The Power of Functional Programming in Modern Development
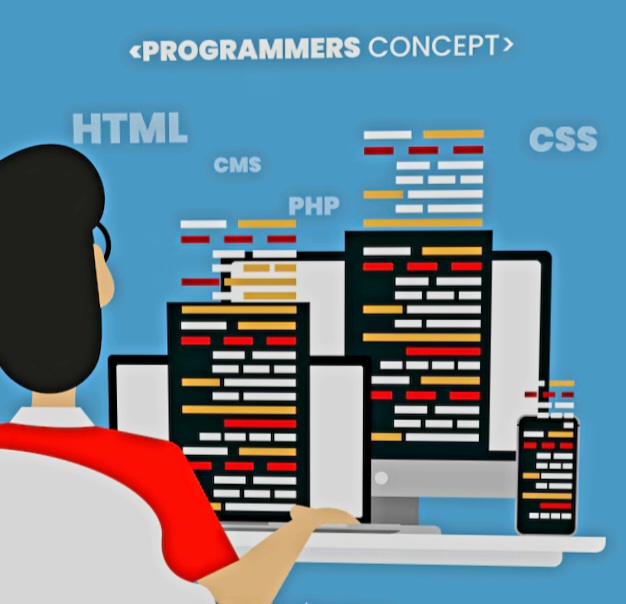
Introduction to Functional Programming in Modern Development
Functional programming (FP) is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state or mutable data. In recent years, FP has gained significant traction in modern software development due to its clean, modular, and reusable approach to code.
Key Principles of Functional Programming
1. Immutability: In functional programming, once a variable is assigned a value, it cannot be changed. This eliminates side effects and makes reasoning about programs much easier.
2. First-Class Functions: Functions in FP are first-class citizens, meaning they can be passed as arguments, returned from other functions, and assigned to variables.
3. Pure Functions: A pure function always produces the same output for the same input and has no side effects. This makes the function predictable and easier to test.
4. Higher-Order Functions: These are functions that either take one or more functions as arguments or return a function as a result. Common examples are `map()`, `filter()`, and `reduce()` in languages like JavaScript.
Why Functional Programming?
1. **Immutability & Concurrency**: In a multithreaded or concurrent environment, immutability allows for safer code execution without worrying about race conditions or data corruption.
2. Easier Debugging and Testing: Pure functions are easier to test because they don’t rely on or alter any external state.
3. Code Modularity: Functional programming encourages small, reusable functions, making codebases easier to maintain and extend.
4. Declarative Style: FP encourages writing code that expresses *what* should be done rather than *how* to do it, leading to more readable and expressive code.
Popular Languages Supporting FP
– Haskell: Known for its purely functional nature, Haskell is a great language for exploring FP concepts.
– Scala: A hybrid language that combines object-oriented and functional paradigms, making it a versatile choice for enterprise-level applications.
– JavaScript: While JavaScript is primarily an imperative language, it supports many FP features, such as higher-order functions and closures.
– Python: Python includes several functional programming constructs, like lambda functions and built-in functions like `map()` and `filter()`.
Real-World Use Cases
1. Big Data Processing: Functional programming excels in environments like big data processing frameworks (e.g., Apache Spark), where immutability and parallelism are crucial.
2. Serverless Architectures: Functional programming is a natural fit for serverless environments because it focuses on stateless, isolated computations.
3. Financial Systems: Functional programming’s predictability and immutability make it ideal for building financial systems that require high reliability and correctness.
Challenges in Adopting Functional Programming
1. Steep Learning Curve: For developers coming from an imperative programming background, FP concepts like higher-order functions and immutability can be difficult to grasp initially.
2. Performance Overhead: While FP provides many benefits, certain functional features, like immutability and recursion, can sometimes result in performance overhead compared to more imperative approaches.
Functional programming brings powerful tools to the software development toolkit. By focusing on pure functions, immutability, and higher-order functions, developers can build more reliable, scalable, and maintainable applications. While adopting FP can be challenging at first, the long-term benefits in terms of code quality and scalability make it an excellent choice for many modern applications.
Read More