibility, safety, and efficiency.
Optionals
Optionals represent variables that may hold a value or nil
, indicating the absence of a value. They are fundamental in handling scenarios where a value might not be present, such as user input or data retrieval.
Declaring Optionals:
var optionalName: String? = "Alice"
optionalName = nil
Unwrapping Optionals:
- Forced Unwrapping:
- Use the exclamation mark
!
to forcefully unwrap an optional. - Caution: If the optional is
nil
, it will cause a runtime crash.
let name: String? = "Bob" print(name!) // Output: Bob
- Use the exclamation mark
- Optional Binding:
- Safely unwraps an optional using
if let
orguard let
.
if let unwrappedName = name { print("Hello, \(unwrappedName)!") } else { print("Name is nil.") }
- Safely unwraps an optional using
- Nil-Coalescing Operator (
??
):- Provides a default value if the optional is
nil
.
let defaultName = name ?? "Guest" print("Hello, \(defaultName)!")
- Provides a default value if the optional is
- Optional Chaining:
- Safely accesses properties and methods on an optional that might be
nil
.
let upperName = name?.uppercased() print(upperName ?? "No name provided.")
- Safely accesses properties and methods on an optional that might be
Example:
struct User {
var username: String
var email: String?
}
let user1 = User(username: "john_doe", email: "[email protected]")
let user2 = User(username: "jane_doe", email: nil)
if let email = user1.email {
print("User1's email: \(email)")
} else {
print("User1 has no email.")
}
let email2 = user2.email ?? "No email provided."
print("User2's email: \(email2)")
Output:
User1's email: [email protected]
User2's email: No email provided.
Closures
Closures are self-contained blocks of functionality that can be passed around and used in your code. They are similar to lambdas or anonymous functions in other programming languages.
Basic Closure Syntax:
let greetingClosure = { (name: String) -> String in
return "Hello, \(name)!"
}
print(greetingClosure("Alice")) // Output: Hello, Alice!
Trailing Closure Syntax:
When a closure is the last parameter of a function, you can use trailing closure syntax for cleaner code.
func performOperation(a: Int, b: Int, operation: (Int, Int) -> Int) -> Int {
return operation(a, b)
}
let sum = performOperation(a: 5, b: 3) { (x, y) in
return x + y
}
print(sum) // Output: 8
Shorthand Argument Names and Implicit Returns:
Swift allows you to simplify closures further by using shorthand argument names and omitting the return
keyword when the closure consists of a single expression.
let multiply = { (x: Int, y: Int) -> Int in
x * y
}
print(multiply(4, 6)) // Output: 24
// Using shorthand argument names
let add = { $0 + $1 }
print(add(10, 15)) // Output: 25
Capturing Values:
Closures can capture and store references to variables and constants from the context in which they were defined.
func makeIncrementer(amount: Int) -> () -> Int {
var total = 0
let incrementer: () -> Int = {
total += amount
return total
}
return incrementer
}
let incrementByTwo = makeIncrementer(amount: 2)
print(incrementByTwo()) // Output: 2
print(incrementByTwo()) // Output: 4
Escaping Closures:
An escaping closure is one that is called after the function it was passed to returns. It’s commonly used in asynchronous operations.
func fetchData(completion: @escaping (String) -> Void) {
DispatchQueue.global().async {
// Simulate network delay
sleep(2)
completion("Data fetched successfully.")
}
}
fetchData { message in
print(message)
}
// Output (after 2 seconds):
// Data fetched successfully.
Protocols and Delegation
Protocols define a blueprint of methods, properties, and other requirements that suit a particular task or piece of functionality. They are fundamental to Swift’s approach to polymorphism and code reuse.
Defining a Protocol:
protocol Drivable {
var speed: Int { get set }
func accelerate()
func brake()
}
Implementing a Protocol:
struct Car: Drivable {
var speed: Int = 0
func accelerate() {
speed += 10
print("Accelerating to \(speed) km/h")
}
func brake() {
speed -= 10
print("Decelerating to \(speed) km/h")
}
}
var myCar = Car()
myCar.accelerate() // Output: Accelerating to 10 km/h
myCar.brake() // Output: Decelerating to 0 km/h
Protocol Inheritance:
Protocols can inherit from other protocols, allowing for the creation of complex protocol hierarchies.
protocol Vehicle {
var make: String { get }
var model: String { get }
}
protocol Electric: Vehicle {
var batteryCapacity: Int { get set }
func chargeBattery()
}
Delegation Pattern:
Delegation is a design pattern that allows an object to communicate back to its owner in a decoupled way. It leverages protocols to define the methods that the delegate should implement.
Example:
protocol DownloadDelegate: AnyObject {
func didStartDownload()
func didFinishDownload(data: Data)
}
class Downloader {
weak var delegate: DownloadDelegate?
func startDownload() {
delegate?.didStartDownload()
// Simulate download
let data = Data() // Placeholder for downloaded data
delegate?.didFinishDownload(data: data)
}
}
class ViewController: DownloadDelegate {
let downloader = Downloader()
init() {
downloader.delegate = self
downloader.startDownload()
}
func didStartDownload() {
print("Download started.")
}
func didFinishDownload(data: Data) {
print("Download finished. Data size: \(data.count) bytes.")
}
}
let vc = ViewController()
// Output:
// Download started.
// Download finished. Data size: 0 bytes.
Explanation:
- DownloadDelegate Protocol: Defines the methods that the delegate must implement.
- Downloader Class: Has a weak delegate property and calls delegate methods during the download process.
- ViewController Class: Implements the
DownloadDelegate
protocol and sets itself as the delegate of theDownloader
instance.
Generics
Generics allow you to write flexible, reusable functions and types that can work with any type, subject to requirements that you define.
Generic Function Example:
func swapValues<T>(_ a: inout T, _ b: inout T) {
let temporaryA = a
a = b
b = temporaryA
}
var first = 10
var second = 20
swapValues(&first, &second)
print("First: \(first), Second: \(second)") // Output: First: 20, Second: 10
var str1 = "Hello"
var str2 = "World"
swapValues(&str1, &str2)
print("str1: \(str1), str2: \(str2)") // Output: str1: World, str2: Hello
Generic Types Example:
struct Stack<Element> {
private var elements: [Element] = []
mutating func push(_ item: Element) {
elements.append(item)
}
mutating func pop() -> Element? {
return elements.popLast()
}
}
var intStack = Stack<Int>()
intStack.push(1)
intStack.push(2)
print(intStack.pop()!) // Output: 2
var stringStack = Stack<String>()
stringStack.push("Swift")
stringStack.push("iOS")
print(stringStack.pop()!) // Output: iOS
Benefits of Generics:
- Reusability: Write functions and types that work with any data type.
- Type Safety: Maintain type safety without sacrificing flexibility.
- Reduced Code Duplication: Avoid writing multiple versions of similar code for different types.
iOS App Development with Swift
Building iOS applications involves more than just writing Swift code. It encompasses understanding the architecture, user interface design, event handling, and leveraging Swift’s features to create seamless user experiences.
Understanding MVC Architecture
Model-View-Controller (MVC) is a design pattern that separates an application into three interconnected components:
- Model:
- Represents the data and business logic.
- Manages the data, logic, and rules of the application.
- View:
- Represents the user interface.
- Displays data to the user and sends user actions to the controller.
- Controller:
- Acts as an intermediary between the model and the view.
- Processes user input, interacts with the model, and updates the view.
Advantages of MVC:
- Separation of Concerns: Enhances code organization and maintainability.
- Reusability: Components can be reused across different parts of the application.
- Scalability: Facilitates the development of large and complex applications.
Example:
Consider a simple to-do app:
- Model:
Task
struct representing a to-do item. - View: User interface displaying the list of tasks.
- Controller: Handles adding, removing, and updating tasks.
// Model
struct Task {
var title: String
var isCompleted: Bool
}
// Controller
class TaskController {
var tasks: [Task] = []
func addTask(title: String) {
let newTask = Task(title: title, isCompleted: false)
tasks.append(newTask)
}
func toggleTaskCompletion(at index: Int) {
tasks[index].isCompleted.toggle()
}
}
// View (Simplified)
class TaskView {
func displayTasks(_ tasks: [Task]) {
for task in tasks {
print("\(task.title) - \(task.isCompleted ? "Completed" : "Pending")")
}
}
}
SwiftUI vs. UIKit
UIKit and SwiftUI are two primary frameworks for building user interfaces in iOS applications.
UIKit:
- Imperative Framework: Developers specify how the UI should change in response to data changes.
- Mature and Feature-Rich: Extensive documentation, community support, and third-party libraries.
- Storyboards and Interface Builder: Visual tools for designing interfaces.
- Backward Compatibility: Supports older versions of iOS.
SwiftUI:
- Declarative Framework: Developers declare what the UI should look like, and SwiftUI handles the updates.
- Modern and Swift-Centric: Designed to leverage Swift’s language features.
- Live Previews: Real-time previews of UI changes within Xcode.
- Less Boilerplate: Simplifies code for building interfaces.
- Requires iOS 13+: Limited support for older iOS versions.
Choosing Between SwiftUI and UIKit:
- New Projects: SwiftUI is ideal for new projects targeting iOS 13 and above due to its modern approach and ease of use.
- Existing Projects: UIKit remains essential for maintaining and extending existing applications, especially those supporting older iOS versions.
- Hybrid Approach: Combining SwiftUI and UIKit can leverage the strengths of both frameworks, using SwiftUI for new components and UIKit for legacy parts.
Building User Interfaces with SwiftUI
SwiftUI simplifies the process of building user interfaces by allowing developers to define the UI in a declarative manner.
Basic SwiftUI Structure:
import SwiftUI
struct ContentView: View {
var body: some View {
Text("Hello, SwiftUI!")
.padding()
}
}
@main
struct MyApp: App {
var body: some Scene {
WindowGroup {
ContentView()
}
}
}
Explanation:
- ContentView: A struct conforming to the
View
protocol, defining the UI. - body: A computed property that describes the view’s content.
- @main: Entry point of the application, launching
ContentView
in a window.
Key SwiftUI Components:
- Text:
- Displays a string of text.
Text("Welcome to SwiftUI") .font(.largeTitle) .foregroundColor(.blue)
- Image:
- Displays an image from assets or system symbols.
Image(systemName: "star.fill") .resizable() .frame(width: 50, height: 50) .foregroundColor(.yellow)
- Button:
- Triggers actions when tapped.
Button(action: { print("Button tapped!") }) { Text("Tap Me") .padding() .background(Color.blue) .foregroundColor(.white) .cornerRadius(8) }
- List:
- Displays a scrollable list of items.
let fruits = ["Apple", "Banana", "Cherry"] List(fruits, id: \.self) { fruit in Text(fruit) }
- VStack and HStack:
- Arrange views vertically and horizontally.
VStack { Text("Top") Divider() Text("Bottom") } HStack { Text("Left") Divider() Text("Right") }
State Management:
SwiftUI uses property wrappers to manage state and data flow within views.
- @State: Manages mutable state within a view.
struct CounterView: View { @State private var count = 0 var body: some View { VStack { Text("Count: \(count)") Button("Increment") { count += 1 } } } }
- @Binding: Creates a two-way connection between a view and its source of truth.
struct ToggleView: View { @Binding var isOn: Bool var body: some View { Toggle("Enable Feature", isOn: $isOn) } }
- @ObservedObject and @EnvironmentObject: Manage shared data across multiple views.
Example:
import SwiftUI
class UserSettings: ObservableObject {
@Published var username: String = "Guest"
}
struct ProfileView: View {
@ObservedObject var settings: UserSettings
var body: some View {
VStack {
Text("Username: \(settings.username)")
TextField("Enter new username", text: $settings.username)
.textFieldStyle(RoundedBorderTextFieldStyle())
.padding()
}
.padding()
}
}
@main
struct MyApp: App {
@StateObject private var settings = UserSettings()
var body: some Scene {
WindowGroup {
ProfileView(settings: settings)
}
}
}
Explanation:
- UserSettings Class: Conforms to
ObservableObject
and uses@Published
to notify views of changes. - ProfileView: Observes
UserSettings
and updates the UI whenusername
changes. - @StateObject: Initializes and owns the
UserSettings
instance in the main app.
Handling User Input and Events
Interacting with users is a core aspect of iOS apps. Swift and SwiftUI provide robust mechanisms to handle user input and respond to events seamlessly.
1. Text Input:
Using TextField
to capture user input.
struct LoginView: View {
@State private var username: String = ""
@State private var password: String = ""
var body: some View {
VStack {
TextField("Username", text: $username)
.padding()
.background(Color(.secondarySystemBackground))
.cornerRadius(5)
SecureField("Password", text: $password)
.padding()
.background(Color(.secondarySystemBackground))
.cornerRadius(5)
Button(action: {
authenticateUser(username: username, password: password)
}) {
Text("Login")
.foregroundColor(.white)
.padding()
.frame(maxWidth: .infinity)
.background(Color.blue)
.cornerRadius(5)
}
.padding(.top, 20)
}
.padding()
}
func authenticateUser(username: String, password: String) {
// Authentication logic
print("Authenticating \(username)...")
}
}
Explanation:
- TextField: Captures the username input.
- SecureField: Captures the password input securely.
- Button: Triggers the authentication process when tapped.
2. Gestures:
SwiftUI allows adding gestures like tap, swipe, and drag to views.
struct DraggableCircle: View {
@State private var offset = CGSize.zero
var body: some View {
Circle()
.fill(Color.green)
.frame(width: 100, height: 100)
.offset(offset)
.gesture(
DragGesture()
.onChanged { gesture in
offset = gesture.translation
}
.onEnded { _ in
offset = .zero
}
)
}
}
Explanation:
- DragGesture: Allows the circle to be dragged around the screen.
- onChanged: Updates the circle’s position as it’s being dragged.
- onEnded: Resets the circle’s position when the drag ends.
3. Buttons and Actions:
Handling button taps to perform actions.
struct ToggleView: View {
@State private var isOn = false
var body: some View {
VStack {
Text(isOn ? "Switch is ON" : "Switch is OFF")
.padding()
Button(action: {
isOn.toggle()
}) {
Text("Toggle Switch")
.foregroundColor(.white)
.padding()
.background(isOn ? Color.green : Color.red)
.cornerRadius(5)
}
}
}
}
Explanation:
- Button: Toggles the
isOn
state when tapped. - Dynamic Styling: Changes the button’s background color based on the state.
4. Sliders and Pickers:
Capturing more nuanced user inputs.
struct VolumeControlView: View {
@State private var volume: Double = 50
var body: some View {
VStack {
Text("Volume: \(Int(volume))%")
.padding()
Slider(value: $volume, in: 0...100)
.padding()
Picker("Quality", selection: $selectedQuality) {
Text("Low").tag("Low")
Text("Medium").tag("Medium")
Text("High").tag("High")
}
.pickerStyle(SegmentedPickerStyle())
.padding()
}
}
@State private var selectedQuality: String = "Medium"
}
Explanation:
- Slider: Allows users to adjust the volume between 0% and 100%.
- Picker: Lets users select the audio quality from predefined options.
Working with Data
Managing data effectively is crucial for any iOS application. Swift provides robust tools and frameworks for handling data storage, retrieval, and manipulation.
Networking with URLSession
Fetching data from the internet is a common requirement. URLSession is a foundational framework for making HTTP requests and handling responses.
Example: Fetching JSON Data from an API
import SwiftUI
struct Post: Codable, Identifiable {
let id: Int
let title: String
let body: String
}
struct ContentView: View {
@State private var posts: [Post] = []
var body: some View {
NavigationView {
List(posts) { post in
VStack(alignment: .leading) {
Text(post.title)
.font(.headline)
Text(post.body)
.font(.subheadline)
.foregroundColor(.secondary)
}
}
.navigationTitle("Posts")
.onAppear {
fetchPosts()
}
}
}
func fetchPosts() {
guard let url = URL(string: "https://jsonplaceholder.typicode.com/posts") else {
print("Invalid URL")
return
}
URLSession.shared.dataTask(with: url) { data, response, error in
if let data = data {
if let decodedPosts = try? JSONDecoder().decode([Post].self, from: data) {
DispatchQueue.main.async {
self.posts = decodedPosts
}
return
}
}
print("Fetch failed: \(error?.localizedDescription ?? "Unknown error")")
}.resume()
}
}
Explanation:
- Post Struct: Conforms to
Codable
andIdentifiable
to map JSON data and uniquely identify each post in the list. - fetchPosts Function: Uses
URLSession
to perform an HTTP GET request to fetch posts from a sample API. - Decoding JSON: Utilizes
JSONDecoder
to parse the received JSON data intoPost
objects. - Updating UI: Updates the
posts
state variable on the main thread to reflect the fetched data in the UI.
Persisting Data with Core Data
Core Data is Apple’s framework for managing object graphs and persistent storage. It provides an efficient way to store, retrieve, and manage data within your app.
Setting Up Core Data in Xcode:
- Create a New Project with Core Data:
- When creating a new project in Xcode, check the Use Core Data option to include Core Data setup.
- Define the Data Model:
- Open the
.xcdatamodeld
file. - Add entities and attributes representing your data.
- Open the
- Generating NSManagedObject Subclasses:
- Xcode can automatically generate Swift classes for your entities.
Example: Using Core Data to Manage Tasks
import SwiftUI
import CoreData
struct ContentView: View {
@Environment(\.managedObjectContext) private var viewContext
@FetchRequest(
entity: Task.entity(),
sortDescriptors: [NSSortDescriptor(keyPath: \Task.timestamp, ascending: true)],
animation: .default)
private var tasks: FetchedResults<Task>
@State private var newTaskTitle: String = ""
var body: some View {
NavigationView {
VStack {
HStack {
TextField("Enter new task", text: $newTaskTitle)
.textFieldStyle(RoundedBorderTextFieldStyle())
.padding()
Button(action: addTask) {
Image(systemName: "plus.circle.fill")
.font(.title)
}
.padding()
}
List {
ForEach(tasks) { task in
Text(task.title ?? "Untitled")
}
.onDelete(perform: deleteTasks)
}
}
.navigationTitle("Tasks")
.toolbar {
EditButton()
}
}
}
private func addTask() {
withAnimation {
let newTask = Task(context: viewContext)
newTask.title = newTaskTitle
newTask.timestamp = Date()
do {
try viewContext.save()
newTaskTitle = ""
} catch {
let nsError = error as NSError
fatalError("Unresolved error \(nsError), \(nsError.userInfo)")
}
}
}
private func deleteTasks(offsets: IndexSet) {
withAnimation {
offsets.map { tasks[$0] }.forEach(viewContext.delete)
do {
try viewContext.save()
} catch {
let nsError = error as NSError
fatalError("Unresolved error \(nsError), \(nsError.userInfo)")
}
}
}
}
Explanation:
- @Environment(.managedObjectContext): Accesses the Core Data context.
- @FetchRequest: Fetches
Task
entities sorted by timestamp. - Adding Tasks: Creates a new
Task
object, sets its properties, and saves the context. - Deleting Tasks: Removes selected
Task
objects from the context and saves the changes.
Advantages of Core Data:
- Performance: Optimized for handling large data sets.
- Data Relationships: Manages complex relationships between data entities.
- Undo and Redo: Supports undo and redo operations out of the box.
- Versioning and Migration: Handles data model changes seamlessly.
JSON Parsing and Codable
Swift’s Codable
protocol simplifies the process of encoding and decoding data, especially when working with JSON.
Example: Parsing JSON Data
import Foundation
struct User: Codable {
let id: Int
let name: String
let email: String
}
let jsonData = """
[
{
"id": 1,
"name": "Alice",
"email": "[email protected]"
},
{
"id": 2,
"name": "Bob",
"email": "[email protected]"
}
]
""".data(using: .utf8)!
do {
let users = try JSONDecoder().decode([User].self, from: jsonData)
for user in users {
print("\(user.name) - \(user.email)")
}
} catch {
print("Failed to decode JSON: \(error.localizedDescription)")
}
Output:
Alice - [email protected]
Bob - [email protected]
Explanation:
- User Struct: Conforms to
Codable
to facilitate JSON encoding and decoding. - JSONDecoder: Parses the JSON data into an array of
User
objects.
Handling Nested JSON:
struct Address: Codable {
let street: String
let city: String
}
struct User: Codable {
let id: Int
let name: String
let email: String
let address: Address
}
let nestedJsonData = """
{
"id": 1,
"name": "Alice",
"email": "[email protected]",
"address": {
"street": "123 Main St",
"city": "Wonderland"
}
}
""".data(using: .utf8)!
do {
let user = try JSONDecoder().decode(User.self, from: nestedJsonData)
print("\(user.name) lives at \(user.address.street), \(user.address.city).")
} catch {
print("Failed to decode JSON: \(error.localizedDescription)")
}
Output:
Alice lives at 123 Main St, Wonderland.
Explanation:
- Address Struct: Represents the nested address information.
- User Struct: Includes an
Address
property, enabling nested JSON parsing.
Testing and Debugging
Ensuring your iOS application functions correctly and efficiently is crucial. Swift and Xcode provide robust tools for testing and debugging your code.
Writing Unit Tests
Unit tests verify the functionality of individual components, such as functions or classes, ensuring they work as intended.
Example: Unit Testing a Calculator Function
- Calculator.swift:
import Foundation struct Calculator { func add(_ a: Int, _ b: Int) -> Int { return a + b } func subtract(_ a: Int, _ b: Int) -> Int { return a - b } }
- CalculatorTests.swift:
import XCTest @testable import YourAppModuleName class CalculatorTests: XCTestCase { var calculator: Calculator! override func setUp() { super.setUp() calculator = Calculator() } override func tearDown() { calculator = nil super.tearDown() } func testAdd() { let result = calculator.add(2, 3) XCTAssertEqual(result, 5, "Expected 2 + 3 to equal 5") } func testSubtract() { let result = calculator.subtract(5, 3) XCTAssertEqual(result, 2, "Expected 5 - 3 to equal 2") } }
Explanation:
- Calculator Struct: Contains basic arithmetic functions.
- CalculatorTests Class: Inherits from
XCTestCase
and includes setup and teardown methods. - testAdd and testSubtract: Verify the correctness of the
add
andsubtract
functions usingXCTAssertEqual
.
Running Tests:
- Open your project in Xcode.
- Select Product > Test or press
Cmd+U
. - Xcode runs the tests and displays the results in the Test Navigator.
UI Testing
UI tests ensure that the user interface behaves as expected, interacting with UI elements programmatically.
Example: UI Testing a Login Screen
- LoginScreenUITests.swift:
import XCTest class LoginScreenUITests: XCTestCase { override func setUpWithError() throws { continueAfterFailure = false XCUIApplication().launch() } func testSuccessfulLogin() throws { let app = XCUIApplication() let usernameTextField = app.textFields["Username"] let passwordSecureField = app.secureTextFields["Password"] let loginButton = app.buttons["Login"] usernameTextField.tap() usernameTextField.typeText("testuser") passwordSecureField.tap() passwordSecureField.typeText("password123") loginButton.tap() let welcomeLabel = app.staticTexts["Welcome, testuser!"] XCTAssertTrue(welcomeLabel.exists, "The welcome message should appear after successful login.") } }
Explanation:
- testSuccessfulLogin: Simulates user interaction by entering a username and password, tapping the login button, and verifying the presence of a welcome message.
Running UI Tests:
- Select Product > Test or press
Cmd+U
in Xcode. - Xcode executes the UI tests and reports the results.
Debugging with Xcode
Xcode’s debugging tools help identify and fix issues in your code effectively.
Key Debugging Features:
- Breakpoints:
- Pause the execution of your app at specific lines of code.
- Inspect variables and the call stack when execution halts.
Setting a Breakpoint:
- Click the gutter next to the line number in the code editor to set a breakpoint.
- LLDB Debugger:
- An interactive debugger that allows you to inspect and manipulate the app’s state.
- Use commands like
po
(print object) to evaluate expressions.
Example:
po someVariable
- View Debugger:
- Visualize the view hierarchy of your app.
- Inspect UI elements, their properties, and relationships.
- Memory Graph Debugger:
- Identify memory leaks and strong reference cycles.
- Visualize object allocations and dependencies.
- Console:
- View output from
print
statements and log messages. - Execute commands using LLDB.
- View output from
Example: Inspecting a Variable in the Debugger
var message = "Hello, Debugger!"
print(message)
- Set a breakpoint on the
print
line. - Run the app in Debug mode.
- When execution pauses, use the console to inspect
message
:(lldb) po message # Output: "Hello, Debugger!"
Best Practices for Debugging:
- Use Conditional Breakpoints: Set breakpoints that trigger only when certain conditions are met.
- Step Through Code: Use step-over, step-into, and step-out features to navigate through code execution.
- Inspect Variables Regularly: Check the values of variables at different execution points to identify anomalies.
- Leverage Xcode’s Instruments: Use performance analysis and profiling tools to optimize your app.
Best Practices in Swift Programming
Adhering to best practices ensures that your Swift code is clean, efficient, and maintainable. This section outlines key guidelines to elevate your Swift programming skills.
Code Readability and Style
Writing readable and consistent code makes it easier to understand, maintain, and collaborate with others.
1. Follow Swift’s API Design Guidelines:
- Clarity: Code should clearly express its intent.
- Consistency: Use consistent naming conventions and code structure.
- Simplicity: Avoid unnecessary complexity.
2. Naming Conventions:
- Variables and Constants: Use camelCase.
var userName = "Alice" let maximumCount = 100
- Functions and Methods: Start with a lowercase letter and describe the action.
func calculateTotal() { }
- Types (Classes, Structs, Enums): Use PascalCase.
class UserProfile { } struct Address { } enum TaskStatus { }
3. Indentation and Spacing:
- Use four spaces for indentation.
- Add spaces around operators for better readability.
let total = price * quantity
4. Commenting:
- Use comments to explain complex logic or decisions.
- Avoid redundant comments that state the obvious.
// Calculate the total price including tax let totalPrice = price + (price * taxRate)
5. Documentation Comments:
- Use
///
for documenting public APIs, functions, and classes./// Calculates the sum of two integers. /// /// - Parameters: /// - a: The first integer. /// - b: The second integer. /// - Returns: The sum of `a` and `b`. func add(a: Int, b: Int) -> Int { return a + b }
Memory Management
Efficient memory management is crucial to prevent leaks and ensure optimal app performance.
1. Automatic Reference Counting (ARC):
Swift uses ARC to track and manage memory usage of class instances. Understanding ARC helps in writing memory-efficient code.
Strong References:
- Default type of references.
- Can lead to strong reference cycles if two objects hold strong references to each other.
Weak and Unowned References:
- Weak References (
weak
):- Do not increase the reference count.
- Must be optional types.
- Used to prevent strong reference cycles, especially in delegate patterns.
class Child { weak var parent: Parent? }
- Unowned References (
unowned
):- Do not increase the reference count.
- Used when the reference is expected to always have a value during its lifetime.
- Must not be optional.
class Employee { unowned var company: Company init(company: Company) { self.company = company } }
Avoiding Strong Reference Cycles:
- Use
weak
orunowned
references where appropriate. - Break reference cycles by setting one of the references to
nil
.
Example:
class Person {
var name: String
weak var apartment: Apartment?
init(name: String) {
self.name = name
}
}
class Apartment {
var number: Int
unowned var tenant: Person
init(number: Int, tenant: Person) {
self.number = number
self.tenant = tenant
}
}
Explanation:
Person
has a weak reference toApartment
to prevent a strong reference cycle.Apartment
has an unowned reference toPerson
since a tenant is expected to exist as long as the apartment does.
Performance Optimization
Optimizing your Swift code enhances app responsiveness and reduces resource consumption.
1. Efficient Data Structures:
- Choose appropriate data structures based on usage patterns.
- Use
Array
for ordered collections andDictionary
for key-value mappings.
2. Lazy Initialization:
- Use
lazy
properties to defer initialization until the property is accessed.lazy var dataManager = DataManager()
3. Avoiding Unnecessary Computations:
- Cache results of expensive operations.
- Use computed properties judiciously.
4. Minimize Memory Footprint:
- Release resources that are no longer needed.
- Use value types (
struct
andenum
) over reference types (class
) when possible to leverage stack allocation.
5. Concurrency:
- Utilize Grand Central Dispatch (GCD) or Operation Queues to perform tasks concurrently.
- Offload heavy computations to background threads to keep the UI responsive.
DispatchQueue.global(qos: .background).async { // Perform heavy task DispatchQueue.main.async { // Update UI } }
6. Profiling with Instruments:
- Use Xcode’s Instruments tool to identify performance bottlenecks, memory leaks, and other issues.
- Regularly profile your app during development to maintain optimal performance.
Example: Using Instruments to Detect Memory Leaks
- Launch Instruments:
- In Xcode, go to Product > Profile or press
Cmd+I
.
- In Xcode, go to Product > Profile or press
- Select the Leaks Template:
- Choose the Leaks template to detect memory leaks.
- Run and Analyze:
- Run your app and perform actions that might cause leaks.
- Instruments will highlight any detected memory leaks for further investigation.
Publishing Your iOS App
After developing and thoroughly testing your iOS app, the next step is to publish it to the App Store. This process involves adhering to Apple’s guidelines, preparing your app for submission, and managing the release.
App Store Guidelines
Apple has stringent guidelines to ensure that apps meet quality, security, and content standards.
Key Areas of Focus:
- Safety:
- Protect user data.
- Ensure content is appropriate for the target audience.
- Performance:
- Apps should be stable and crash-free.
- Optimize performance to prevent slowdowns.
- Business:
- Comply with all legal requirements.
- Use proper pricing and in-app purchase models.
- Design:
- Follow Human Interface Guidelines (HIG) for intuitive and consistent UI/UX.
- Ensure accessibility for all users.
- Legal:
- Respect intellectual property rights.
- Avoid prohibited content.
Resources:
- App Store Review Guidelines: Apple’s Guidelines
- Human Interface Guidelines: Apple’s HIG
App Submission Process
Submitting your app to the App Store involves several steps to ensure compliance and readiness.
1. Enroll in the Apple Developer Program:
- Requirements:
- Apple ID.
- Legal entity status (for company accounts).
- Enrollment fee of $99 per year.
- Enrollment Steps:
- Visit Apple Developer Program.
- Sign in with your Apple ID.
- Complete the enrollment form and payment.
2. Prepare Your App for Submission:
- App Metadata:
- App Name: Unique and descriptive.
- Description: Clear explanation of app functionality.
- Keywords: Relevant keywords for discoverability.
- Support URL and Marketing URL: Contact information and promotional website.
- App Icon: High-resolution icon adhering to Apple’s specifications.
- Screenshots: Capture app’s UI across different devices and orientations.
- App Version:
- Assign a version number following semantic versioning (e.g., 1.0, 1.1).
3. Configure App in App Store Connect:
- Create a New App:
- Log in to App Store Connect.
- Navigate to My Apps and click the + button to add a new app.
- Fill in required details, including platform, bundle ID, and pricing.
- App Privacy:
- Complete the privacy questionnaire detailing data collection and usage.
4. Archive and Upload Your App:
- Archiving:
- In Xcode, select your project scheme and choose Any iOS Device.
- Go to Product > Archive to create an archive of your app.
- Uploading:
- Once archived, the Organizer window appears.
- Select the archive and click Distribute App.
- Choose App Store Connect as the distribution method and follow the prompts to upload.
5. Submit for Review:
- Complete App Information:
- Fill out additional information, such as app categories and age ratings.
- Submit:
- Once all information is complete, click Submit for Review.
- Monitor the app’s status in App Store Connect.
6. App Review and Approval:
- Review Process:
- Apple’s review team assesses your app for compliance with guidelines.
- May take a few days to a week, depending on various factors.
- Responding to Feedback:
- If your app is rejected, review the feedback, make necessary changes, and resubmit.
7. Release Your App:
- Manual or Automatic Release:
- Choose to release the app immediately after approval or schedule a release date.
- Post-Release:
- Monitor app performance, user feedback, and ratings.
- Update the app as needed to fix bugs or add features.
Additional Resources:
- App Store Connect Help: Apple’s Support
- Xcode Help: Apple’s Xcode Documentation
Conclusion
Swift has transformed the landscape of iOS app development with its modern features, safety mechanisms, and performance optimizations. Its seamless integration with Apple’s ecosystem, coupled with a vibrant community and continuous enhancements, makes Swift an indispensable tool for developers aiming to create cutting-edge iOS applications.
Throughout this guide, we’ve explored the foundational aspects of Programming in Swift for iOS Apps, from setting up your development environment and mastering Swift basics to building sophisticated user interfaces with SwiftUI, managing data effectively, and adhering to best practices for maintainable and efficient code. Additionally, we’ve delved into the critical process of publishing your app to the App Store, ensuring that your creations reach a broad audience.
Key Takeaways:
- Swift’s Advantages: Modern syntax, safety features, and high performance make Swift ideal for iOS development.
- SwiftUI vs. UIKit: Understanding both frameworks allows for flexible and effective UI design.
- Data Management: Efficient handling of data through Core Data and networking with URLSession enhances app functionality.
- Testing and Debugging: Robust testing strategies and Xcode’s debugging tools ensure app reliability and performance.
- Best Practices: Emphasizing code readability, memory management, and performance optimization leads to high-quality applications.
- App Store Submission: Adhering to Apple’s guidelines and following a structured submission process is essential for successful app release.
Embarking on iOS app development with Swift opens up a world of possibilities, enabling you to build engaging, high-performance applications that enrich users’ lives. Embrace Swift’s capabilities, stay updated with the latest developments, and continuously refine your skills to excel in the dynamic field of iOS development.
Additional Resources
To further enhance your understanding and proficiency in Programming in Swift for iOS Apps, the following resources are invaluable:
Official Documentation and Tutorials
- Swift.org: Comprehensive resource for Swift language documentation and resources. Visit Swift.org
- Apple Developer Documentation: Extensive guides and references for iOS development. Visit Apple Developer
- SwiftUI Tutorials: Official tutorials to master SwiftUI. Start Learning
- Swift Playgrounds: Interactive learning environment for Swift on Mac and iPad. Download Swift Playgrounds
Books
- “The Swift Programming Language” by Apple: The definitive guide to Swift, available for free on Apple Books. Read Online
- “SwiftUI Essentials” by Apple: Focuses on building interfaces with SwiftUI. Read Online
- “iOS Programming: The Big Nerd Ranch Guide” by Christian Keur and Aaron Hillegass: Comprehensive guide covering Swift and iOS development.
Online Courses
- Udemy’s “iOS & Swift – The Complete iOS App Development Bootcamp”: Comprehensive course covering Swift, UIKit, and SwiftUI. Enroll Now
- Coursera’s “iOS App Development with Swift”: Structured program from top universities. Start Learning
- Ray Wenderlich’s Swift Tutorials: High-quality tutorials and video courses. Explore Tutorials
Community and Support
- Swift Forums: Engage with the Swift community and discuss language features. Join Swift Forums
- Stack Overflow: Ask questions and find answers related to Swift and iOS development. Browse Questions
- Reddit’s r/swift: Active community sharing news, tutorials, and discussions. Visit r/swift
- Swift on Twitter: Follow influential Swift developers and stay updated with the latest trends.
Tools and Utilities
- Xcode: Apple’s official IDE for macOS, essential for iOS development. Download Xcode
- AppCode by JetBrains: An alternative IDE with advanced features for Swift development. Learn More
- CocoaPods: Dependency manager for integrating third-party libraries. Get CocoaPods
- Swift Package Manager: Integrated package management tool for Swift projects. Learn More
SwiftUI Resources
- Hacking with SwiftUI: Practical projects and tutorials for mastering SwiftUI. Visit Hacking with Swift
- SwiftUI Lab: In-depth articles and experimental SwiftUI components. Explore SwiftUI Lab
- Design+Code’s SwiftUI Course: Video tutorials covering SwiftUI design and development. Start Learning
Podcasts
- Swift Unwrapped: Discusses Swift language features and development practices. Listen Here
- Stacktrace: Weekly discussions on software development, including Swift and iOS topics. Listen Here
- Swift by Sundell: In-depth conversations with Swift developers. Listen Here
Conferences and Events
- WWDC (Apple Worldwide Developers Conference): Annual conference with sessions on Swift and iOS development. Visit WWDC
- SwiftConf: Community-driven conference focusing on Swift. Learn More
- iOS Dev UK: Conference covering all aspects of iOS development. Visit iOS Dev UK
- Local Meetups: Join local Swift and iOS development meetups to network and learn from peers. Find Meetups
Swift Blogs and Articles
- NSHipster: Weekly journal of obscure Swift and Cocoa topics. Read NSHipster
- Swift by Sundell: Practical articles and tutorials on Swift and iOS development. Explore Swift by Sundell
- iOS Dev Weekly: Curated newsletter with the latest iOS development news. Subscribe Here
Embarking on iOS app development with Swift empowers you to create engaging, high-performance applications that delight users and stand out in the App Store. With its robust features, supportive community, and continuous evolution, Swift remains at the forefront of modern programming languages. Utilize the knowledge and resources provided in this guide to enhance your Swift programming skills and build exceptional iOS applications that make a meaningful impact.